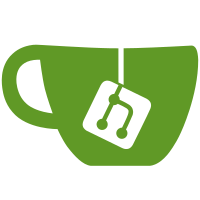
I updated everything to the latest Unity Editor. Also realized I had the wrong shaders on my hairs, those are fixed and the hairs look MUCH better!
3277 lines
135 KiB
C#
3277 lines
135 KiB
C#
//------------------------------------------------------------------------------
|
|
// <auto-generated>
|
|
// This code was auto-generated by com.unity.inputsystem:InputLayoutCodeGenerator
|
|
// version 1.5.1
|
|
// from "Keyboard" layout
|
|
//
|
|
// Changes to this file may cause incorrect behavior and will be lost if
|
|
// the code is regenerated.
|
|
// </auto-generated>
|
|
//------------------------------------------------------------------------------
|
|
|
|
using UnityEngine.InputSystem;
|
|
using UnityEngine.InputSystem.LowLevel;
|
|
using UnityEngine.InputSystem.Utilities;
|
|
|
|
// Suppress warnings from local variables for control references
|
|
// that we don't end up using.
|
|
#pragma warning disable CS0219
|
|
|
|
namespace UnityEngine.InputSystem
|
|
{
|
|
internal partial class FastKeyboard : UnityEngine.InputSystem.Keyboard
|
|
{
|
|
public const string metadata = ";AnyKey;Button;Axis;Key;DiscreteButton;Keyboard";
|
|
public FastKeyboard()
|
|
{
|
|
var builder = this.Setup(115, 15, 7)
|
|
.WithName("Keyboard")
|
|
.WithDisplayName("Keyboard")
|
|
.WithChildren(0, 115)
|
|
.WithLayout(new InternedString("Keyboard"))
|
|
.WithStateBlock(new InputStateBlock { format = new FourCC(1262836051), sizeInBits = 112 });
|
|
|
|
var kAnyKeyLayout = new InternedString("AnyKey");
|
|
var kKeyLayout = new InternedString("Key");
|
|
var kDiscreteButtonLayout = new InternedString("DiscreteButton");
|
|
var kButtonLayout = new InternedString("Button");
|
|
|
|
// /Keyboard/anyKey
|
|
var ctrlKeyboardanyKey = Initialize_ctrlKeyboardanyKey(kAnyKeyLayout, this);
|
|
|
|
// /Keyboard/escape
|
|
var ctrlKeyboardescape = Initialize_ctrlKeyboardescape(kKeyLayout, this);
|
|
|
|
// /Keyboard/space
|
|
var ctrlKeyboardspace = Initialize_ctrlKeyboardspace(kKeyLayout, this);
|
|
|
|
// /Keyboard/enter
|
|
var ctrlKeyboardenter = Initialize_ctrlKeyboardenter(kKeyLayout, this);
|
|
|
|
// /Keyboard/tab
|
|
var ctrlKeyboardtab = Initialize_ctrlKeyboardtab(kKeyLayout, this);
|
|
|
|
// /Keyboard/backquote
|
|
var ctrlKeyboardbackquote = Initialize_ctrlKeyboardbackquote(kKeyLayout, this);
|
|
|
|
// /Keyboard/quote
|
|
var ctrlKeyboardquote = Initialize_ctrlKeyboardquote(kKeyLayout, this);
|
|
|
|
// /Keyboard/semicolon
|
|
var ctrlKeyboardsemicolon = Initialize_ctrlKeyboardsemicolon(kKeyLayout, this);
|
|
|
|
// /Keyboard/comma
|
|
var ctrlKeyboardcomma = Initialize_ctrlKeyboardcomma(kKeyLayout, this);
|
|
|
|
// /Keyboard/period
|
|
var ctrlKeyboardperiod = Initialize_ctrlKeyboardperiod(kKeyLayout, this);
|
|
|
|
// /Keyboard/slash
|
|
var ctrlKeyboardslash = Initialize_ctrlKeyboardslash(kKeyLayout, this);
|
|
|
|
// /Keyboard/backslash
|
|
var ctrlKeyboardbackslash = Initialize_ctrlKeyboardbackslash(kKeyLayout, this);
|
|
|
|
// /Keyboard/leftBracket
|
|
var ctrlKeyboardleftBracket = Initialize_ctrlKeyboardleftBracket(kKeyLayout, this);
|
|
|
|
// /Keyboard/rightBracket
|
|
var ctrlKeyboardrightBracket = Initialize_ctrlKeyboardrightBracket(kKeyLayout, this);
|
|
|
|
// /Keyboard/minus
|
|
var ctrlKeyboardminus = Initialize_ctrlKeyboardminus(kKeyLayout, this);
|
|
|
|
// /Keyboard/equals
|
|
var ctrlKeyboardequals = Initialize_ctrlKeyboardequals(kKeyLayout, this);
|
|
|
|
// /Keyboard/upArrow
|
|
var ctrlKeyboardupArrow = Initialize_ctrlKeyboardupArrow(kKeyLayout, this);
|
|
|
|
// /Keyboard/downArrow
|
|
var ctrlKeyboarddownArrow = Initialize_ctrlKeyboarddownArrow(kKeyLayout, this);
|
|
|
|
// /Keyboard/leftArrow
|
|
var ctrlKeyboardleftArrow = Initialize_ctrlKeyboardleftArrow(kKeyLayout, this);
|
|
|
|
// /Keyboard/rightArrow
|
|
var ctrlKeyboardrightArrow = Initialize_ctrlKeyboardrightArrow(kKeyLayout, this);
|
|
|
|
// /Keyboard/a
|
|
var ctrlKeyboarda = Initialize_ctrlKeyboarda(kKeyLayout, this);
|
|
|
|
// /Keyboard/b
|
|
var ctrlKeyboardb = Initialize_ctrlKeyboardb(kKeyLayout, this);
|
|
|
|
// /Keyboard/c
|
|
var ctrlKeyboardc = Initialize_ctrlKeyboardc(kKeyLayout, this);
|
|
|
|
// /Keyboard/d
|
|
var ctrlKeyboardd = Initialize_ctrlKeyboardd(kKeyLayout, this);
|
|
|
|
// /Keyboard/e
|
|
var ctrlKeyboarde = Initialize_ctrlKeyboarde(kKeyLayout, this);
|
|
|
|
// /Keyboard/f
|
|
var ctrlKeyboardf = Initialize_ctrlKeyboardf(kKeyLayout, this);
|
|
|
|
// /Keyboard/g
|
|
var ctrlKeyboardg = Initialize_ctrlKeyboardg(kKeyLayout, this);
|
|
|
|
// /Keyboard/h
|
|
var ctrlKeyboardh = Initialize_ctrlKeyboardh(kKeyLayout, this);
|
|
|
|
// /Keyboard/i
|
|
var ctrlKeyboardi = Initialize_ctrlKeyboardi(kKeyLayout, this);
|
|
|
|
// /Keyboard/j
|
|
var ctrlKeyboardj = Initialize_ctrlKeyboardj(kKeyLayout, this);
|
|
|
|
// /Keyboard/k
|
|
var ctrlKeyboardk = Initialize_ctrlKeyboardk(kKeyLayout, this);
|
|
|
|
// /Keyboard/l
|
|
var ctrlKeyboardl = Initialize_ctrlKeyboardl(kKeyLayout, this);
|
|
|
|
// /Keyboard/m
|
|
var ctrlKeyboardm = Initialize_ctrlKeyboardm(kKeyLayout, this);
|
|
|
|
// /Keyboard/n
|
|
var ctrlKeyboardn = Initialize_ctrlKeyboardn(kKeyLayout, this);
|
|
|
|
// /Keyboard/o
|
|
var ctrlKeyboardo = Initialize_ctrlKeyboardo(kKeyLayout, this);
|
|
|
|
// /Keyboard/p
|
|
var ctrlKeyboardp = Initialize_ctrlKeyboardp(kKeyLayout, this);
|
|
|
|
// /Keyboard/q
|
|
var ctrlKeyboardq = Initialize_ctrlKeyboardq(kKeyLayout, this);
|
|
|
|
// /Keyboard/r
|
|
var ctrlKeyboardr = Initialize_ctrlKeyboardr(kKeyLayout, this);
|
|
|
|
// /Keyboard/s
|
|
var ctrlKeyboards = Initialize_ctrlKeyboards(kKeyLayout, this);
|
|
|
|
// /Keyboard/t
|
|
var ctrlKeyboardt = Initialize_ctrlKeyboardt(kKeyLayout, this);
|
|
|
|
// /Keyboard/u
|
|
var ctrlKeyboardu = Initialize_ctrlKeyboardu(kKeyLayout, this);
|
|
|
|
// /Keyboard/v
|
|
var ctrlKeyboardv = Initialize_ctrlKeyboardv(kKeyLayout, this);
|
|
|
|
// /Keyboard/w
|
|
var ctrlKeyboardw = Initialize_ctrlKeyboardw(kKeyLayout, this);
|
|
|
|
// /Keyboard/x
|
|
var ctrlKeyboardx = Initialize_ctrlKeyboardx(kKeyLayout, this);
|
|
|
|
// /Keyboard/y
|
|
var ctrlKeyboardy = Initialize_ctrlKeyboardy(kKeyLayout, this);
|
|
|
|
// /Keyboard/z
|
|
var ctrlKeyboardz = Initialize_ctrlKeyboardz(kKeyLayout, this);
|
|
|
|
// /Keyboard/1
|
|
var ctrlKeyboard1 = Initialize_ctrlKeyboard1(kKeyLayout, this);
|
|
|
|
// /Keyboard/2
|
|
var ctrlKeyboard2 = Initialize_ctrlKeyboard2(kKeyLayout, this);
|
|
|
|
// /Keyboard/3
|
|
var ctrlKeyboard3 = Initialize_ctrlKeyboard3(kKeyLayout, this);
|
|
|
|
// /Keyboard/4
|
|
var ctrlKeyboard4 = Initialize_ctrlKeyboard4(kKeyLayout, this);
|
|
|
|
// /Keyboard/5
|
|
var ctrlKeyboard5 = Initialize_ctrlKeyboard5(kKeyLayout, this);
|
|
|
|
// /Keyboard/6
|
|
var ctrlKeyboard6 = Initialize_ctrlKeyboard6(kKeyLayout, this);
|
|
|
|
// /Keyboard/7
|
|
var ctrlKeyboard7 = Initialize_ctrlKeyboard7(kKeyLayout, this);
|
|
|
|
// /Keyboard/8
|
|
var ctrlKeyboard8 = Initialize_ctrlKeyboard8(kKeyLayout, this);
|
|
|
|
// /Keyboard/9
|
|
var ctrlKeyboard9 = Initialize_ctrlKeyboard9(kKeyLayout, this);
|
|
|
|
// /Keyboard/0
|
|
var ctrlKeyboard0 = Initialize_ctrlKeyboard0(kKeyLayout, this);
|
|
|
|
// /Keyboard/leftShift
|
|
var ctrlKeyboardleftShift = Initialize_ctrlKeyboardleftShift(kKeyLayout, this);
|
|
|
|
// /Keyboard/rightShift
|
|
var ctrlKeyboardrightShift = Initialize_ctrlKeyboardrightShift(kKeyLayout, this);
|
|
|
|
// /Keyboard/shift
|
|
var ctrlKeyboardshift = Initialize_ctrlKeyboardshift(kDiscreteButtonLayout, this);
|
|
|
|
// /Keyboard/leftAlt
|
|
var ctrlKeyboardleftAlt = Initialize_ctrlKeyboardleftAlt(kKeyLayout, this);
|
|
|
|
// /Keyboard/rightAlt
|
|
var ctrlKeyboardrightAlt = Initialize_ctrlKeyboardrightAlt(kKeyLayout, this);
|
|
|
|
// /Keyboard/alt
|
|
var ctrlKeyboardalt = Initialize_ctrlKeyboardalt(kDiscreteButtonLayout, this);
|
|
|
|
// /Keyboard/leftCtrl
|
|
var ctrlKeyboardleftCtrl = Initialize_ctrlKeyboardleftCtrl(kKeyLayout, this);
|
|
|
|
// /Keyboard/rightCtrl
|
|
var ctrlKeyboardrightCtrl = Initialize_ctrlKeyboardrightCtrl(kKeyLayout, this);
|
|
|
|
// /Keyboard/ctrl
|
|
var ctrlKeyboardctrl = Initialize_ctrlKeyboardctrl(kDiscreteButtonLayout, this);
|
|
|
|
// /Keyboard/leftMeta
|
|
var ctrlKeyboardleftMeta = Initialize_ctrlKeyboardleftMeta(kKeyLayout, this);
|
|
|
|
// /Keyboard/rightMeta
|
|
var ctrlKeyboardrightMeta = Initialize_ctrlKeyboardrightMeta(kKeyLayout, this);
|
|
|
|
// /Keyboard/contextMenu
|
|
var ctrlKeyboardcontextMenu = Initialize_ctrlKeyboardcontextMenu(kKeyLayout, this);
|
|
|
|
// /Keyboard/backspace
|
|
var ctrlKeyboardbackspace = Initialize_ctrlKeyboardbackspace(kKeyLayout, this);
|
|
|
|
// /Keyboard/pageDown
|
|
var ctrlKeyboardpageDown = Initialize_ctrlKeyboardpageDown(kKeyLayout, this);
|
|
|
|
// /Keyboard/pageUp
|
|
var ctrlKeyboardpageUp = Initialize_ctrlKeyboardpageUp(kKeyLayout, this);
|
|
|
|
// /Keyboard/home
|
|
var ctrlKeyboardhome = Initialize_ctrlKeyboardhome(kKeyLayout, this);
|
|
|
|
// /Keyboard/end
|
|
var ctrlKeyboardend = Initialize_ctrlKeyboardend(kKeyLayout, this);
|
|
|
|
// /Keyboard/insert
|
|
var ctrlKeyboardinsert = Initialize_ctrlKeyboardinsert(kKeyLayout, this);
|
|
|
|
// /Keyboard/delete
|
|
var ctrlKeyboarddelete = Initialize_ctrlKeyboarddelete(kKeyLayout, this);
|
|
|
|
// /Keyboard/capsLock
|
|
var ctrlKeyboardcapsLock = Initialize_ctrlKeyboardcapsLock(kKeyLayout, this);
|
|
|
|
// /Keyboard/numLock
|
|
var ctrlKeyboardnumLock = Initialize_ctrlKeyboardnumLock(kKeyLayout, this);
|
|
|
|
// /Keyboard/printScreen
|
|
var ctrlKeyboardprintScreen = Initialize_ctrlKeyboardprintScreen(kKeyLayout, this);
|
|
|
|
// /Keyboard/scrollLock
|
|
var ctrlKeyboardscrollLock = Initialize_ctrlKeyboardscrollLock(kKeyLayout, this);
|
|
|
|
// /Keyboard/pause
|
|
var ctrlKeyboardpause = Initialize_ctrlKeyboardpause(kKeyLayout, this);
|
|
|
|
// /Keyboard/numpadEnter
|
|
var ctrlKeyboardnumpadEnter = Initialize_ctrlKeyboardnumpadEnter(kKeyLayout, this);
|
|
|
|
// /Keyboard/numpadDivide
|
|
var ctrlKeyboardnumpadDivide = Initialize_ctrlKeyboardnumpadDivide(kKeyLayout, this);
|
|
|
|
// /Keyboard/numpadMultiply
|
|
var ctrlKeyboardnumpadMultiply = Initialize_ctrlKeyboardnumpadMultiply(kKeyLayout, this);
|
|
|
|
// /Keyboard/numpadPlus
|
|
var ctrlKeyboardnumpadPlus = Initialize_ctrlKeyboardnumpadPlus(kKeyLayout, this);
|
|
|
|
// /Keyboard/numpadMinus
|
|
var ctrlKeyboardnumpadMinus = Initialize_ctrlKeyboardnumpadMinus(kKeyLayout, this);
|
|
|
|
// /Keyboard/numpadPeriod
|
|
var ctrlKeyboardnumpadPeriod = Initialize_ctrlKeyboardnumpadPeriod(kKeyLayout, this);
|
|
|
|
// /Keyboard/numpadEquals
|
|
var ctrlKeyboardnumpadEquals = Initialize_ctrlKeyboardnumpadEquals(kKeyLayout, this);
|
|
|
|
// /Keyboard/numpad1
|
|
var ctrlKeyboardnumpad1 = Initialize_ctrlKeyboardnumpad1(kKeyLayout, this);
|
|
|
|
// /Keyboard/numpad2
|
|
var ctrlKeyboardnumpad2 = Initialize_ctrlKeyboardnumpad2(kKeyLayout, this);
|
|
|
|
// /Keyboard/numpad3
|
|
var ctrlKeyboardnumpad3 = Initialize_ctrlKeyboardnumpad3(kKeyLayout, this);
|
|
|
|
// /Keyboard/numpad4
|
|
var ctrlKeyboardnumpad4 = Initialize_ctrlKeyboardnumpad4(kKeyLayout, this);
|
|
|
|
// /Keyboard/numpad5
|
|
var ctrlKeyboardnumpad5 = Initialize_ctrlKeyboardnumpad5(kKeyLayout, this);
|
|
|
|
// /Keyboard/numpad6
|
|
var ctrlKeyboardnumpad6 = Initialize_ctrlKeyboardnumpad6(kKeyLayout, this);
|
|
|
|
// /Keyboard/numpad7
|
|
var ctrlKeyboardnumpad7 = Initialize_ctrlKeyboardnumpad7(kKeyLayout, this);
|
|
|
|
// /Keyboard/numpad8
|
|
var ctrlKeyboardnumpad8 = Initialize_ctrlKeyboardnumpad8(kKeyLayout, this);
|
|
|
|
// /Keyboard/numpad9
|
|
var ctrlKeyboardnumpad9 = Initialize_ctrlKeyboardnumpad9(kKeyLayout, this);
|
|
|
|
// /Keyboard/numpad0
|
|
var ctrlKeyboardnumpad0 = Initialize_ctrlKeyboardnumpad0(kKeyLayout, this);
|
|
|
|
// /Keyboard/f1
|
|
var ctrlKeyboardf1 = Initialize_ctrlKeyboardf1(kKeyLayout, this);
|
|
|
|
// /Keyboard/f2
|
|
var ctrlKeyboardf2 = Initialize_ctrlKeyboardf2(kKeyLayout, this);
|
|
|
|
// /Keyboard/f3
|
|
var ctrlKeyboardf3 = Initialize_ctrlKeyboardf3(kKeyLayout, this);
|
|
|
|
// /Keyboard/f4
|
|
var ctrlKeyboardf4 = Initialize_ctrlKeyboardf4(kKeyLayout, this);
|
|
|
|
// /Keyboard/f5
|
|
var ctrlKeyboardf5 = Initialize_ctrlKeyboardf5(kKeyLayout, this);
|
|
|
|
// /Keyboard/f6
|
|
var ctrlKeyboardf6 = Initialize_ctrlKeyboardf6(kKeyLayout, this);
|
|
|
|
// /Keyboard/f7
|
|
var ctrlKeyboardf7 = Initialize_ctrlKeyboardf7(kKeyLayout, this);
|
|
|
|
// /Keyboard/f8
|
|
var ctrlKeyboardf8 = Initialize_ctrlKeyboardf8(kKeyLayout, this);
|
|
|
|
// /Keyboard/f9
|
|
var ctrlKeyboardf9 = Initialize_ctrlKeyboardf9(kKeyLayout, this);
|
|
|
|
// /Keyboard/f10
|
|
var ctrlKeyboardf10 = Initialize_ctrlKeyboardf10(kKeyLayout, this);
|
|
|
|
// /Keyboard/f11
|
|
var ctrlKeyboardf11 = Initialize_ctrlKeyboardf11(kKeyLayout, this);
|
|
|
|
// /Keyboard/f12
|
|
var ctrlKeyboardf12 = Initialize_ctrlKeyboardf12(kKeyLayout, this);
|
|
|
|
// /Keyboard/OEM1
|
|
var ctrlKeyboardOEM1 = Initialize_ctrlKeyboardOEM1(kKeyLayout, this);
|
|
|
|
// /Keyboard/OEM2
|
|
var ctrlKeyboardOEM2 = Initialize_ctrlKeyboardOEM2(kKeyLayout, this);
|
|
|
|
// /Keyboard/OEM3
|
|
var ctrlKeyboardOEM3 = Initialize_ctrlKeyboardOEM3(kKeyLayout, this);
|
|
|
|
// /Keyboard/OEM4
|
|
var ctrlKeyboardOEM4 = Initialize_ctrlKeyboardOEM4(kKeyLayout, this);
|
|
|
|
// /Keyboard/OEM5
|
|
var ctrlKeyboardOEM5 = Initialize_ctrlKeyboardOEM5(kKeyLayout, this);
|
|
|
|
// /Keyboard/IMESelected
|
|
var ctrlKeyboardIMESelected = Initialize_ctrlKeyboardIMESelected(kButtonLayout, this);
|
|
|
|
// Usages.
