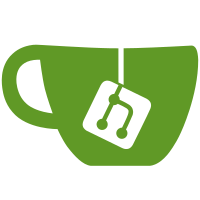
Upgraded the framework which includes the Distant cell rendering. I have been merging meshes to cut down the Draw calls, so far gaining on average 20FPS everywhere. New bugs are the magic fails (Again) and the environment presets fail to call when outside. Currently trying to build new lightmaps for the combined meshes.
194 lines
5.5 KiB
C#
194 lines
5.5 KiB
C#
using UnityEngine;
|
|
using RPGCreationKit;
|
|
using RPGCreationKit.SaveSystem;
|
|
|
|
namespace RPGCreationKit
|
|
{
|
|
[ExecuteAlways]
|
|
public class SunLightManager : MonoBehaviour
|
|
{
|
|
public static SunLightManager instance;
|
|
private bool Loaded = false;
|
|
private float TimeTrigger = 0f;
|
|
|
|
[Header("\tReferences")]
|
|
[Tooltip("The Sun.")]
|
|
[SerializeField] private Light DirectionalLight;
|
|
[Tooltip("The preset to use.")]
|
|
[SerializeField] public SunLightPreset Preset;
|
|
[Tooltip("The Stars.")]
|
|
[SerializeField] private Transform StarsSky;
|
|
[Tooltip("The player.")]
|
|
[SerializeField] private Transform Player;
|
|
[Tooltip("The settings to use in an Interior cell.")]
|
|
[SerializeField] private LightingSettings InteriorLS;
|
|
[Tooltip("The settings to use in an Exterior cell.")]
|
|
[SerializeField] private LightingSettings ExteriorLS;
|
|
|
|
[Header("\tEnable / Disable")]
|
|
[SerializeField] private bool EnableDirectionalColor = true;
|
|
[SerializeField] private bool EnableFog = true;
|
|
[SerializeField] private bool EnableSunIntensity = true;
|
|
[SerializeField] private bool EnableAmbientLight = true;
|
|
[SerializeField] private bool EnableStars = true;
|
|
|
|
[Header("\tCurrent Time")]
|
|
[Tooltip("How many frames need to pass before this script runs again. (Just to save resources)")]
|
|
[SerializeField] private int SlowDown = 2;
|
|
[SerializeField] public int Year = 0;
|
|
[SerializeField] public int Month = 1;
|
|
[SerializeField] public int Day = 1;
|
|
[SerializeField, Range(0, 24)] public float TimeOfDay;
|
|
|
|
[Header("\tTime Settings")]
|
|
[Tooltip("How many days are in a month.")]
|
|
[SerializeField] private int MonthLength = 28;
|
|
[Tooltip("How many months are in a year.")]
|
|
[SerializeField] private int YearLength = 13;
|
|
|
|
private Material StarsMat;
|
|
public bool IsInterior = false;
|
|
private int SlowDownCount = 1;
|
|
|
|
void Start() {
|
|
StarsMat = null;
|
|
StarsMat = StarsSky.GetComponent<ParticleSystem>().GetComponent<Renderer>().sharedMaterial;
|
|
|
|
Year = SaveSystemManager.instance.saveFile.Environment.Year;
|
|
Month = SaveSystemManager.instance.saveFile.Environment.Month;
|
|
Day = SaveSystemManager.instance.saveFile.Environment.Day;
|
|
TimeOfDay = SaveSystemManager.instance.saveFile.Environment.TimeOfDay;
|
|
|
|
Loaded = true;
|
|
}
|
|
|
|
// Update is called once per frame
|
|
void Update() {
|
|
if (SlowDownCount < SlowDown) { // this is just to slow down. makes this run every other frame, using only half as much power.
|
|
SlowDownCount += 1;
|
|
} else {
|
|
SlowDownCount = 0;
|
|
|
|
if (TimeTrigger > TimeOfDay) { // Should only trigger once a day.