|
|
builder.WithControlUsage(0, new InternedString("Back"), ctrlKeyboardescape);
|
|
builder.WithControlUsage(1, new InternedString("Cancel"), ctrlKeyboardescape);
|
|
builder.WithControlUsage(2, new InternedString("Submit"), ctrlKeyboardenter);
|
|
builder.WithControlUsage(3, new InternedString("Modifier"), ctrlKeyboardleftShift);
|
|
builder.WithControlUsage(4, new InternedString("Modifier"), ctrlKeyboardrightShift);
|
|
builder.WithControlUsage(5, new InternedString("Modifier"), ctrlKeyboardshift);
|
|
builder.WithControlUsage(6, new InternedString("Modifier"), ctrlKeyboardleftAlt);
|
|
builder.WithControlUsage(7, new InternedString("Modifier"), ctrlKeyboardrightAlt);
|
|
builder.WithControlUsage(8, new InternedString("Modifier"), ctrlKeyboardalt);
|
|
builder.WithControlUsage(9, new InternedString("Modifier"), ctrlKeyboardleftCtrl);
|
|
builder.WithControlUsage(10, new InternedString("Modifier"), ctrlKeyboardrightCtrl);
|
|
builder.WithControlUsage(11, new InternedString("Modifier"), ctrlKeyboardctrl);
|
|
builder.WithControlUsage(12, new InternedString("Modifier"), ctrlKeyboardleftMeta);
|
|
builder.WithControlUsage(13, new InternedString("Modifier"), ctrlKeyboardrightMeta);
|
|
builder.WithControlUsage(14, new InternedString("Modifier"), ctrlKeyboardcontextMenu);
|
|
|
|
// Aliases.
|
|
builder.WithControlAlias(0, new InternedString("AltGr"));
|
|
builder.WithControlAlias(1, new InternedString("LeftWindows"));
|
|
builder.WithControlAlias(2, new InternedString("LeftApple"));
|
|
builder.WithControlAlias(3, new InternedString("LeftCommand"));
|
|
builder.WithControlAlias(4, new InternedString("RightWindows"));
|
|
builder.WithControlAlias(5, new InternedString("RightApple"));
|
|
builder.WithControlAlias(6, new InternedString("RightCommand"));
|
|
|
|
// Control getters/arrays.
|
|
this.keys = new UnityEngine.InputSystem.Controls.KeyControl[110];
|
|
this.keys[0] = ctrlKeyboardspace;
|
|
this.keys[1] = ctrlKeyboardenter;
|
|
this.keys[2] = ctrlKeyboardtab;
|
|
this.keys[3] = ctrlKeyboardbackquote;
|
|
this.keys[4] = ctrlKeyboardquote;
|
|
this.keys[5] = ctrlKeyboardsemicolon;
|
|
this.keys[6] = ctrlKeyboardcomma;
|
|
this.keys[7] = ctrlKeyboardperiod;
|
|
this.keys[8] = ctrlKeyboardslash;
|
|
this.keys[9] = ctrlKeyboardbackslash;
|
|
this.keys[10] = ctrlKeyboardleftBracket;
|
|
this.keys[11] = ctrlKeyboardrightBracket;
|
|
this.keys[12] = ctrlKeyboardminus;
|
|
this.keys[13] = ctrlKeyboardequals;
|
|
this.keys[14] = ctrlKeyboarda;
|
|
this.keys[15] = ctrlKeyboardb;
|
|
this.keys[16] = ctrlKeyboardc;
|
|
this.keys[17] = ctrlKeyboardd;
|
|
this.keys[18] = ctrlKeyboarde;
|
|
this.keys[19] = ctrlKeyboardf;
|
|
this.keys[20] = ctrlKeyboardg;
|
|
this.keys[21] = ctrlKeyboardh;
|
|
this.keys[22] = ctrlKeyboardi;
|
|
this.keys[23] = ctrlKeyboardj;
|
|
this.keys[24] = ctrlKeyboardk;
|
|
this.keys[25] = ctrlKeyboardl;
|
|
this.keys[26] = ctrlKeyboardm;
|
|
this.keys[27] = ctrlKeyboardn;
|
|
this.keys[28] = ctrlKeyboardo;
|
|
this.keys[29] = ctrlKeyboardp;
|
|
this.keys[30] = ctrlKeyboardq;
|
|
this.keys[31] = ctrlKeyboardr;
|
|
this.keys[32] = ctrlKeyboards;
|
|
this.keys[33] = ctrlKeyboardt;
|
|
this.keys[34] = ctrlKeyboardu;
|
|
this.keys[35] = ctrlKeyboardv;
|
|
this.keys[36] = ctrlKeyboardw;
|
|
this.keys[37] = ctrlKeyboardx;
|
|
this.keys[38] = ctrlKeyboardy;
|
|
this.keys[39] = ctrlKeyboardz;
|
|
this.keys[40] = ctrlKeyboard1;
|
|
this.keys[41] = ctrlKeyboard2;
|
|
this.keys[42] = ctrlKeyboard3;
|
|
this.keys[43] = ctrlKeyboard4;
|
|
this.keys[44] = ctrlKeyboard5;
|
|
this.keys[45] = ctrlKeyboard6;
|
|
this.keys[46] = ctrlKeyboard7;
|
|
this.keys[47] = ctrlKeyboard8;
|
|
this.keys[48] = ctrlKeyboard9;
|
|
this.keys[49] = ctrlKeyboard0;
|
|
this.keys[50] = ctrlKeyboardleftShift;
|
|
this.keys[51] = ctrlKeyboardrightShift;
|
|
this.keys[52] = ctrlKeyboardleftAlt;
|
|
this.keys[53] = ctrlKeyboardrightAlt;
|
|
this.keys[54] = ctrlKeyboardleftCtrl;
|
|
this.keys[55] = ctrlKeyboardrightCtrl;
|
|
this.keys[56] = ctrlKeyboardleftMeta;
|
|
this.keys[57] = ctrlKeyboardrightMeta;
|
|
this.keys[58] = ctrlKeyboardcontextMenu;
|
|
this.keys[59] = ctrlKeyboardescape;
|
|
this.keys[60] = ctrlKeyboardleftArrow;
|
|
this.keys[61] = ctrlKeyboardrightArrow;
|
|
this.keys[62] = ctrlKeyboardupArrow;
|
|
this.keys[63] = ctrlKeyboarddownArrow;
|
|
this.keys[64] = ctrlKeyboardbackspace;
|
|
this.keys[65] = ctrlKeyboardpageDown;
|
|
this.keys[66] = ctrlKeyboardpageUp;
|
|
this.keys[67] = ctrlKeyboardhome;
|
|
this.keys[68] = ctrlKeyboardend;
|
|
this.keys[69] = ctrlKeyboardinsert;
|
|
this.keys[70] = ctrlKeyboarddelete;
|
|
this.keys[71] = ctrlKeyboardcapsLock;
|
|
this.keys[72] = ctrlKeyboardnumLock;
|
|
this.keys[73] = ctrlKeyboardprintScreen;
|
|
this.keys[74] = ctrlKeyboardscrollLock;
|
|
this.keys[75] = ctrlKeyboardpause;
|
|
this.keys[76] = ctrlKeyboardnumpadEnter;
|
|
this.keys[77] = ctrlKeyboardnumpadDivide;
|
|
this.keys[78] = ctrlKeyboardnumpadMultiply;
|
|
this.keys[79] = ctrlKeyboardnumpadPlus;
|
|
this.keys[80] = ctrlKeyboardnumpadMinus;
|
|
this.keys[81] = ctrlKeyboardnumpadPeriod;
|
|
this.keys[82] = ctrlKeyboardnumpadEquals;
|
|
this.keys[83] = ctrlKeyboardnumpad0;
|
|
this.keys[84] = ctrlKeyboardnumpad1;
|
|
this.keys[85] = ctrlKeyboardnumpad2;
|
|
this.keys[86] = ctrlKeyboardnumpad3;
|
|
this.keys[87] = ctrlKeyboardnumpad4;
|
|
this.keys[88] = ctrlKeyboardnumpad5;
|
|
this.keys[89] = ctrlKeyboardnumpad6;
|
|
this.keys[90] = ctrlKeyboardnumpad7;
|
|
this.keys[91] = ctrlKeyboardnumpad8;
|
|
this.keys[92] = ctrlKeyboardnumpad9;
|
|
this.keys[93] = ctrlKeyboardf1;
|
|
this.keys[94] = ctrlKeyboardf2;
|
|
this.keys[95] = ctrlKeyboardf3;
|
|
this.keys[96] = ctrlKeyboardf4;
|
|
this.keys[97] = ctrlKeyboardf5;
|
|
this.keys[98] = ctrlKeyboardf6;
|
|
this.keys[99] = ctrlKeyboardf7;
|
|
this.keys[100] = ctrlKeyboardf8;
|
|
this.keys[101] = ctrlKeyboardf9;
|
|
this.keys[102] = ctrlKeyboardf10;
|
|
this.keys[103] = ctrlKeyboardf11;
|
|
this.keys[104] = ctrlKeyboardf12;
|
|
this.keys[105] = ctrlKeyboardOEM1;
|
|
this.keys[106] = ctrlKeyboardOEM2;
|
|
this.keys[107] = ctrlKeyboardOEM3;
|
|
this.keys[108] = ctrlKeyboardOEM4;
|
|
this.keys[109] = ctrlKeyboardOEM5;
|
|
this.anyKey = ctrlKeyboardanyKey;
|
|
this.shiftKey = ctrlKeyboardshift;
|
|
this.ctrlKey = ctrlKeyboardctrl;
|
|
this.altKey = ctrlKeyboardalt;
|
|
this.imeSelected = ctrlKeyboardIMESelected;
|
|
|
|
// State offset to control index map.
|
|
builder.WithStateOffsetToControlIndexMap(new uint[]
|
|
{
|
|
111616u, 525314u, 1049603u, 1573892u, 2098181u, 2622470u, 3146759u, 3671048u, 4195337u, 4719626u
|
|
, 5243915u, 5768204u, 6292493u, 6816782u, 7341071u, 7865364u, 8389653u, 8913942u, 9438231u, 9962520u
|
|
, 10486809u, 11011098u, 11535387u, 12059676u, 12583965u, 13108254u, 13632543u, 14156832u, 14681121u, 15205410u
|
|
, 15729699u, 16253988u, 16778277u, 17302566u, 17826855u, 18351144u, 18875433u, 19399722u, 19924011u, 20448300u
|
|
, 20972589u, 21496878u, 22021167u, 22545456u, 23069745u, 23594034u, 24118323u, 24642612u, 25166901u, 25691190u
|
|
, 26215479u, 26739768u, 26740794u, 27264057u, 27788347u, 27789373u, 28312636u, 28836926u, 28837952u, 29361215u
|
|
, 29885505u, 30409794u, 30934083u, 31458305u, 31982610u, 32506899u, 33031184u, 33555473u, 34079812u, 34604101u
|
|
, 35128390u, 35652679u, 36176968u, 36701257u, 37225546u, 37749835u, 38274124u, 38798413u, 39322702u, 39846991u
|
|
, 40371280u, 40895569u, 41419858u, 41944147u, 42468436u, 42992725u, 43517014u, 44041312u, 44565591u, 45089880u
|
|
, 45614169u, 46138458u, 46662747u, 47187036u, 47711325u, 48235614u, 48759903u, 49284193u, 49808482u, 50332771u
|
|
, 50857060u, 51381349u, 51905638u, 52429927u, 52954216u, 53478505u, 54002794u, 54527083u, 55051372u, 55575661u
|
|
, 56099950u, 56624239u, 57148528u, 57672817u, 58197106u
|
|
});
|
|
|
|
builder.WithControlTree(new byte[]
|
|
{
|
|
// Control tree nodes as bytes
|
|
111, 0, 1, 0, 0, 0, 0, 56, 0, 15, 0, 0, 0, 2, 111, 0, 3, 0, 2, 0, 2, 84, 0, 5, 0, 0, 0, 0, 111, 0
|
|
, 169, 0, 0, 0, 0, 70, 0, 7, 0, 0, 0, 0, 84, 0, 143, 0, 0, 0, 0, 63, 0, 9, 0, 0, 0, 0, 70, 0, 53, 0
|
|
, 0, 0, 0, 60, 0, 131, 0, 0, 0, 0, 63, 0, 11, 0, 0, 0, 0, 62, 0, 13, 0, 0, 0, 0, 63, 0, 255, 255, 22, 0
|
|
, 1, 61, 0, 255, 255, 4, 0, 1, 62, 0, 255, 255, 21, 0, 1, 28, 0, 17, 0, 0, 0, 0, 56, 0, 77, 0, 0, 0, 0, 14
|
|
, 0, 19, 0, 0, 0, 0, 28, 0, 45, 0, 0, 0, 0, 7, 0, 21, 0, 0, 0, 0, 14, 0, 33, 0, 0, 0, 0, 4, 0, 23
|
|
, 0, 0, 0, 0, 7, 0, 29, 0, 0, 0, 0, 2, 0, 25, 0, 0, 0, 0, 4, 0, 27, 0, 0, 0, 0, 1, 0, 255, 255, 0
|
|
, 0, 0, 2, 0, 255, 255, 5, 0, 1, 3, 0, 255, 255, 6, 0, 1, 4, 0, 255, 255, 7, 0, 1, 6, 0, 31, 0, 0, 0, 0
|
|
, 7, 0, 255, 255, 10, 0, 1, 5, 0, 255, 255, 8, 0, 1, 6, 0, 255, 255, 9, 0, 1, 11, 0, 35, 0, 0, 0, 0, 14, 0
|
|
, 41, 0, 0, 0, 0, 9, 0, 37, 0, 0, 0, 0, 11, 0, 39, 0, 0, 0, 0, 8, 0, 255, 255, 11, 0, 1, 9, 0, 255, 255
|
|
, 12, 0, 1, 10, 0, 255, 255, 13, 0, 1, 11, 0, 255, 255, 14, 0, 1, 13, 0, 43, 0, 0, 0, 0, 14, 0, 255, 255, 17, 0
|
|
, 1, 12, 0, 255, 255, 15, 0, 1, 13, 0, 255, 255, 16, 0, 1, 21, 0, 47, 0, 0, 0, 0, 28, 0, 65, 0, 0, 0, 0, 18
|
|
, 0, 49, 0, 0, 0, 0, 21, 0, 61, 0, 0, 0, 0, 16, 0, 51, 0, 0, 0, 0, 18, 0, 59, 0, 0, 0, 0, 15, 0, 255
|
|
, 255, 18, 0, 1, 16, 0, 255, 255, 23, 0, 1, 67, 0, 55, 0, 0, 0, 0, 70, 0, 139, 0, 0, 0, 0, 65, 0, 57, 0, 0
|
|
, 0, 0, 67, 0, 137, 0, 0, 0, 0, 64, 0, 255, 255, 19, 0, 1, 65, 0, 255, 255, 20, 0, 1, 17, 0, 255, 255, 24, 0, 1
|
|
, 18, 0, 255, 255, 25, 0, 1, 20, 0, 63, 0, 0, 0, 0, 21, 0, 255, 255, 28, 0, 1, 19, 0, 255, 255, 26, 0, 1, 20, 0
|
|
, 255, 255, 27, 0, 1, 25, 0, 67, 0, 0, 0, 0, 28, 0, 73, 0, 0, 0, 0, 23, 0, 69, 0, 0, 0, 0, 25, 0, 71, 0
|
|
, 0, 0, 0, 22, 0, 255, 255, 29, 0, 1, 23, 0, 255, 255, 30, 0, 1, 24, 0, 255, 255, 31, 0, 1, 25, 0, 255, 255, 32, 0
|
|
, 1, 27, 0, 75, 0, 0, 0, 0, 28, 0, 255, 255, 35, 0, 1, 26, 0, 255, 255, 33, 0, 1, 27, 0, 255, 255, 34, 0, 1, 42
|
|
, 0, 79, 0, 0, 0, 0, 56, 0, 105, 0, 0, 0, 0, 35, 0, 81, 0, 0, 0, 0, 42, 0, 93, 0, 0, 0, 0, 32, 0, 83
|
|
, 0, 0, 0, 0, 35, 0, 89, 0, 0, 0, 0, 30, 0, 85, 0, 0, 0, 0, 32, 0, 87, 0, 0, 0, 0, 29, 0, 255, 255, 36
|
|
, 0, 1, 30, 0, 255, 255, 37, 0, 1, 31, 0, 255, 255, 38, 0, 1, 32, 0, 255, 255, 39, 0, 1, 34, 0, 91, 0, 0, 0, 0
|
|
, 35, 0, 255, 255, 42, 0, 1, 33, 0, 255, 255, 40, 0, 1, 34, 0, 255, 255, 41, 0, 1, 39, 0, 95, 0, 0, 0, 0, 42, 0
|
|
, 101, 0, 0, 0, 0, 37, 0, 97, 0, 0, 0, 0, 39, 0, 99, 0, 0, 0, 0, 36, 0, 255, 255, 43, 0, 1, 37, 0, 255, 255
|
|
, 44, 0, 1, 38, 0, 255, 255, 45, 0, 1, 39, 0, 255, 255, 46, 0, 1, 41, 0, 103, 0, 0, 0, 0, 42, 0, 255, 255, 49, 0
|
|
, 1, 40, 0, 255, 255, 47, 0, 1, 41, 0, 255, 255, 48, 0, 1, 49, 0, 107, 0, 0, 0, 0, 56, 0, 119, 0, 0, 0, 0, 46
|
|
, 0, 109, 0, 0, 0, 0, 49, 0, 115, 0, 0, 0, 0, 44, 0, 111, 0, 0, 0, 0, 46, 0, 113, 0, 0, 0, 0, 43, 0, 255
|
|
, 255, 50, 0, 1, 44, 0, 255, 255, 51, 0, 1, 45, 0, 255, 255, 52, 0, 1, 46, 0, 255, 255, 53, 0, 1, 48, 0, 117, 0, 0
|
|
, 0, 0, 49, 0, 255, 255, 56, 0, 1, 47, 0, 255, 255, 54, 0, 1, 48, 0, 255, 255, 55, 0, 1, 53, 0, 121, 0, 0, 0, 0
|
|
, 56, 0, 127, 0, 0, 0, 0, 51, 0, 123, 0, 0, 0, 0, 53, 0, 125, 0, 61, 0, 1, 50, 0, 255, 255, 57, 0, 1, 51, 0
|
|
, 255, 255, 58, 0, 1, 52, 0, 255, 255, 59, 0, 1, 53, 0, 255, 255, 60, 0, 1, 55, 0, 129, 0, 64, 0, 1, 56, 0, 255, 255
|
|
, 65, 0, 1, 54, 0, 255, 255, 62, 0, 1, 55, 0, 255, 255, 63, 0, 1, 58, 0, 133, 0, 0, 0, 0, 60, 0, 135, 0, 0, 0
|
|
, 0, 57, 0, 255, 255, 66, 0, 1, 58, 0, 255, 255, 67, 0, 1, 59, 0, 255, 255, 68, 0, 1, 60, 0, 255, 255, 69, 0, 1, 66
|
|
, 0, 255, 255, 70, 0, 1, 67, 0, 255, 255, 71, 0, 1, 69, 0, 141, 0, 0, 0, 0, 70, 0, 255, 255, 74, 0, 1, 68, 0, 255
|
|
, 255, 72, 0, 1, 69, 0, 255, 255, 73, 0, 1, 77, 0, 145, 0, 0, 0, 0, 84, 0, 157, 0, 0, 0, 0, 74, 0, 147, 0, 0
|
|
, 0, 0, 77, 0, 153, 0, 0, 0, 0, 72, 0, 149, 0, 0, 0, 0, 74, 0, 151, 0, 0, 0, 0, 71, 0, 255, 255, 75, 0, 1
|
|
, 72, 0, 255, 255, 76, 0, 1, 73, 0, 255, 255, 77, 0, 1, 74, 0, 255, 255, 78, 0, 1, 76, 0, 155, 0, 0, 0, 0, 77, 0
|
|
, 255, 255, 81, 0, 1, 75, 0, 255, 255, 79, 0, 1, 76, 0, 255, 255, 80, 0, 1, 81, 0, 159, 0, 0, 0, 0, 84, 0, 165, 0
|
|
, 0, 0, 0, 79, 0, 161, 0, 0, 0, 0, 81, 0, 163, 0, 0, 0, 0, 78, 0, 255, 255, 82, 0, 1, 79, 0, 255, 255, 83, 0
|
|
, 1, 80, 0, 255, 255, 84, 0, 1, 81, 0, 255, 255, 85, 0, 1, 83, 0, 167, 0, 0, 0, 0, 84, 0, 255, 255, 88, 0, 1, 82
|
|
, 0, 255, 255, 86, 0, 1, 83, 0, 255, 255, 87, 0, 1, 98, 0, 171, 0, 0, 0, 0, 111, 0, 197, 0, 0, 0, 0, 91, 0, 173
|
|
, 0, 0, 0, 0, 98, 0, 185, 0, 0, 0, 0, 88, 0, 175, 0, 0, 0, 0, 91, 0, 181, 0, 0, 0, 0, 86, 0, 177, 0, 0
|
|
, 0, 0, 88, 0, 179, 0, 0, 0, 0, 85, 0, 255, 255, 98, 0, 1, 86, 0, 255, 255, 89, 0, 1, 87, 0, 255, 255, 90, 0, 1
|
|
, 88, 0, 255, 255, 91, 0, 1, 90, 0, 183, 0, 0, 0, 0, 91, 0, 255, 255, 94, 0, 1, 89, 0, 255, 255, 92, 0, 1, 90, 0
|
|
, 255, 255, 93, 0, 1, 95, 0, 187, 0, 0, 0, 0, 98, 0, 193, 0, 0, 0, 0, 93, 0, 189, 0, 0, 0, 0, 95, 0, 191, 0
|
|
, 0, 0, 0, 92, 0, 255, 255, 95, 0, 1, 93, 0, 255, 255, 96, 0, 1, 94, 0, 255, 255, 97, 0, 1, 95, 0, 255, 255, 99, 0
|
|
, 1, 97, 0, 195, 0, 0, 0, 0, 98, 0, 255, 255, 102, 0, 1, 96, 0, 255, 255, 100, 0, 1, 97, 0, 255, 255, 101, 0, 1, 105
|
|
, 0, 199, 0, 0, 0, 0, 111, 0, 211, 0, 0, 0, 0, 102, 0, 201, 0, 0, 0, 0, 105, 0, 207, 0, 0, 0, 0, 100, 0, 203
|
|
, 0, 0, 0, 0, 102, 0, 205, 0, 0, 0, 0, 99, 0, 255, 255, 103, 0, 1, 100, 0, 255, 255, 104, 0, 1, 101, 0, 255, 255, 105
|
|
, 0, 1, 102, 0, 255, 255, 106, 0, 1, 104, 0, 209, 0, 0, 0, 0, 105, 0, 255, 255, 109, 0, 1, 103, 0, 255, 255, 107, 0, 1
|
|
, 104, 0, 255, 255, 108, 0, 1, 108, 0, 213, 0, 0, 0, 0, 111, 0, 217, 0, 0, 0, 0, 107, 0, 215, 0, 0, 0, 0, 108, 0
|
|
, 255, 255, 112, 0, 1, 106, 0, 255, 255, 110, 0, 1, 107, 0, 255, 255, 111, 0, 1, 110, 0, 219, 0, 0, 0, 0, 111, 0, 221, 0
|
|
, 115, 0, 1, 109, 0, 255, 255, 113, 0, 1, 110, 0, 255, 255, 114, 0, 1, 111, 0, 255, 255, 0, 0, 0, 111, 0, 223, 0, 0, 0
|
|
, 0, 112, 0, 255, 255, 116, 0, 1, 111, 0, 255, 255, 0, 0, 0
|
|
}, new ushort[]
|
|
{
|
|
// Control tree node indicies
|
|
|
|
0, 64, 0, 64, 1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15, 16, 17, 18, 19, 20, 21, 22, 23, 24, 25, 26
|
|
, 27, 28, 29, 30, 31, 32, 33, 34, 35, 36, 37, 38, 39, 40, 41, 42, 43, 44, 45, 46, 47, 48, 49, 50, 51, 52, 53, 54, 55, 56
|
|
, 57, 58, 59, 60, 61, 62, 63, 65, 66, 67, 68, 69, 70, 71, 72, 73, 74, 75, 76, 77, 78, 79, 80, 81, 82, 83, 84, 85, 86, 87
|
|
, 88, 89, 90, 91, 92, 93, 94, 95, 96, 97, 98, 99, 100, 101, 102, 103, 104, 105, 106, 107, 108, 109, 110, 111, 112, 113, 114
|
|
});
|
|
|
|
builder.Finish();
|
|
}
|
|
|
|
private UnityEngine.InputSystem.Controls.AnyKeyControl Initialize_ctrlKeyboardanyKey(InternedString kAnyKeyLayout, InputControl parent)
|
|
{
|
|
var ctrlKeyboardanyKey = new UnityEngine.InputSystem.Controls.AnyKeyControl();
|
|
ctrlKeyboardanyKey.Setup()
|
|
.At(this, 0)
|
|
.WithParent(parent)
|
|
.WithName("anyKey")
|
|
.WithDisplayName("Any Key")
|
|
.WithLayout(kAnyKeyLayout)
|
|
.IsSynthetic(true)
|
|
.IsButton(true)
|
|
.WithStateBlock(new InputStateBlock
|
|
{
|
|
format = new FourCC(1112101920),
|
|
byteOffset = 0,
|
|
bitOffset = 0,
|
|
sizeInBits = 109
|
|
})
|
|
.WithMinAndMax(0, 1)
|
|
.Finish();
|
|
return ctrlKeyboardanyKey;
|
|
}
|
|
|
|
private UnityEngine.InputSystem.Controls.KeyControl Initialize_ctrlKeyboardescape(InternedString kKeyLayout, InputControl parent)
|
|
{
|
|
var ctrlKeyboardescape = new UnityEngine.InputSystem.Controls.KeyControl();
|
|
ctrlKeyboardescape.Setup()
|
|
.At(this, 1)
|
|
.WithParent(parent)
|
|
.WithName("escape")
|
|
.WithDisplayName("Escape")
|
|
.WithLayout(kKeyLayout)
|
|
.WithUsages(0, 2)
|
|
.IsButton(true)
|
|
.WithStateBlock(new InputStateBlock
|
|
{
|
|
format = new FourCC(1112101920),
|
|
byteOffset = 0,
|
|
bitOffset = 60,
|
|
sizeInBits = 1
|
|
})
|
|
.WithMinAndMax(0, 1)
|
|
.Finish();
|
|
ctrlKeyboardescape.keyCode = UnityEngine.InputSystem.Key.Escape;
|
|
return ctrlKeyboardescape;
|
|
}
|
|
|
|
private UnityEngine.InputSystem.Controls.KeyControl Initialize_ctrlKeyboardspace(InternedString kKeyLayout, InputControl parent)
|
|
{
|
|
var ctrlKeyboardspace = new UnityEngine.InputSystem.Controls.KeyControl();
|
|
ctrlKeyboardspace.Setup()
|
|
.At(this, 2)
|
|
.WithParent(parent)
|
|
.WithName("space")
|
|
.WithDisplayName("Space")
|
|
.WithLayout(kKeyLayout)
|
|
.IsButton(true)
|
|
.WithStateBlock(new InputStateBlock
|
|
{
|
|
format = new FourCC(1112101920),
|
|
byteOffset = 0,
|
|
bitOffset = 1,
|
|
sizeInBits = 1
|
|
})
|
|
.WithMinAndMax(0, 1)
|
|
.Finish();
|
|
ctrlKeyboardspace.keyCode = UnityEngine.InputSystem.Key.Space;
|
|
return ctrlKeyboardspace;
|
|
}
|
|
|
|
private UnityEngine.InputSystem.Controls.KeyControl Initialize_ctrlKeyboardenter(InternedString kKeyLayout, InputControl parent)
|
|
{
|
|
var ctrlKeyboardenter = new UnityEngine.InputSystem.Controls.KeyControl();
|
|
ctrlKeyboardenter.Setup()
|
|
.At(this, 3)
|
|
.WithParent(parent)
|
|
.WithName("enter")
|
|
.WithDisplayName("Enter")
|
|
.WithLayout(kKeyLayout)
|
|
.WithUsages(2, 1)
|
|
.IsButton(true)
|
|
.WithStateBlock(new InputStateBlock
|
|
{
|
|
format = new FourCC(1112101920),
|
|
byteOffset = 0,
|
|
bitOffset = 2,
|
|
sizeInBits = 1
|
|
})
|
|
.WithMinAndMax(0, 1)
|
|
.Finish();
|
|
ctrlKeyboardenter.keyCode = UnityEngine.InputSystem.Key.Enter;
|
|
return ctrlKeyboardenter;
|
|
}
|
|
|
|
private UnityEngine.InputSystem.Controls.KeyControl Initialize_ctrlKeyboardtab(InternedString kKeyLayout, InputControl parent)