|
|
Day += 1;
|
|
}
|
|
|
|
TimeTrigger = TimeOfDay;
|
|
|
|
if (Day > MonthLength) {
|
|
Day = 1;
|
|
Month += 1;
|
|
}
|
|
|
|
if (Month > YearLength) {
|
|
Month = 1;
|
|
Year += 1;
|
|
}
|
|
|
|
if (Loaded == false) {
|
|
return;
|
|
}
|
|
|
|
if (Preset == null) {
|
|
return;
|
|
}
|
|
|
|
if (Application.isPlaying) {
|
|
TimeOfDay += (Time.deltaTime / 180f);
|
|
TimeOfDay %= 24;
|
|
float TimePercent = TimeOfDay / 24f;
|
|
|
|
//Color WorldBrightness = Preset.WorldBrightness.Evaluate(TimePercent);
|
|
|
|
if (IsInterior == false) {
|
|
|
|
// Setting the Sun
|
|
if (DirectionalLight.gameObject.activeSelf == true) {
|
|
if (RenderSettings.sun != DirectionalLight) {
|
|
RenderSettings.sun = DirectionalLight;
|
|
|
|
}
|
|
}
|
|
|
|
// Setting the Sun
|
|
if (EnableDirectionalColor) {
|
|
Color DirectionalTempColor = Preset.DirectionalColor.Evaluate(TimePercent);
|
|
DirectionalLight.color = DirectionalTempColor;
|
|
RenderSettings.skybox.SetFloat("_Exposure", (DirectionalTempColor.a * 5));
|
|
}
|
|
|
|
// Setting the Ambiance Lighting
|
|
//InteriorLS.indirectScale = WorldBrightness.a * 1.5f;
|
|
} else {
|
|
//ExteriorLS.indirectScale = WorldBrightness.a * 1.5f;
|
|
}
|
|
UpdateLighting(TimePercent);
|
|
}
|
|
}
|
|
}
|
|
|
|
private void UpdateLighting(float TimePercent) {
|
|
if (EnableAmbientLight) {
|
|
RenderSettings.ambientLight = Preset.AmbientColor.Evaluate(TimePercent);
|
|
}
|
|
|
|
// Fog
|
|
if (EnableFog) {
|
|
Color FogTempColor = Preset.FogColor.Evaluate(TimePercent);
|
|
RenderSettings.fogColor = FogTempColor;
|
|
RenderSettings.fogDensity = ((FogTempColor.a / 10) * 2);
|
|
}
|
|
|
|
// Setting the stars
|
|
if (EnableStars) {
|
|
StarsSky.position = Player.position;
|
|
Color StarsColor = Preset.StarsVisability.Evaluate(TimePercent);
|
|
Color color = StarsMat.color;
|
|
color.a = StarsColor.a;
|
|
StarsMat.color = color;
|
|
}
|
|
|
|
// Sun Intensity (Item reflections power)
|
|
if (EnableSunIntensity) {
|
|
Color SunTempInt = Preset.SunIntensity.Evaluate(TimePercent);
|
|
DirectionalLight.intensity = ((SunTempInt.a) * 1.5f);
|
|
}
|
|
|
|
if (DirectionalLight != null) {
|
|
DirectionalLight.transform.localRotation = Quaternion.Euler(new Vector3((TimePercent * 360f) -90f, 170f, 0f));
|
|
StarsSky.localRotation = Quaternion.Euler(new Vector3((TimePercent * 360f) -90f, -100, 40));
|
|
}
|
|
}
|
|
|
|
public string GetMonth(int A) { // Ari, Tau, Gen, Can, Le, Ver, Lib, Sco, Sol, Sag, Cap, Aua, Pis.
|
|
if (A == 0) {
|
|
return "Aries";
|
|
} else if (A == 1) {
|
|
return "Taurus";
|
|
} else if (A == 2) {
|
|
return "Gemini";
|
|
} else if (A == 3) {
|
|
return "Cancer";
|
|
} else if (A == 4) {
|
|
return "Leo";
|
|
} else if (A == 5) {
|
|
return "Virgo";
|
|
} else if (A == 6) {
|
|
return "Libra";
|
|
} else if (A == 7) {
|
|
return "Scorpio";
|
|
} else if (A == 8) {
|
|
return "Sol";
|
|
} else if (A == 9) {
|
|
return "Sagittarius";
|
|
} else if (A == 10) {
|
|
return "Capricorn";
|
|
} else if (A == 11) {
|
|
return "Aquarius";
|
|
} else {
|
|
return "Pisces";
|
|
}
|
|
}
|
|
}
|
|
}
|