|
|
{
|
|
var ctrlKeyboardtab = new UnityEngine.InputSystem.Controls.KeyControl();
|
|
ctrlKeyboardtab.Setup()
|
|
.At(this, 4)
|
|
.WithParent(parent)
|
|
.WithName("tab")
|
|
.WithDisplayName("Tab")
|
|
.WithLayout(kKeyLayout)
|
|
.IsButton(true)
|
|
.WithStateBlock(new InputStateBlock
|
|
{
|
|
format = new FourCC(1112101920),
|
|
byteOffset = 0,
|
|
bitOffset = 3,
|
|
sizeInBits = 1
|
|
})
|
|
.WithMinAndMax(0, 1)
|
|
.Finish();
|
|
ctrlKeyboardtab.keyCode = UnityEngine.InputSystem.Key.Tab;
|
|
return ctrlKeyboardtab;
|
|
}
|
|
|
|
private UnityEngine.InputSystem.Controls.KeyControl Initialize_ctrlKeyboardbackquote(InternedString kKeyLayout, InputControl parent)
|
|
{
|
|
var ctrlKeyboardbackquote = new UnityEngine.InputSystem.Controls.KeyControl();
|
|
ctrlKeyboardbackquote.Setup()
|
|
.At(this, 5)
|
|
.WithParent(parent)
|
|
.WithName("backquote")
|
|
.WithDisplayName("`")
|
|
.WithLayout(kKeyLayout)
|
|
.IsButton(true)
|
|
.WithStateBlock(new InputStateBlock
|
|
{
|
|
format = new FourCC(1112101920),
|
|
byteOffset = 0,
|
|
bitOffset = 4,
|
|
sizeInBits = 1
|
|
})
|
|
.WithMinAndMax(0, 1)
|
|
.Finish();
|
|
ctrlKeyboardbackquote.keyCode = UnityEngine.InputSystem.Key.Backquote;
|
|
return ctrlKeyboardbackquote;
|
|
}
|
|
|
|
private UnityEngine.InputSystem.Controls.KeyControl Initialize_ctrlKeyboardquote(InternedString kKeyLayout, InputControl parent)
|
|
{
|
|
var ctrlKeyboardquote = new UnityEngine.InputSystem.Controls.KeyControl();
|
|
ctrlKeyboardquote.Setup()
|
|
.At(this, 6)
|
|
.WithParent(parent)
|
|
.WithName("quote")
|
|
.WithDisplayName("'")
|
|
.WithLayout(kKeyLayout)
|
|
.IsButton(true)
|
|
.WithStateBlock(new InputStateBlock
|
|
{
|
|
format = new FourCC(1112101920),
|
|
byteOffset = 0,
|
|
bitOffset = 5,
|
|
sizeInBits = 1
|
|
})
|
|
.WithMinAndMax(0, 1)
|
|
.Finish();
|
|
ctrlKeyboardquote.keyCode = UnityEngine.InputSystem.Key.Quote;
|
|
return ctrlKeyboardquote;
|
|
}
|
|
|
|
private UnityEngine.InputSystem.Controls.KeyControl Initialize_ctrlKeyboardsemicolon(InternedString kKeyLayout, InputControl parent)
|
|
{
|
|
var ctrlKeyboardsemicolon = new UnityEngine.InputSystem.Controls.KeyControl();
|
|
ctrlKeyboardsemicolon.Setup()
|
|
.At(this, 7)
|
|
.WithParent(parent)
|
|
.WithName("semicolon")
|
|
.WithDisplayName(";")
|
|
.WithLayout(kKeyLayout)
|
|
.IsButton(true)
|
|
.WithStateBlock(new InputStateBlock
|
|
{
|
|
format = new FourCC(1112101920),
|
|
byteOffset = 0,
|
|
bitOffset = 6,
|
|
sizeInBits = 1
|
|
})
|
|
.WithMinAndMax(0, 1)
|
|
.Finish();
|
|
ctrlKeyboardsemicolon.keyCode = UnityEngine.InputSystem.Key.Semicolon;
|
|
return ctrlKeyboardsemicolon;
|
|
}
|
|
|
|
private UnityEngine.InputSystem.Controls.KeyControl Initialize_ctrlKeyboardcomma(InternedString kKeyLayout, InputControl parent)
|
|
{
|
|
var ctrlKeyboardcomma = new UnityEngine.InputSystem.Controls.KeyControl();
|
|
ctrlKeyboardcomma.Setup()
|
|
.At(this, 8)
|
|
.WithParent(parent)
|
|
.WithName("comma")
|
|
.WithDisplayName(",")
|
|
.WithLayout(kKeyLayout)
|
|
.IsButton(true)
|
|
.WithStateBlock(new InputStateBlock
|
|
{
|
|
format = new FourCC(1112101920),
|
|
byteOffset = 0,
|
|
bitOffset = 7,
|
|
sizeInBits = 1
|
|
})
|
|
.WithMinAndMax(0, 1)
|
|
.Finish();
|
|
ctrlKeyboardcomma.keyCode = UnityEngine.InputSystem.Key.Comma;
|
|
return ctrlKeyboardcomma;
|
|
}
|
|
|
|
private UnityEngine.InputSystem.Controls.KeyControl Initialize_ctrlKeyboardperiod(InternedString kKeyLayout, InputControl parent)
|
|
{
|
|
var ctrlKeyboardperiod = new UnityEngine.InputSystem.Controls.KeyControl();
|
|
ctrlKeyboardperiod.Setup()
|
|
.At(this, 9)
|
|
.WithParent(parent)
|
|
.WithName("period")
|
|
.WithDisplayName(".")
|
|
.WithLayout(kKeyLayout)
|
|
.IsButton(true)
|
|
.WithStateBlock(new InputStateBlock
|
|
{
|
|
format = new FourCC(1112101920),
|
|
byteOffset = 0,
|
|
bitOffset = 8,
|
|
sizeInBits = 1
|
|
})
|
|
.WithMinAndMax(0, 1)
|
|
.Finish();
|
|
ctrlKeyboardperiod.keyCode = UnityEngine.InputSystem.Key.Period;
|
|
return ctrlKeyboardperiod;
|
|
}
|
|
|
|
private UnityEngine.InputSystem.Controls.KeyControl Initialize_ctrlKeyboardslash(InternedString kKeyLayout, InputControl parent)
|
|
{
|
|
var ctrlKeyboardslash = new UnityEngine.InputSystem.Controls.KeyControl();
|
|
ctrlKeyboardslash.Setup()
|
|
.At(this, 10)
|
|
.WithParent(parent)
|
|
.WithName("slash")
|
|
.WithDisplayName("/")
|
|
.WithLayout(kKeyLayout)
|
|
.IsButton(true)
|
|
.WithStateBlock(new InputStateBlock
|
|
{
|
|
format = new FourCC(1112101920),
|
|
byteOffset = 0,
|
|
bitOffset = 9,
|
|
sizeInBits = 1
|
|
})
|
|
.WithMinAndMax(0, 1)
|
|
.Finish();
|
|
ctrlKeyboardslash.keyCode = UnityEngine.InputSystem.Key.Slash;
|
|
return ctrlKeyboardslash;
|
|
}
|
|
|
|
private UnityEngine.InputSystem.Controls.KeyControl Initialize_ctrlKeyboardbackslash(InternedString kKeyLayout, InputControl parent)
|
|
{
|
|
var ctrlKeyboardbackslash = new UnityEngine.InputSystem.Controls.KeyControl();
|
|
ctrlKeyboardbackslash.Setup()
|
|
.At(this, 11)
|
|
.WithParent(parent)
|
|
.WithName("backslash")
|
|
.WithDisplayName("\\")
|
|
.WithLayout(kKeyLayout)
|
|
.IsButton(true)
|
|
.WithStateBlock(new InputStateBlock
|
|
{
|
|
format = new FourCC(1112101920),
|
|
byteOffset = 0,
|
|
bitOffset = 10,
|
|
sizeInBits = 1
|
|
})
|
|
.WithMinAndMax(0, 1)
|
|
.Finish();
|
|
ctrlKeyboardbackslash.keyCode = UnityEngine.InputSystem.Key.Backslash;
|
|
return ctrlKeyboardbackslash;
|
|
}
|
|
|
|
private UnityEngine.InputSystem.Controls.KeyControl Initialize_ctrlKeyboardleftBracket(InternedString kKeyLayout, InputControl parent)
|
|
{
|
|
var ctrlKeyboardleftBracket = new UnityEngine.InputSystem.Controls.KeyControl();
|
|
ctrlKeyboardleftBracket.Setup()
|
|
.At(this, 12)
|
|
.WithParent(parent)
|
|
.WithName("leftBracket")
|
|
.WithDisplayName("[")
|
|
.WithLayout(kKeyLayout)
|
|
.IsButton(true)
|
|
.WithStateBlock(new InputStateBlock
|
|
{
|
|
format = new FourCC(1112101920),
|
|
byteOffset = 0,
|
|
bitOffset = 11,
|
|
sizeInBits = 1
|
|
})
|
|
.WithMinAndMax(0, 1)
|
|
.Finish();
|
|
ctrlKeyboardleftBracket.keyCode = UnityEngine.InputSystem.Key.LeftBracket;
|
|
return ctrlKeyboardleftBracket;
|
|
}
|
|
|
|
private UnityEngine.InputSystem.Controls.KeyControl Initialize_ctrlKeyboardrightBracket(InternedString kKeyLayout, InputControl parent)
|
|
{
|
|
var ctrlKeyboardrightBracket = new UnityEngine.InputSystem.Controls.KeyControl();
|
|
ctrlKeyboardrightBracket.Setup()
|
|
.At(this, 13)
|
|
.WithParent(parent)
|
|
.WithName("rightBracket")
|
|
.WithDisplayName("]")
|
|
.WithLayout(kKeyLayout)
|
|
.IsButton(true)
|
|
.WithStateBlock(new InputStateBlock
|
|
{
|
|
format = new FourCC(1112101920),
|
|
byteOffset = 0,
|
|
bitOffset = 12,
|
|
sizeInBits = 1
|
|
})
|
|
.WithMinAndMax(0, 1)
|
|
.Finish();
|
|
ctrlKeyboardrightBracket.keyCode = UnityEngine.InputSystem.Key.RightBracket;
|
|
return ctrlKeyboardrightBracket;
|
|
}
|
|
|
|
private UnityEngine.InputSystem.Controls.KeyControl Initialize_ctrlKeyboardminus(InternedString kKeyLayout, InputControl parent)
|
|
{
|
|
var ctrlKeyboardminus = new UnityEngine.InputSystem.Controls.KeyControl();
|
|
ctrlKeyboardminus.Setup()
|
|
.At(this, 14)
|
|
.WithParent(parent)
|
|
.WithName("minus")
|
|
.WithDisplayName("-")
|
|
.WithLayout(kKeyLayout)
|
|
.IsButton(true)
|
|
.WithStateBlock(new InputStateBlock
|
|
{
|
|
format = new FourCC(1112101920),
|
|
byteOffset = 0,
|
|
bitOffset = 13,
|
|
sizeInBits = 1
|
|
})
|
|
.WithMinAndMax(0, 1)
|
|
.Finish();
|
|
ctrlKeyboardminus.keyCode = UnityEngine.InputSystem.Key.Minus;
|
|
return ctrlKeyboardminus;
|
|
}
|
|
|
|
private UnityEngine.InputSystem.Controls.KeyControl Initialize_ctrlKeyboardequals(InternedString kKeyLayout, InputControl parent)
|
|
{
|
|
var ctrlKeyboardequals = new UnityEngine.InputSystem.Controls.KeyControl();
|
|
ctrlKeyboardequals.Setup()
|
|
.At(this, 15)
|
|
.WithParent(parent)
|
|
.WithName("equals")
|
|
.WithDisplayName("=")
|
|
.WithLayout(kKeyLayout)
|
|
.IsButton(true)
|
|
.WithStateBlock(new InputStateBlock
|
|
{
|
|
format = new FourCC(1112101920),
|
|
byteOffset = 0,
|
|
bitOffset = 14,
|
|
sizeInBits = 1
|
|
})
|
|
.WithMinAndMax(0, 1)
|
|
.Finish();
|
|
ctrlKeyboardequals.keyCode = UnityEngine.InputSystem.Key.Equals;
|
|
return ctrlKeyboardequals;
|
|
}
|
|
|
|
private UnityEngine.InputSystem.Controls.KeyControl Initialize_ctrlKeyboardupArrow(InternedString kKeyLayout, InputControl parent)
|
|
{
|
|
var ctrlKeyboardupArrow = new UnityEngine.InputSystem.Controls.KeyControl();
|
|
ctrlKeyboardupArrow.Setup()
|
|
.At(this, 16)
|
|
.WithParent(parent)
|
|
.WithName("upArrow")
|
|
.WithDisplayName("Up Arrow")
|
|
.WithLayout(kKeyLayout)
|
|
.IsButton(true)
|
|
.WithStateBlock(new InputStateBlock
|
|
{
|
|
format = new FourCC(1112101920),
|
|
byteOffset = 0,
|
|
bitOffset = 63,
|
|
sizeInBits = 1
|
|
})
|
|
.WithMinAndMax(0, 1)
|
|
.Finish();
|
|
ctrlKeyboardupArrow.keyCode = UnityEngine.InputSystem.Key.UpArrow;
|
|
return ctrlKeyboardupArrow;
|
|
}
|
|
|
|
private UnityEngine.InputSystem.Controls.KeyControl Initialize_ctrlKeyboarddownArrow(InternedString kKeyLayout, InputControl parent)
|
|
{
|
|
var ctrlKeyboarddownArrow = new UnityEngine.InputSystem.Controls.KeyControl();
|
|
ctrlKeyboarddownArrow.Setup()
|
|
.At(this, 17)
|
|
.WithParent(parent)
|
|
.WithName("downArrow")
|
|
.WithDisplayName("Down Arrow")
|
|
.WithLayout(kKeyLayout)
|
|
.IsButton(true)
|
|
.WithStateBlock(new InputStateBlock
|
|
{
|
|
format = new FourCC(1112101920),
|
|
byteOffset = 0,
|
|
bitOffset = 64,
|
|
sizeInBits = 1
|
|
})
|
|
.WithMinAndMax(0, 1)
|
|
.Finish();
|
|
ctrlKeyboarddownArrow.keyCode = UnityEngine.InputSystem.Key.DownArrow;
|
|
return ctrlKeyboarddownArrow;
|
|
}
|
|
|
|
private UnityEngine.InputSystem.Controls.KeyControl Initialize_ctrlKeyboardleftArrow(InternedString kKeyLayout, InputControl parent)
|
|
{
|
|
var ctrlKeyboardleftArrow = new UnityEngine.InputSystem.Controls.KeyControl();
|
|
ctrlKeyboardleftArrow.Setup()
|
|
.At(this, 18)
|
|
.WithParent(parent)
|
|
.WithName("leftArrow")
|
|
.WithDisplayName("Left Arrow")
|
|
.WithLayout(kKeyLayout)
|
|
.IsButton(true)
|
|
.WithStateBlock(new InputStateBlock
|
|
{
|
|
format = new FourCC(1112101920),
|
|
byteOffset = 0,
|
|
bitOffset = 61,
|
|
sizeInBits = 1
|
|
})
|
|
.WithMinAndMax(0, 1)
|
|
.Finish();
|
|
ctrlKeyboardleftArrow.keyCode = UnityEngine.InputSystem.Key.LeftArrow;
|
|
return ctrlKeyboardleftArrow;
|
|
}
|
|
|
|
private UnityEngine.InputSystem.Controls.KeyControl Initialize_ctrlKeyboardrightArrow(InternedString kKeyLayout, InputControl parent)
|
|
{
|
|
var ctrlKeyboardrightArrow = new UnityEngine.InputSystem.Controls.KeyControl();
|
|
ctrlKeyboardrightArrow.Setup()
|
|
.At(this, 19)
|
|
.WithParent(parent)
|
|
.WithName("rightArrow")
|
|
.WithDisplayName("Right Arrow")
|
|
.WithLayout(kKeyLayout)
|
|
.IsButton(true)
|
|
.WithStateBlock(new InputStateBlock
|
|
{
|
|
format = new FourCC(1112101920),
|
|
byteOffset = 0,
|
|
bitOffset = 62,
|
|
sizeInBits = 1
|
|
})
|
|
.WithMinAndMax(0, 1)
|
|
.Finish();
|
|
ctrlKeyboardrightArrow.keyCode = UnityEngine.InputSystem.Key.RightArrow;
|
|
return ctrlKeyboardrightArrow;
|
|
}
|
|
|
|
private UnityEngine.InputSystem.Controls.KeyControl Initialize_ctrlKeyboarda(InternedString kKeyLayout, InputControl parent)
|
|
{
|
|
var ctrlKeyboarda = new UnityEngine.InputSystem.Controls.KeyControl();
|
|
ctrlKeyboarda.Setup()
|
|
.At(this, 20)
|
|
.WithParent(parent)
|
|
.WithName("a")
|
|
.WithDisplayName("A")
|
|
.WithLayout(kKeyLayout)
|
|
.IsButton(true)
|
|
.WithStateBlock(new InputStateBlock
|
|
{
|
|
format = new FourCC(1112101920),
|
|
byteOffset = 0,
|
|
bitOffset = 15,
|
|
sizeInBits = 1
|
|
})
|
|
.WithMinAndMax(0, 1)
|
|
.Finish();
|
|
ctrlKeyboarda.keyCode = UnityEngine.InputSystem.Key.A;
|
|
return ctrlKeyboarda;
|
|
}
|
|
|
|
private UnityEngine.InputSystem.Controls.KeyControl Initialize_ctrlKeyboardb(InternedString kKeyLayout, InputControl parent)
|
|
{
|
|
var ctrlKeyboardb = new UnityEngine.InputSystem.Controls.KeyControl();
|
|
ctrlKeyboardb.Setup()
|
|
.At(this, 21)
|
|
.WithParent(parent)
|
|
.WithName("b")
|
|
.WithDisplayName("B")
|
|
.WithLayout(kKeyLayout)
|
|
.IsButton(true)
|
|
.WithStateBlock(new InputStateBlock
|
|
{
|
|
format = new FourCC(1112101920),
|
|
byteOffset = 0,
|
|
bitOffset = 16,
|
|
sizeInBits = 1
|
|
})
|
|
.WithMinAndMax(0, 1)
|
|
.Finish();
|
|
ctrlKeyboardb.keyCode = UnityEngine.InputSystem.Key.B;
|
|
return ctrlKeyboardb;
|
|
}
|
|
|
|
private UnityEngine.InputSystem.Controls.KeyControl Initialize_ctrlKeyboardc(InternedString kKeyLayout, InputControl parent)
|
|
{
|
|
var ctrlKeyboardc = new UnityEngine.InputSystem.Controls.KeyControl();
|
|
ctrlKeyboardc.Setup()
|
|
.At(this, 22)
|
|
.WithParent(parent)
|
|
.WithName("c")
|
|
.WithDisplayName("C")
|
|
.WithLayout(kKeyLayout)
|
|
.IsButton(true)
|
|
.WithStateBlock(new InputStateBlock
|
|
{
|
|
format = new FourCC(1112101920),
|
|
byteOffset = 0,
|
|
bitOffset = 17,
|
|
sizeInBits = 1
|
|
})
|
|
.WithMinAndMax(0, 1)
|
|
.Finish();
|
|
ctrlKeyboardc.keyCode = UnityEngine.InputSystem.Key.C;
|
|
return ctrlKeyboardc;
|
|
}
|
|
|
|
private UnityEngine.InputSystem.Controls.KeyControl Initialize_ctrlKeyboardd(InternedString kKeyLayout, InputControl parent)
|
|
{
|
|
var ctrlKeyboardd = new UnityEngine.InputSystem.Controls.KeyControl();
|
|
ctrlKeyboardd.Setup()
|
|
.At(this, 23)
|
|
.WithParent(parent)
|
|
.WithName("d")
|
|
.WithDisplayName("D")
|
|
.WithLayout(kKeyLayout)
|
|
.IsButton(true)
|
|
.WithStateBlock(new InputStateBlock
|
|
{
|
|
format = new FourCC(1112101920),
|
|
byteOffset = 0,
|
|
bitOffset = 18,
|
|
sizeInBits = 1
|
|
})
|
|
.WithMinAndMax(0, 1)
|
|
.Finish();
|
|
ctrlKeyboardd.keyCode = UnityEngine.InputSystem.Key.D;
|
|
return ctrlKeyboardd;
|
|
}
|
|
|
|
private UnityEngine.InputSystem.Controls.KeyControl Initialize_ctrlKeyboarde(InternedString kKeyLayout, InputControl parent)
|
|
{
|
|
var ctrlKeyboarde = new UnityEngine.InputSystem.Controls.KeyControl();
|
|
ctrlKeyboarde.Setup()
|
|
.At(this, 24)
|
|
.WithParent(parent)
|
|
.WithName("e")
|
|
.WithDisplayName("E")
|
|
.WithLayout(kKeyLayout)
|
|
.IsButton(true)
|
|
.WithStateBlock(new InputStateBlock
|
|
{
|
|
format = new FourCC(1112101920),
|
|
byteOffset = 0,
|
|
bitOffset = 19,
|
|
sizeInBits = 1
|
|
})
|
|
.WithMinAndMax(0, 1)
|
|
.Finish();
|
|
ctrlKeyboarde.keyCode = UnityEngine.InputSystem.Key.E;
|
|
return ctrlKeyboarde;
|
|
}
|
|
|
|
private UnityEngine.InputSystem.Controls.KeyControl Initialize_ctrlKeyboardf(InternedString kKeyLayout, InputControl parent)
|
|
{
|
|
var ctrlKeyboardf = new UnityEngine.InputSystem.Controls.KeyControl();
|
|
ctrlKeyboardf.Setup()
|
|
.At(this, 25)
|
|
.WithParent(parent)
|
|
.WithName("f")
|
|
.WithDisplayName("F")
|
|
.WithLayout(kKeyLayout)
|
|
.IsButton(true)
|
|
.WithStateBlock(new InputStateBlock
|
|
{
|
|
format = new FourCC(1112101920),
|
|
byteOffset = 0,
|
|
bitOffset = 20,
|
|
sizeInBits = 1
|
|
})
|
|
.WithMinAndMax(0, 1)
|
|
.Finish();
|
|
ctrlKeyboardf.keyCode = UnityEngine.InputSystem.Key.F;
|
|
return ctrlKeyboardf;
|
|
}
|
|
|
|
private UnityEngine.InputSystem.Controls.KeyControl Initialize_ctrlKeyboardg(InternedString kKeyLayout, InputControl parent)
|
|
{
|
|
var ctrlKeyboardg = new UnityEngine.InputSystem.Controls.KeyControl();
|
|
ctrlKeyboardg.Setup()
|
|
.At(this, 26)
|
|
.WithParent(parent)
|
|
.WithName("g")
|
|
.WithDisplayName("G")
|
|
.WithLayout(kKeyLayout)
|
|
.IsButton(true)
|
|
.WithStateBlock(new InputStateBlock
|
|
{
|
|
format = new FourCC(1112101920),
|
|
byteOffset = 0,
|
|
bitOffset = 21,
|
|
sizeInBits = 1
|
|
})
|
|
.WithMinAndMax(0, 1)
|
|
.Finish();
|
|
ctrlKeyboardg.keyCode = UnityEngine.InputSystem.Key.G;
|
|
return ctrlKeyboardg;
|
|
}
|
|
|
|
private UnityEngine.InputSystem.Controls.KeyControl Initialize_ctrlKeyboardh(InternedString kKeyLayout, InputControl parent)
|
|
{
|
|
var ctrlKeyboardh = new UnityEngine.InputSystem.Controls.KeyControl();
|
|
ctrlKeyboardh.Setup()
|
|
.At(this, 27)
|
|
.WithParent(parent)
|
|
.WithName("h")
|
|
.WithDisplayName("H")
|
|
.WithLayout(kKeyLayout)
|
|
.IsButton(true)
|
|
.WithStateBlock(new InputStateBlock
|
|
{
|
|
format = new FourCC(1112101920),
|
|
byteOffset = 0,
|
|
bitOffset = 22,
|
|
sizeInBits = 1
|
|
})
|
|
.WithMinAndMax(0, 1)
|
|
.Finish();
|
|
ctrlKeyboardh.keyCode = UnityEngine.InputSystem.Key.H;
|
|
return ctrlKeyboardh;
|
|
}
|
|
|
|
private UnityEngine.InputSystem.Controls.KeyControl Initialize_ctrlKeyboardi(InternedString kKeyLayout, InputControl parent)
|
|
{
|
|
var ctrlKeyboardi = new UnityEngine.InputSystem.Controls.KeyControl();
|
|
ctrlKeyboardi.Setup()
|
|
.At(this, 28)
|
|
.WithParent(parent)
|
|
.WithName("i")
|
|
.WithDisplayName("I")
|
|
.WithLayout(kKeyLayout)
|
|
.IsButton(true)
|
|
.WithStateBlock(new InputStateBlock
|
|
{
|
|
format = new FourCC(1112101920),
|
|
byteOffset = 0,
|
|
bitOffset = 23,
|
|
sizeInBits = 1
|
|
})
|
|
.WithMinAndMax(0, 1)
|
|
.Finish();
|
|
ctrlKeyboardi.keyCode = UnityEngine.InputSystem.Key.I;
|
|
return ctrlKeyboardi;
|
|
}
|
|
|
|
private UnityEngine.InputSystem.Controls.KeyControl Initialize_ctrlKeyboardj(InternedString kKeyLayout, InputControl parent)
|
|
{
|
|
var ctrlKeyboardj = new UnityEngine.InputSystem.Controls.KeyControl();
|
|
ctrlKeyboardj.Setup()
|
|
.At(this, 29)
|
|
.WithParent(parent)
|
|
.WithName("j")
|
|
.WithDisplayName("J")
|
|
.WithLayout(kKeyLayout)
|
|
.IsButton(true)
|
|
.WithStateBlock(new InputStateBlock
|
|
{
|
|
format = new FourCC(1112101920),
|
|
byteOffset = 0,
|
|
bitOffset = 24,
|
|
sizeInBits = 1
|
|
})
|
|
.WithMinAndMax(0, 1)
|
|
.Finish();
|
|
ctrlKeyboardj.keyCode = UnityEngine.InputSystem.Key.J;
|
|
return ctrlKeyboardj;
|
|
}
|
|
|
|
private UnityEngine.InputSystem.Controls.KeyControl Initialize_ctrlKeyboardk(InternedString kKeyLayout, InputControl parent)
|
|
{
|
|
var ctrlKeyboardk = new UnityEngine.InputSystem.Controls.KeyControl();
|
|
ctrlKeyboardk.Setup()
|
|
.At(this, 30)
|
|
.WithParent(parent)
|
|
.WithName("k")
|
|
.WithDisplayName("K")
|
|
.WithLayout(kKeyLayout)
|
|
.IsButton(true)
|
|
.WithStateBlock(new InputStateBlock
|
|
{
|
|
format = new FourCC(1112101920),
|
|
byteOffset = 0,
|
|
bitOffset = 25,
|
|
sizeInBits = 1
|
|
})
|
|
.WithMinAndMax(0, 1)
|
|
.Finish();
|
|
ctrlKeyboardk.keyCode = UnityEngine.InputSystem.Key.K;
|
|
return ctrlKeyboardk;
|
|
}
|
|
|
|
private UnityEngine.InputSystem.Controls.KeyControl Initialize_ctrlKeyboardl(InternedString kKeyLayout, InputControl parent)
|
|
{
|
|
var ctrlKeyboardl = new UnityEngine.InputSystem.Controls.KeyControl();
|
|
ctrlKeyboardl.Setup()
|
|
.At(this, 31)
|
|
.WithParent(parent)
|
|
.WithName("l")
|
|
.WithDisplayName("L")
|
|
.WithLayout(kKeyLayout)
|
|
.IsButton(true)
|
|
.WithStateBlock(new InputStateBlock
|
|
{
|
|
format = new FourCC(1112101920),
|
|
byteOffset = 0,
|
|
bitOffset = 26,
|
|
sizeInBits = 1
|
|
})
|
|
.WithMinAndMax(0, 1)
|
|
.Finish();
|
|
ctrlKeyboardl.keyCode = UnityEngine.InputSystem.Key.L;
|
|
return ctrlKeyboardl;
|
|
}
|
|
|
|
private UnityEngine.InputSystem.Controls.KeyControl Initialize_ctrlKeyboardm(InternedString kKeyLayout, InputControl parent)
|
|
{
|
|
var ctrlKeyboardm = new UnityEngine.InputSystem.Controls.KeyControl();
|
|
ctrlKeyboardm.Setup()
|
|
.At(this, 32)
|
|
.WithParent(parent)
|
|
.WithName("m")
|
|
.WithDisplayName("M")
|
|
.WithLayout(kKeyLayout)
|
|
.IsButton(true)
|
|
.WithStateBlock(new InputStateBlock
|
|
{
|
|
format = new FourCC(1112101920),
|
|
byteOffset = 0,
|
|
bitOffset = 27,
|
|
sizeInBits = 1
|
|
})
|
|
.WithMinAndMax(0, 1)
|
|
.Finish();
|
|
ctrlKeyboardm.keyCode = UnityEngine.InputSystem.Key.M;
|
|
return ctrlKeyboardm;
|
|
}
|
|
|
|
private UnityEngine.InputSystem.Controls.KeyControl Initialize_ctrlKeyboardn(InternedString kKeyLayout, InputControl parent)
|
|
{
|
|
var ctrlKeyboardn = new UnityEngine.InputSystem.Controls.KeyControl();
|
|
ctrlKeyboardn.Setup()
|
|
.At(this, 33)
|
|
.WithParent(parent)
|
|
.WithName("n")
|
|
.WithDisplayName("N")
|
|
.WithLayout(kKeyLayout)
|
|
.IsButton(true)
|
|
.WithStateBlock(new InputStateBlock
|
|
{
|
|
format = new FourCC(1112101920),
|
|
byteOffset = 0,
|
|
bitOffset = 28,
|
|
sizeInBits = 1
|
|
})
|
|
.WithMinAndMax(0, 1)
|
|
.Finish();
|
|
ctrlKeyboardn.keyCode = UnityEngine.InputSystem.Key.N;
|
|
return ctrlKeyboardn;
|
|
}
|
|
|
|
private UnityEngine.InputSystem.Controls.KeyControl Initialize_ctrlKeyboardo(InternedString kKeyLayout, InputControl parent)
|
|
{
|
|
var ctrlKeyboardo = new UnityEngine.InputSystem.Controls.KeyControl();
|
|
ctrlKeyboardo.Setup()
|
|
.At(this, 34)
|
|
.WithParent(parent)
|
|
.WithName("o")
|
|
.WithDisplayName("O")
|
|
.WithLayout(kKeyLayout)
|
|
.IsButton(true)
|
|
.WithStateBlock(new InputStateBlock
|
|
{
|
|
format = new FourCC(1112101920),
|
|
byteOffset = 0,
|
|
bitOffset = 29,
|
|
sizeInBits = 1
|
|
})
|
|
.WithMinAndMax(0, 1)
|
|
.Finish();
|
|
ctrlKeyboardo.keyCode = UnityEngine.InputSystem.Key.O;
|
|
return ctrlKeyboardo;
|
|
}
|
|
|
|
private UnityEngine.InputSystem.Controls.KeyControl Initialize_ctrlKeyboardp(InternedString kKeyLayout, InputControl parent)
|
|
{
|
|
var ctrlKeyboardp = new UnityEngine.InputSystem.Controls.KeyControl();
|
|
ctrlKeyboardp.Setup()
|
|
.At(this, 35)
|
|
.WithParent(parent)
|
|
.WithName("p")
|
|
.WithDisplayName("P")
|
|
.WithLayout(kKeyLayout)
|
|
.IsButton(true)
|
|
.WithStateBlock(new InputStateBlock
|
|
{
|
|
format = new FourCC(1112101920),
|
|
byteOffset = 0,
|
|
bitOffset = 30,
|
|
sizeInBits = 1
|
|
})
|
|
.WithMinAndMax(0, 1)
|
|
.Finish();
|
|
ctrlKeyboardp.keyCode = UnityEngine.InputSystem.Key.P;
|
|
return ctrlKeyboardp;
|
|
}
|
|
|
|
private UnityEngine.InputSystem.Controls.KeyControl Initialize_ctrlKeyboardq(InternedString kKeyLayout, InputControl parent)
|
|
{
|
|
var ctrlKeyboardq = new UnityEngine.InputSystem.Controls.KeyControl();
|
|
ctrlKeyboardq.Setup()
|
|
.At(this, 36)
|
|
.WithParent(parent)
|
|
.WithName("q")
|
|
.WithDisplayName("Q")
|
|
.WithLayout(kKeyLayout)
|
|
.IsButton(true)
|
|
.WithStateBlock(new InputStateBlock
|
|
{
|
|
format = new FourCC(1112101920),
|
|
byteOffset = 0,
|
|
bitOffset = 31,
|
|
sizeInBits = 1
|
|
})
|
|
.WithMinAndMax(0, 1)
|
|
.Finish();
|
|
ctrlKeyboardq.keyCode = UnityEngine.InputSystem.Key.Q;
|
|
return ctrlKeyboardq;
|
|
}
|
|
|
|
private UnityEngine.InputSystem.Controls.KeyControl Initialize_ctrlKeyboardr(InternedString kKeyLayout, InputControl parent)
|
|
{
|
|
var ctrlKeyboardr = new UnityEngine.InputSystem.Controls.KeyControl();
|
|
ctrlKeyboardr.Setup()
|
|
.At(this, 37)
|
|
.WithParent(parent)
|
|
.WithName("r")
|
|
.WithDisplayName("R")
|
|
.WithLayout(kKeyLayout)
|
|
.IsButton(true)
|
|
.WithStateBlock(new InputStateBlock
|
|
{
|
|
format = new FourCC(1112101920),
|
|
byteOffset = 0,
|
|
bitOffset = 32,
|
|
sizeInBits = 1
|
|
})
|
|
.WithMinAndMax(0, 1)
|
|
.Finish();
|
|
ctrlKeyboardr.keyCode = UnityEngine.InputSystem.Key.R;
|
|
return ctrlKeyboardr;
|
|
}
|
|
|
|
private UnityEngine.InputSystem.Controls.KeyControl Initialize_ctrlKeyboards(InternedString kKeyLayout, InputControl parent)
|
|
{
|
|
var ctrlKeyboards = new UnityEngine.InputSystem.Controls.KeyControl();
|
|
ctrlKeyboards.Setup()
|
|
.At(this, 38)
|
|
.WithParent(parent)
|
|
.WithName("s")
|
|
.WithDisplayName("S")
|
|
.WithLayout(kKeyLayout)
|
|
.IsButton(true)
|
|
.WithStateBlock(new InputStateBlock
|
|
{
|
|
format = new FourCC(1112101920),
|
|
byteOffset = 0,
|
|
bitOffset = 33,
|
|
sizeInBits = 1
|
|
})
|
|
.WithMinAndMax(0, 1)
|
|
.Finish();
|
|
ctrlKeyboards.keyCode = UnityEngine.InputSystem.Key.S;
|
|
return ctrlKeyboards;
|
|
}
|
|
|
|
private UnityEngine.InputSystem.Controls.KeyControl Initialize_ctrlKeyboardt(InternedString kKeyLayout, InputControl parent)
|
|
{
|
|
var ctrlKeyboardt = new UnityEngine.InputSystem.Controls.KeyControl();
|
|
ctrlKeyboardt.Setup()
|
|
.At(this, 39)
|
|
.WithParent(parent)
|
|
.WithName("t")
|
|
.WithDisplayName("T")
|
|
.WithLayout(kKeyLayout)
|
|
.IsButton(true)
|
|
.WithStateBlock(new InputStateBlock
|
|
{
|
|
format = new FourCC(1112101920),
|
|
byteOffset = 0,
|
|
bitOffset = 34,
|
|
sizeInBits = 1
|
|
})
|
|
.WithMinAndMax(0, 1)
|
|
.Finish();
|
|
ctrlKeyboardt.keyCode = UnityEngine.InputSystem.Key.T;
|
|
return ctrlKeyboardt;
|
|
}
|
|
|
|
private UnityEngine.InputSystem.Controls.KeyControl Initialize_ctrlKeyboardu(InternedString kKeyLayout, InputControl parent)
|
|
{
|
|
var ctrlKeyboardu = new UnityEngine.InputSystem.Controls.KeyControl();
|
|
ctrlKeyboardu.Setup()
|
|
.At(this, 40)
|
|
.WithParent(parent)
|
|
.WithName("u")
|
|
.WithDisplayName("U")
|
|
.WithLayout(kKeyLayout)
|
|
.IsButton(true)
|
|
.WithStateBlock(new InputStateBlock
|
|
{
|
|
format = new FourCC(1112101920),
|
|
byteOffset = 0,
|
|
bitOffset = 35,
|
|
sizeInBits = 1
|
|
})
|
|
.WithMinAndMax(0, 1)
|
|
.Finish();
|
|
ctrlKeyboardu.keyCode = UnityEngine.InputSystem.Key.U;
|
|
return ctrlKeyboardu;
|
|
}
|
|
|
|
private UnityEngine.InputSystem.Controls.KeyControl Initialize_ctrlKeyboardv(InternedString kKeyLayout, InputControl parent)
|
|
{
|
|
var ctrlKeyboardv = new UnityEngine.InputSystem.Controls.KeyControl();
|
|
ctrlKeyboardv.Setup()
|
|
.At(this, 41)
|
|
.WithParent(parent)
|
|
.WithName("v")
|
|
.WithDisplayName("V")
|
|
.WithLayout(kKeyLayout)
|
|
.IsButton(true)
|
|
.WithStateBlock(new InputStateBlock
|
|
{
|
|
format = new FourCC(1112101920),
|
|
byteOffset = 0,
|
|
bitOffset = 36,
|
|
sizeInBits = 1
|
|
})
|
|
.WithMinAndMax(0, 1)
|
|
.Finish();
|
|
ctrlKeyboardv.keyCode = UnityEngine.InputSystem.Key.V;
|
|
return ctrlKeyboardv;
|
|
}
|
|
|
|
private UnityEngine.InputSystem.Controls.KeyControl Initialize_ctrlKeyboardw(InternedString kKeyLayout, InputControl parent)
|
|
{
|
|
var ctrlKeyboardw = new UnityEngine.InputSystem.Controls.KeyControl();
|
|
ctrlKeyboardw.Setup()
|
|
.At(this, 42)
|
|
.WithParent(parent)
|
|
.WithName("w")
|
|
.WithDisplayName("W")
|
|
.WithLayout(kKeyLayout)
|
|
.IsButton(true)
|
|
.WithStateBlock(new InputStateBlock
|
|
{
|
|
format = new FourCC(1112101920),
|
|
byteOffset = 0,
|
|
bitOffset = 37,
|
|
sizeInBits = 1
|
|
})
|
|
.WithMinAndMax(0, 1)
|
|
.Finish();
|
|
ctrlKeyboardw.keyCode = UnityEngine.InputSystem.Key.W;
|
|
return ctrlKeyboardw;
|
|
}
|
|
|
|
private UnityEngine.InputSystem.Controls.KeyControl Initialize_ctrlKeyboardx(InternedString kKeyLayout, InputControl parent)
|
|
{
|
|
var ctrlKeyboardx = new UnityEngine.InputSystem.Controls.KeyControl();
|
|
ctrlKeyboardx.Setup()
|
|
.At(this, 43)
|
|
.WithParent(parent)
|
|
.WithName("x")
|
|
.WithDisplayName("X")
|
|
.WithLayout(kKeyLayout)
|
|
.IsButton(true)
|
|
.WithStateBlock(new InputStateBlock
|
|
{
|
|
format = new FourCC(1112101920),
|
|
byteOffset = 0,
|
|
bitOffset = 38,
|
|
sizeInBits = 1
|
|
})
|
|
.WithMinAndMax(0, 1)
|
|
.Finish();
|
|
ctrlKeyboardx.keyCode = UnityEngine.InputSystem.Key.X;
|
|
return ctrlKeyboardx;
|
|
}
|
|
|
|
private UnityEngine.InputSystem.Controls.KeyControl Initialize_ctrlKeyboardy(InternedString kKeyLayout, InputControl parent)
|
|
{
|
|
var ctrlKeyboardy = new UnityEngine.InputSystem.Controls.KeyControl();
|
|
ctrlKeyboardy.Setup()
|
|
.At(this, 44)
|
|
.WithParent(parent)
|
|
.WithName("y")
|
|
.WithDisplayName("Y")
|
|
.WithLayout(kKeyLayout)
|
|
.IsButton(true)
|
|
.WithStateBlock(new InputStateBlock
|
|
{
|
|
format = new FourCC(1112101920),
|
|
byteOffset = 0,
|
|
bitOffset = 39,
|
|
sizeInBits = 1
|
|
})
|
|
.WithMinAndMax(0, 1)
|
|
.Finish();
|
|
ctrlKeyboardy.keyCode = UnityEngine.InputSystem.Key.Y;
|
|
return ctrlKeyboardy;
|
|
}
|
|
|
|
private UnityEngine.InputSystem.Controls.KeyControl Initialize_ctrlKeyboardz(InternedString kKeyLayout, InputControl parent)
|
|
{
|
|
var ctrlKeyboardz = new UnityEngine.InputSystem.Controls.KeyControl();
|
|
ctrlKeyboardz.Setup()
|
|
.At(this, 45)
|
|
.WithParent(parent)
|
|
.WithName("z")
|
|
.WithDisplayName("Z")
|
|
.WithLayout(kKeyLayout)
|
|
.IsButton(true)
|
|
.WithStateBlock(new InputStateBlock
|
|
{
|
|
format = new FourCC(1112101920),
|
|
byteOffset = 0,
|
|
bitOffset = 40,
|
|
sizeInBits = 1
|
|
})
|
|
.WithMinAndMax(0, 1)
|
|
.Finish();
|
|
ctrlKeyboardz.keyCode = UnityEngine.InputSystem.Key.Z;
|
|
return ctrlKeyboardz;
|
|
}
|
|
|
|
private UnityEngine.InputSystem.Controls.KeyControl Initialize_ctrlKeyboard1(InternedString kKeyLayout, InputControl parent)
|
|
{
|
|
var ctrlKeyboard1 = new UnityEngine.InputSystem.Controls.KeyControl();
|
|
ctrlKeyboard1.Setup()
|
|
.At(this, 46)
|
|
.WithParent(parent)
|
|
.WithName("1")
|
|
.WithDisplayName("1")
|
|
.WithLayout(kKeyLayout)
|
|
.IsButton(true)
|
|
.WithStateBlock(new InputStateBlock
|
|
{
|
|
format = new FourCC(1112101920),
|
|
byteOffset = 0,
|
|
bitOffset = 41,
|
|
sizeInBits = 1
|
|
})
|
|
.WithMinAndMax(0, 1)
|
|
.Finish();
|
|
ctrlKeyboard1.keyCode = UnityEngine.InputSystem.Key.Digit1;
|
|
return ctrlKeyboard1;
|
|
}
|
|
|
|
private UnityEngine.InputSystem.Controls.KeyControl Initialize_ctrlKeyboard2(InternedString kKeyLayout, InputControl parent)
|
|
{
|
|
var ctrlKeyboard2 = new UnityEngine.InputSystem.Controls.KeyControl();
|
|
ctrlKeyboard2.Setup()
|
|
.At(this, 47)
|
|
.WithParent(parent)
|
|
.WithName("2")
|
|
.WithDisplayName("2")
|
|
.WithLayout(kKeyLayout)
|
|
.IsButton(true)
|
|
.WithStateBlock(new InputStateBlock
|
|
{
|
|
format = new FourCC(1112101920),
|
|
byteOffset = 0,
|
|
bitOffset = 42,
|
|
sizeInBits = 1
|
|
})
|
|
.WithMinAndMax(0, 1)
|
|
.Finish();
|
|
ctrlKeyboard2.keyCode = UnityEngine.InputSystem.Key.Digit2;
|
|
return ctrlKeyboard2;
|
|
}
|
|
|
|
private UnityEngine.InputSystem.Controls.KeyControl Initialize_ctrlKeyboard3(InternedString kKeyLayout, InputControl parent)
|
|
{
|
|
var ctrlKeyboard3 = new UnityEngine.InputSystem.Controls.KeyControl();
|
|
ctrlKeyboard3.Setup()
|
|
.At(this, 48)
|
|
.WithParent(parent)
|
|
.WithName("3")
|
|
.WithDisplayName("3")
|
|
.WithLayout(kKeyLayout)
|
|
.IsButton(true)
|
|
.WithStateBlock(new InputStateBlock
|
|
{
|
|
format = new FourCC(1112101920),
|
|
byteOffset = 0,
|
|
bitOffset = 43,
|
|
sizeInBits = 1
|
|
})
|
|
.WithMinAndMax(0, 1)
|
|
.Finish();
|
|
ctrlKeyboard3.keyCode = UnityEngine.InputSystem.Key.Digit3;
|
|
return ctrlKeyboard3;
|
|
}
|
|
|
|
private UnityEngine.InputSystem.Controls.KeyControl Initialize_ctrlKeyboard4(InternedString kKeyLayout, InputControl parent)
|
|
{
|
|
var ctrlKeyboard4 = new UnityEngine.InputSystem.Controls.KeyControl();
|
|
ctrlKeyboard4.Setup()
|
|
.At(this, 49)
|
|
.WithParent(parent)
|
|
.WithName("4")
|
|
.WithDisplayName("4")
|
|
.WithLayout(kKeyLayout)
|
|
.IsButton(true)
|
|
.WithStateBlock(new InputStateBlock
|
|
{
|
|
format = new FourCC(1112101920),
|
|
byteOffset = 0,
|
|
bitOffset = 44,
|
|
sizeInBits = 1
|
|
})
|
|
.WithMinAndMax(0, 1)
|
|
.Finish();
|
|
ctrlKeyboard4.keyCode = UnityEngine.InputSystem.Key.Digit4;
|
|
return ctrlKeyboard4;
|
|
}
|
|
|
|
private UnityEngine.InputSystem.Controls.KeyControl Initialize_ctrlKeyboard5(InternedString kKeyLayout, InputControl parent)
|
|
{
|
|
var ctrlKeyboard5 = new UnityEngine.InputSystem.Controls.KeyControl();
|
|
ctrlKeyboard5.Setup()
|
|
.At(this, 50)
|
|
.WithParent(parent)
|
|
.WithName("5")
|
|
.WithDisplayName("5")
|
|
.WithLayout(kKeyLayout)
|
|
.IsButton(true)
|
|
.WithStateBlock(new InputStateBlock
|
|
{
|
|
format = new FourCC(1112101920),
|
|
byteOffset = 0,
|
|
bitOffset = 45,
|
|
sizeInBits = 1
|
|
})
|
|
.WithMinAndMax(0, 1)
|
|
.Finish();
|
|
ctrlKeyboard5.keyCode = UnityEngine.InputSystem.Key.Digit5;
|
|
return ctrlKeyboard5;
|
|
}
|
|
|
|
private UnityEngine.InputSystem.Controls.KeyControl Initialize_ctrlKeyboard6(InternedString kKeyLayout, InputControl parent)
|
|
{
|
|
var ctrlKeyboard6 = new UnityEngine.InputSystem.Controls.KeyControl();
|
|
ctrlKeyboard6.Setup()
|
|
.At(this, 51)
|
|
.WithParent(parent)
|
|
.WithName("6")
|
|
.WithDisplayName("6")
|
|
.WithLayout(kKeyLayout)
|
|
.IsButton(true)
|
|
.WithStateBlock(new InputStateBlock
|
|
{
|
|
format = new FourCC(1112101920),
|
|
byteOffset = 0,
|
|
bitOffset = 46,
|
|
sizeInBits = 1
|
|
})
|
|
.WithMinAndMax(0, 1)
|
|
.Finish();
|
|
ctrlKeyboard6.keyCode = UnityEngine.InputSystem.Key.Digit6;
|
|
return ctrlKeyboard6;
|
|
}
|
|
|
|
private UnityEngine.InputSystem.Controls.KeyControl Initialize_ctrlKeyboard7(InternedString kKeyLayout, InputControl parent)
|
|
{
|
|
var ctrlKeyboard7 = new UnityEngine.InputSystem.Controls.KeyControl();
|
|
ctrlKeyboard7.Setup()
|
|
.At(this, 52)
|
|
.WithParent(parent)
|
|
.WithName("7")
|
|
.WithDisplayName("7")
|
|
.WithLayout(kKeyLayout)
|
|
.IsButton(true)
|
|
.WithStateBlock(new InputStateBlock
|
|
{
|
|
format = new FourCC(1112101920),
|
|
byteOffset = 0,
|
|
bitOffset = 47,
|
|
sizeInBits = 1
|
|
})
|
|
.WithMinAndMax(0, 1)
|
|
.Finish();
|
|
ctrlKeyboard7.keyCode = UnityEngine.InputSystem.Key.Digit7;
|
|
return ctrlKeyboard7;
|
|
}
|
|
|
|
private UnityEngine.InputSystem.Controls.KeyControl Initialize_ctrlKeyboard8(InternedString kKeyLayout, InputControl parent)
|
|
{
|
|
var ctrlKeyboard8 = new UnityEngine.InputSystem.Controls.KeyControl();
|
|
ctrlKeyboard8.Setup()
|
|
.At(this, 53)
|
|
.WithParent(parent)
|
|
.WithName("8")
|
|
.WithDisplayName("8")
|
|
.WithLayout(kKeyLayout)
|
|
.IsButton(true)
|
|
.WithStateBlock(new InputStateBlock
|
|
{
|
|
format = new FourCC(1112101920),
|
|
byteOffset = 0,
|
|
bitOffset = 48,
|
|
sizeInBits = 1
|
|
})
|
|
.WithMinAndMax(0, 1)
|
|
.Finish();
|
|
ctrlKeyboard8.keyCode = UnityEngine.InputSystem.Key.Digit8;
|
|
return ctrlKeyboard8;
|
|
}
|
|
|
|
private UnityEngine.InputSystem.Controls.KeyControl Initialize_ctrlKeyboard9(InternedString kKeyLayout, InputControl parent)
|
|
{
|
|
var ctrlKeyboard9 = new UnityEngine.InputSystem.Controls.KeyControl();
|
|
ctrlKeyboard9.Setup()
|
|
.At(this, 54)
|
|
.WithParent(parent)
|
|
.WithName("9")
|
|
.WithDisplayName("9")
|
|
.WithLayout(kKeyLayout)
|
|
.IsButton(true)
|
|
.WithStateBlock(new InputStateBlock
|
|
{
|
|
format = new FourCC(1112101920),
|
|
byteOffset = 0,
|
|
bitOffset = 49,
|
|
sizeInBits = 1
|
|
})
|
|
.WithMinAndMax(0, 1)
|
|
.Finish();
|
|
ctrlKeyboard9.keyCode = UnityEngine.InputSystem.Key.Digit9;
|
|
return ctrlKeyboard9;
|
|
}
|
|
|
|
private UnityEngine.InputSystem.Controls.KeyControl Initialize_ctrlKeyboard0(InternedString kKeyLayout, InputControl parent)
|
|
{
|
|
var ctrlKeyboard0 = new UnityEngine.InputSystem.Controls.KeyControl();
|
|
ctrlKeyboard0.Setup()
|
|
.At(this, 55)
|
|
.WithParent(parent)
|
|
.WithName("0")
|
|
.WithDisplayName("0")
|
|
.WithLayout(kKeyLayout)
|
|
.IsButton(true)
|
|
.WithStateBlock(new InputStateBlock
|
|
{
|
|
format = new FourCC(1112101920),
|
|
byteOffset = 0,
|
|
bitOffset = 50,
|
|
sizeInBits = 1
|
|
})
|
|
.WithMinAndMax(0, 1)
|
|
.Finish();
|
|
ctrlKeyboard0.keyCode = UnityEngine.InputSystem.Key.Digit0;
|
|
return ctrlKeyboard0;
|
|
}
|
|
|
|
private UnityEngine.InputSystem.Controls.KeyControl Initialize_ctrlKeyboardleftShift(InternedString kKeyLayout, InputControl parent)
|
|
{
|
|
var ctrlKeyboardleftShift = new UnityEngine.InputSystem.Controls.KeyControl();
|
|
ctrlKeyboardleftShift.Setup()
|
|
.At(this, 56)
|
|
.WithParent(parent)
|
|
.WithName("leftShift")
|
|
.WithDisplayName("Left Shift")
|
|
.WithLayout(kKeyLayout)
|
|
.WithUsages(3, 1)
|
|
.IsButton(true)
|
|
.WithStateBlock(new InputStateBlock
|
|
{
|
|
format = new FourCC(1112101920),
|
|
byteOffset = 0,
|
|
bitOffset = 51,
|
|
sizeInBits = 1
|
|
})
|
|
.WithMinAndMax(0, 1)
|
|
.Finish();
|
|
ctrlKeyboardleftShift.keyCode = UnityEngine.InputSystem.Key.LeftShift;
|
|
return ctrlKeyboardleftShift;
|
|
}
|
|
|
|
private UnityEngine.InputSystem.Controls.KeyControl Initialize_ctrlKeyboardrightShift(InternedString kKeyLayout, InputControl parent)
|
|
{
|
|
var ctrlKeyboardrightShift = new UnityEngine.InputSystem.Controls.KeyControl();
|
|
ctrlKeyboardrightShift.Setup()
|
|
.At(this, 57)
|
|
.WithParent(parent)
|
|
.WithName("rightShift")
|
|
.WithDisplayName("Right Shift")
|
|
.WithLayout(kKeyLayout)
|
|
.WithUsages(4, 1)
|
|
.IsButton(true)
|
|
.WithStateBlock(new InputStateBlock
|
|
{
|
|
format = new FourCC(1112101920),
|
|
byteOffset = 0,
|
|
bitOffset = 52,
|
|
sizeInBits = 1
|
|
})
|
|
.WithMinAndMax(0, 1)
|
|
.Finish();
|
|
ctrlKeyboardrightShift.keyCode = UnityEngine.InputSystem.Key.RightShift;
|
|
return ctrlKeyboardrightShift;
|
|
}
|
|
|
|
private UnityEngine.InputSystem.Controls.DiscreteButtonControl Initialize_ctrlKeyboardshift(InternedString kDiscreteButtonLayout, InputControl parent)
|
|
{
|
|
var ctrlKeyboardshift = new UnityEngine.InputSystem.Controls.DiscreteButtonControl { minValue = 1, maxValue = 3, writeMode = UnityEngine.InputSystem.Controls.DiscreteButtonControl.WriteMode.WriteNullAndMaxValue };
|
|
ctrlKeyboardshift.Setup()
|
|
.At(this, 58)
|
|
.WithParent(parent)
|
|
.WithName("shift")
|
|
.WithDisplayName("Shift")
|
|
.WithLayout(kDiscreteButtonLayout)
|
|
.WithUsages(5, 1)
|
|
.IsSynthetic(true)
|
|
.IsButton(true)
|
|
.WithStateBlock(new InputStateBlock
|
|
{
|
|
format = new FourCC(1112101920),
|
|
byteOffset = 0,
|
|
bitOffset = 51,
|
|
sizeInBits = 2
|
|
})
|
|
.WithMinAndMax(0, 1)
|
|
.Finish();
|
|
return ctrlKeyboardshift;
|
|
}
|
|
|
|
private UnityEngine.InputSystem.Controls.KeyControl Initialize_ctrlKeyboardleftAlt(InternedString kKeyLayout, InputControl parent)
|
|
{
|
|
var ctrlKeyboardleftAlt = new UnityEngine.InputSystem.Controls.KeyControl();
|
|
ctrlKeyboardleftAlt.Setup()
|
|
.At(this, 59)
|
|
.WithParent(parent)
|
|
.WithName("leftAlt")
|
|
.WithDisplayName("Left Alt")
|
|
.WithLayout(kKeyLayout)
|
|
.WithUsages(6, 1)
|
|
.IsButton(true)
|
|
.WithStateBlock(new InputStateBlock
|
|
{
|
|
format = new FourCC(1112101920),
|
|
byteOffset = 0,
|
|
bitOffset = 53,
|
|
sizeInBits = 1
|
|
})
|
|
.WithMinAndMax(0, 1)
|
|
.Finish();
|
|
ctrlKeyboardleftAlt.keyCode = UnityEngine.InputSystem.Key.LeftAlt;
|
|
return ctrlKeyboardleftAlt;
|
|
}
|
|
|
|
private UnityEngine.InputSystem.Controls.KeyControl Initialize_ctrlKeyboardrightAlt(InternedString kKeyLayout, InputControl parent)
|
|
{
|
|
var ctrlKeyboardrightAlt = new UnityEngine.InputSystem.Controls.KeyControl();
|
|
ctrlKeyboardrightAlt.Setup()
|
|
.At(this, 60)
|
|
.WithParent(parent)
|
|
.WithName("rightAlt")
|
|
.WithDisplayName("Right Alt")
|
|
.WithLayout(kKeyLayout)
|
|
.WithUsages(7, 1)
|
|
.WithAliases(0, 1)
|
|
.IsButton(true)
|
|
.WithStateBlock(new InputStateBlock
|
|
{
|
|
format = new FourCC(1112101920),
|
|
byteOffset = 0,
|
|
bitOffset = 54,
|
|
sizeInBits = 1
|
|
})
|
|
.WithMinAndMax(0, 1)
|
|
.Finish();
|
|
ctrlKeyboardrightAlt.keyCode = UnityEngine.InputSystem.Key.AltGr;
|
|
return ctrlKeyboardrightAlt;
|
|
}
|
|
|
|
private UnityEngine.InputSystem.Controls.DiscreteButtonControl Initialize_ctrlKeyboardalt(InternedString kDiscreteButtonLayout, InputControl parent)
|
|
{
|
|
var ctrlKeyboardalt = new UnityEngine.InputSystem.Controls.DiscreteButtonControl { minValue = 1, maxValue = 3, writeMode = UnityEngine.InputSystem.Controls.DiscreteButtonControl.WriteMode.WriteNullAndMaxValue };
|
|
ctrlKeyboardalt.Setup()
|
|
.At(this, 61)
|
|
.WithParent(parent)
|
|
.WithName("alt")
|
|
.WithDisplayName("Alt")
|
|
.WithLayout(kDiscreteButtonLayout)
|
|
.WithUsages(8, 1)
|
|
.IsSynthetic(true)
|
|
.IsButton(true)
|
|
.WithStateBlock(new InputStateBlock
|
|
{
|
|
format = new FourCC(1112101920),
|
|
byteOffset = 0,
|
|
bitOffset = 53,
|
|
sizeInBits = 2
|
|
})
|
|
.WithMinAndMax(0, 1)
|
|
.Finish();
|
|
return ctrlKeyboardalt;
|
|
}
|
|
|
|
private UnityEngine.InputSystem.Controls.KeyControl Initialize_ctrlKeyboardleftCtrl(InternedString kKeyLayout, InputControl parent)
|
|
{
|
|
var ctrlKeyboardleftCtrl = new UnityEngine.InputSystem.Controls.KeyControl();
|
|
ctrlKeyboardleftCtrl.Setup()
|
|
.At(this, 62)
|
|
.WithParent(parent)
|
|
.WithName("leftCtrl")
|
|
.WithDisplayName("Left Control")
|
|
.WithLayout(kKeyLayout)
|
|
.WithUsages(9, 1)
|
|
.IsButton(true)
|
|
.WithStateBlock(new InputStateBlock
|
|
{
|
|
format = new FourCC(1112101920),
|
|
byteOffset = 0,
|
|
bitOffset = 55,
|
|
sizeInBits = 1
|
|
})
|
|
.WithMinAndMax(0, 1)
|
|
.Finish();
|
|
ctrlKeyboardleftCtrl.keyCode = UnityEngine.InputSystem.Key.LeftCtrl;
|
|
return ctrlKeyboardleftCtrl;
|
|
}
|
|
|
|
private UnityEngine.InputSystem.Controls.KeyControl Initialize_ctrlKeyboardrightCtrl(InternedString kKeyLayout, InputControl parent)
|
|
{
|
|
var ctrlKeyboardrightCtrl = new UnityEngine.InputSystem.Controls.KeyControl();
|
|
ctrlKeyboardrightCtrl.Setup()
|
|
.At(this, 63)
|
|
.WithParent(parent)
|
|
.WithName("rightCtrl")
|
|
.WithDisplayName("Right Control")
|
|
.WithLayout(kKeyLayout)
|
|
.WithUsages(10, 1)
|
|
.IsButton(true)
|
|
.WithStateBlock(new InputStateBlock
|
|
{
|
|
format = new FourCC(1112101920),
|
|
byteOffset = 0,
|
|
bitOffset = 56,
|
|
sizeInBits = 1
|
|
})
|
|
.WithMinAndMax(0, 1)
|
|
.Finish();
|
|
ctrlKeyboardrightCtrl.keyCode = UnityEngine.InputSystem.Key.RightCtrl;
|
|
return ctrlKeyboardrightCtrl;
|
|
}
|
|
|
|
private UnityEngine.InputSystem.Controls.DiscreteButtonControl Initialize_ctrlKeyboardctrl(InternedString kDiscreteButtonLayout, InputControl parent)
|
|
{
|
|
var ctrlKeyboardctrl = new UnityEngine.InputSystem.Controls.DiscreteButtonControl { minValue = 1, maxValue = 3, writeMode = UnityEngine.InputSystem.Controls.DiscreteButtonControl.WriteMode.WriteNullAndMaxValue };
|
|
ctrlKeyboardctrl.Setup()
|
|
.At(this, 64)
|
|
.WithParent(parent)
|
|
.WithName("ctrl")
|
|
.WithDisplayName("Control")
|
|
.WithLayout(kDiscreteButtonLayout)
|
|
.WithUsages(11, 1)
|
|
.IsSynthetic(true)
|
|
.IsButton(true)
|
|
.WithStateBlock(new InputStateBlock
|
|
{
|
|
format = new FourCC(1112101920),
|
|
byteOffset = 0,
|
|
bitOffset = 55,
|
|
sizeInBits = 2
|
|
})
|
|
.WithMinAndMax(0, 1)
|
|
.Finish();
|
|
return ctrlKeyboardctrl;
|
|
}
|
|
|
|
private UnityEngine.InputSystem.Controls.KeyControl Initialize_ctrlKeyboardleftMeta(InternedString kKeyLayout, InputControl parent)
|
|
{
|
|
var ctrlKeyboardleftMeta = new UnityEngine.InputSystem.Controls.KeyControl();
|
|
ctrlKeyboardleftMeta.Setup()
|
|
.At(this, 65)
|
|
.WithParent(parent)
|
|
.WithName("leftMeta")
|
|
.WithDisplayName("Left System")
|
|
.WithLayout(kKeyLayout)
|
|
.WithUsages(12, 1)
|
|
.WithAliases(1, 3)
|
|
.IsButton(true)
|
|
.WithStateBlock(new InputStateBlock
|
|
{
|
|
format = new FourCC(1112101920),
|
|
byteOffset = 0,
|
|
bitOffset = 57,
|
|
sizeInBits = 1
|
|
})
|
|
.WithMinAndMax(0, 1)
|
|
.Finish();
|
|
ctrlKeyboardleftMeta.keyCode = UnityEngine.InputSystem.Key.LeftWindows;
|
|
return ctrlKeyboardleftMeta;
|
|
}
|
|
|
|
private UnityEngine.InputSystem.Controls.KeyControl Initialize_ctrlKeyboardrightMeta(InternedString kKeyLayout, InputControl parent)
|
|
{
|
|
var ctrlKeyboardrightMeta = new UnityEngine.InputSystem.Controls.KeyControl();
|
|
ctrlKeyboardrightMeta.Setup()
|
|
.At(this, 66)
|
|
.WithParent(parent)
|
|
.WithName("rightMeta")
|
|
.WithDisplayName("Right System")
|
|
.WithLayout(kKeyLayout)
|
|
.WithUsages(13, 1)
|
|
.WithAliases(4, 3)
|
|
.IsButton(true)
|
|
.WithStateBlock(new InputStateBlock
|
|
{
|
|
format = new FourCC(1112101920),
|
|
byteOffset = 0,
|
|
bitOffset = 58,
|
|
sizeInBits = 1
|
|
})
|
|
.WithMinAndMax(0, 1)
|
|
.Finish();
|
|
ctrlKeyboardrightMeta.keyCode = UnityEngine.InputSystem.Key.RightCommand;
|
|
return ctrlKeyboardrightMeta;
|
|
}
|
|
|
|
private UnityEngine.InputSystem.Controls.KeyControl Initialize_ctrlKeyboardcontextMenu(InternedString kKeyLayout, InputControl parent)
|
|
{
|
|
var ctrlKeyboardcontextMenu = new UnityEngine.InputSystem.Controls.KeyControl();
|
|
ctrlKeyboardcontextMenu.Setup()
|
|
.At(this, 67)
|
|
.WithParent(parent)
|
|
.WithName("contextMenu")
|
|
.WithDisplayName("Context Menu")
|
|
.WithLayout(kKeyLayout)
|
|
.WithUsages(14, 1)
|
|
.IsButton(true)
|
|
.WithStateBlock(new InputStateBlock
|
|
{
|
|
format = new FourCC(1112101920),
|
|
byteOffset = 0,
|
|
bitOffset = 59,
|
|
sizeInBits = 1
|
|
})
|
|
.WithMinAndMax(0, 1)
|
|
.Finish();
|
|
ctrlKeyboardcontextMenu.keyCode = UnityEngine.InputSystem.Key.ContextMenu;
|
|
return ctrlKeyboardcontextMenu;
|
|
}
|
|
|
|
private UnityEngine.InputSystem.Controls.KeyControl Initialize_ctrlKeyboardbackspace(InternedString kKeyLayout, InputControl parent)
|
|
{
|
|
var ctrlKeyboardbackspace = new UnityEngine.InputSystem.Controls.KeyControl();
|
|
ctrlKeyboardbackspace.Setup()
|
|
.At(this, 68)
|
|
.WithParent(parent)
|
|
.WithName("backspace")
|
|
.WithDisplayName("Backspace")
|
|
.WithLayout(kKeyLayout)
|
|
.IsButton(true)
|
|
.WithStateBlock(new InputStateBlock
|
|
{
|
|
format = new FourCC(1112101920),
|
|
byteOffset = 0,
|
|
bitOffset = 65,
|
|
sizeInBits = 1
|
|
})
|
|
.WithMinAndMax(0, 1)
|
|
.Finish();
|
|
ctrlKeyboardbackspace.keyCode = UnityEngine.InputSystem.Key.Backspace;
|
|
return ctrlKeyboardbackspace;
|
|
}
|
|
|
|
private UnityEngine.InputSystem.Controls.KeyControl Initialize_ctrlKeyboardpageDown(InternedString kKeyLayout, InputControl parent)
|
|
{
|
|
var ctrlKeyboardpageDown = new UnityEngine.InputSystem.Controls.KeyControl();
|
|
ctrlKeyboardpageDown.Setup()
|
|
.At(this, 69)
|
|
.WithParent(parent)
|
|
.WithName("pageDown")
|
|
.WithDisplayName("Page Down")
|
|
.WithLayout(kKeyLayout)
|
|
.IsButton(true)
|
|
.WithStateBlock(new InputStateBlock
|
|
{
|
|
format = new FourCC(1112101920),
|
|
byteOffset = 0,
|
|
bitOffset = 66,
|
|
sizeInBits = 1
|
|
})
|
|
.WithMinAndMax(0, 1)
|
|
.Finish();
|
|
ctrlKeyboardpageDown.keyCode = UnityEngine.InputSystem.Key.PageDown;
|
|
return ctrlKeyboardpageDown;
|
|
}
|
|
|
|
private UnityEngine.InputSystem.Controls.KeyControl Initialize_ctrlKeyboardpageUp(InternedString kKeyLayout, InputControl parent)
|
|
{
|
|
var ctrlKeyboardpageUp = new UnityEngine.InputSystem.Controls.KeyControl();
|
|
ctrlKeyboardpageUp.Setup()
|
|
.At(this, 70)
|
|
.WithParent(parent)
|
|
.WithName("pageUp")
|
|
.WithDisplayName("Page Up")
|
|
.WithLayout(kKeyLayout)
|
|
.IsButton(true)
|
|
.WithStateBlock(new InputStateBlock
|
|
{
|
|
format = new FourCC(1112101920),
|
|
byteOffset = 0,
|
|
bitOffset = 67,
|
|
sizeInBits = 1
|
|
})
|
|
.WithMinAndMax(0, 1)
|
|
.Finish();
|
|
ctrlKeyboardpageUp.keyCode = UnityEngine.InputSystem.Key.PageUp;
|
|
return ctrlKeyboardpageUp;
|
|
}
|
|
|
|
private UnityEngine.InputSystem.Controls.KeyControl Initialize_ctrlKeyboardhome(InternedString kKeyLayout, InputControl parent)
|
|
{
|
|
var ctrlKeyboardhome = new UnityEngine.InputSystem.Controls.KeyControl();
|
|
ctrlKeyboardhome.Setup()
|
|
.At(this, 71)
|
|
.WithParent(parent)
|
|
.WithName("home")
|
|
.WithDisplayName("Home")
|
|
.WithLayout(kKeyLayout)
|
|
.IsButton(true)
|
|
.WithStateBlock(new InputStateBlock
|
|
{
|
|
format = new FourCC(1112101920),
|
|
byteOffset = 0,
|
|
bitOffset = 68,
|
|
sizeInBits = 1
|
|
})
|
|
.WithMinAndMax(0, 1)
|
|
.Finish();
|
|
ctrlKeyboardhome.keyCode = UnityEngine.InputSystem.Key.Home;
|
|
return ctrlKeyboardhome;
|
|
}
|
|
|
|
private UnityEngine.InputSystem.Controls.KeyControl Initialize_ctrlKeyboardend(InternedString kKeyLayout, InputControl parent)
|
|
{
|
|
var ctrlKeyboardend = new UnityEngine.InputSystem.Controls.KeyControl();
|
|
ctrlKeyboardend.Setup()
|
|
.At(this, 72)
|
|
.WithParent(parent)
|
|
.WithName("end")
|
|
.WithDisplayName("End")
|
|
.WithLayout(kKeyLayout)
|
|
.IsButton(true)
|
|
.WithStateBlock(new InputStateBlock
|
|
{
|
|
format = new FourCC(1112101920),
|
|
byteOffset = 0,
|
|
bitOffset = 69,
|
|
sizeInBits = 1
|
|
})
|
|
.WithMinAndMax(0, 1)
|
|
.Finish();
|
|
ctrlKeyboardend.keyCode = UnityEngine.InputSystem.Key.End;
|
|
return ctrlKeyboardend;
|
|
}
|
|
|
|
private UnityEngine.InputSystem.Controls.KeyControl Initialize_ctrlKeyboardinsert(InternedString kKeyLayout, InputControl parent)
|
|
{
|
|
var ctrlKeyboardinsert = new UnityEngine.InputSystem.Controls.KeyControl();
|
|
ctrlKeyboardinsert.Setup()
|
|
.At(this, 73)
|
|
.WithParent(parent)
|
|
.WithName("insert")
|
|
.WithDisplayName("Insert")
|
|
.WithLayout(kKeyLayout)
|
|
.IsButton(true)
|
|
.WithStateBlock(new InputStateBlock
|
|
{
|
|
format = new FourCC(1112101920),
|
|
byteOffset = 0,
|
|
bitOffset = 70,
|
|
sizeInBits = 1
|
|
})
|
|
.WithMinAndMax(0, 1)
|
|
.Finish();
|
|
ctrlKeyboardinsert.keyCode = UnityEngine.InputSystem.Key.Insert;
|
|
return ctrlKeyboardinsert;
|
|
}
|
|
|
|
private UnityEngine.InputSystem.Controls.KeyControl Initialize_ctrlKeyboarddelete(InternedString kKeyLayout, InputControl parent)
|
|
{
|
|
var ctrlKeyboarddelete = new UnityEngine.InputSystem.Controls.KeyControl();
|
|
ctrlKeyboarddelete.Setup()
|
|
.At(this, 74)
|
|
.WithParent(parent)
|
|
.WithName("delete")
|
|
.WithDisplayName("Delete")
|
|
.WithLayout(kKeyLayout)
|
|
.IsButton(true)
|
|
.WithStateBlock(new InputStateBlock
|
|
{
|
|
format = new FourCC(1112101920),
|
|
byteOffset = 0,
|
|
bitOffset = 71,
|
|
sizeInBits = 1
|
|
})
|
|
.WithMinAndMax(0, 1)
|
|
.Finish();
|
|
ctrlKeyboarddelete.keyCode = UnityEngine.InputSystem.Key.Delete;
|
|
return ctrlKeyboarddelete;
|
|
}
|
|
|
|
private UnityEngine.InputSystem.Controls.KeyControl Initialize_ctrlKeyboardcapsLock(InternedString kKeyLayout, InputControl parent)
|
|
{
|
|
var ctrlKeyboardcapsLock = new UnityEngine.InputSystem.Controls.KeyControl();
|
|
ctrlKeyboardcapsLock.Setup()
|
|
.At(this, 75)
|
|
.WithParent(parent)
|
|
.WithName("capsLock")
|
|
.WithDisplayName("Caps Lock")
|
|
.WithLayout(kKeyLayout)
|
|
.IsButton(true)
|
|
.WithStateBlock(new InputStateBlock
|
|
{
|
|
format = new FourCC(1112101920),
|
|
byteOffset = 0,
|
|
bitOffset = 72,
|
|
sizeInBits = 1
|
|
})
|
|
.WithMinAndMax(0, 1)
|
|
.Finish();
|
|
ctrlKeyboardcapsLock.keyCode = UnityEngine.InputSystem.Key.CapsLock;
|
|
return ctrlKeyboardcapsLock;
|
|
}
|
|
|
|
private UnityEngine.InputSystem.Controls.KeyControl Initialize_ctrlKeyboardnumLock(InternedString kKeyLayout, InputControl parent)
|
|
{
|
|
var ctrlKeyboardnumLock = new UnityEngine.InputSystem.Controls.KeyControl();
|
|
ctrlKeyboardnumLock.Setup()
|
|
.At(this, 76)
|
|
.WithParent(parent)
|
|
.WithName("numLock")
|
|
.WithDisplayName("Num Lock")
|
|
.WithLayout(kKeyLayout)
|
|
.IsButton(true)
|
|
.WithStateBlock(new InputStateBlock
|
|
{
|
|
format = new FourCC(1112101920),
|
|
byteOffset = 0,
|
|
bitOffset = 73,
|
|
sizeInBits = 1
|
|
})
|
|
.WithMinAndMax(0, 1)
|
|
.Finish();
|
|
ctrlKeyboardnumLock.keyCode = UnityEngine.InputSystem.Key.NumLock;
|
|
return ctrlKeyboardnumLock;
|
|
}
|
|
|
|
private UnityEngine.InputSystem.Controls.KeyControl Initialize_ctrlKeyboardprintScreen(InternedString kKeyLayout, InputControl parent)
|
|
{
|
|
var ctrlKeyboardprintScreen = new UnityEngine.InputSystem.Controls.KeyControl();
|
|
ctrlKeyboardprintScreen.Setup()
|
|
.At(this, 77)
|
|
.WithParent(parent)
|
|
.WithName("printScreen")
|
|
.WithDisplayName("Print Screen")
|
|
.WithLayout(kKeyLayout)
|
|
.IsButton(true)
|
|
.WithStateBlock(new InputStateBlock
|
|
{
|
|
format = new FourCC(1112101920),
|
|
byteOffset = 0,
|
|
bitOffset = 74,
|
|
sizeInBits = 1
|
|
})
|
|
.WithMinAndMax(0, 1)
|
|
.Finish();
|
|
ctrlKeyboardprintScreen.keyCode = UnityEngine.InputSystem.Key.PrintScreen;
|
|
return ctrlKeyboardprintScreen;
|
|
}
|
|
|
|
private UnityEngine.InputSystem.Controls.KeyControl Initialize_ctrlKeyboardscrollLock(InternedString kKeyLayout, InputControl parent)
|
|
{
|
|
var ctrlKeyboardscrollLock = new UnityEngine.InputSystem.Controls.KeyControl();
|
|
ctrlKeyboardscrollLock.Setup()
|
|
.At(this, 78)
|
|
.WithParent(parent)
|
|
.WithName("scrollLock")
|
|
.WithDisplayName("Scroll Lock")
|
|
.WithLayout(kKeyLayout)
|
|
.IsButton(true)
|
|
.WithStateBlock(new InputStateBlock
|
|
{
|
|
format = new FourCC(1112101920),
|
|
byteOffset = 0,
|
|
bitOffset = 75,
|
|
sizeInBits = 1
|
|
})
|
|
.WithMinAndMax(0, 1)
|
|
.Finish();
|
|
ctrlKeyboardscrollLock.keyCode = UnityEngine.InputSystem.Key.ScrollLock;
|
|
return ctrlKeyboardscrollLock;
|
|
}
|
|
|
|
private UnityEngine.InputSystem.Controls.KeyControl Initialize_ctrlKeyboardpause(InternedString kKeyLayout, InputControl parent)
|
|
{
|
|
var ctrlKeyboardpause = new UnityEngine.InputSystem.Controls.KeyControl();
|
|
ctrlKeyboardpause.Setup()
|
|
.At(this, 79)
|
|
.WithParent(parent)
|
|
.WithName("pause")
|
|
.WithDisplayName("Pause/Break")
|
|
.WithLayout(kKeyLayout)
|
|
.IsButton(true)
|
|
.WithStateBlock(new InputStateBlock
|
|
{
|
|
format = new FourCC(1112101920),
|
|
byteOffset = 0,
|
|
bitOffset = 76,
|
|
sizeInBits = 1
|
|
})
|
|
.WithMinAndMax(0, 1)
|
|
.Finish();
|
|
ctrlKeyboardpause.keyCode = UnityEngine.InputSystem.Key.Pause;
|
|
return ctrlKeyboardpause;
|
|
}
|
|
|
|
private UnityEngine.InputSystem.Controls.KeyControl Initialize_ctrlKeyboardnumpadEnter(InternedString kKeyLayout, InputControl parent)
|
|
{
|
|
var ctrlKeyboardnumpadEnter = new UnityEngine.InputSystem.Controls.KeyControl();
|
|
ctrlKeyboardnumpadEnter.Setup()
|
|
.At(this, 80)
|
|
.WithParent(parent)
|
|
.WithName("numpadEnter")
|
|
.WithDisplayName("Numpad Enter")
|
|
.WithLayout(kKeyLayout)
|
|
.IsButton(true)
|
|
.WithStateBlock(new InputStateBlock
|
|
{
|
|
format = new FourCC(1112101920),
|
|
byteOffset = 0,
|
|
bitOffset = 77,
|
|
sizeInBits = 1
|
|
})
|
|
.WithMinAndMax(0, 1)
|
|
.Finish();
|
|
ctrlKeyboardnumpadEnter.keyCode = UnityEngine.InputSystem.Key.NumpadEnter;
|
|
return ctrlKeyboardnumpadEnter;
|
|
}
|
|
|
|
private UnityEngine.InputSystem.Controls.KeyControl Initialize_ctrlKeyboardnumpadDivide(InternedString kKeyLayout, InputControl parent)
|
|
{
|
|
var ctrlKeyboardnumpadDivide = new UnityEngine.InputSystem.Controls.KeyControl();
|
|
ctrlKeyboardnumpadDivide.Setup()
|
|
.At(this, 81)
|
|
.WithParent(parent)
|
|
.WithName("numpadDivide")
|
|
.WithDisplayName("Numpad /")
|
|
.WithLayout(kKeyLayout)
|
|
.IsButton(true)
|
|
.WithStateBlock(new InputStateBlock
|
|
{
|
|
format = new FourCC(1112101920),
|
|
byteOffset = 0,
|
|
bitOffset = 78,
|
|
sizeInBits = 1
|
|
})
|
|
.WithMinAndMax(0, 1)
|
|
.Finish();
|
|
ctrlKeyboardnumpadDivide.keyCode = UnityEngine.InputSystem.Key.NumpadDivide;
|
|
return ctrlKeyboardnumpadDivide;
|
|
}
|
|
|
|
private UnityEngine.InputSystem.Controls.KeyControl Initialize_ctrlKeyboardnumpadMultiply(InternedString kKeyLayout, InputControl parent)
|
|
{
|
|
var ctrlKeyboardnumpadMultiply = new UnityEngine.InputSystem.Controls.KeyControl();
|
|
ctrlKeyboardnumpadMultiply.Setup()
|
|
.At(this, 82)
|
|
.WithParent(parent)
|
|
.WithName("numpadMultiply")
|
|
.WithDisplayName("Numpad *")
|
|
.WithLayout(kKeyLayout)
|
|
.IsButton(true)
|
|
.WithStateBlock(new InputStateBlock
|
|
{
|
|
format = new FourCC(1112101920),
|
|
byteOffset = 0,
|
|
bitOffset = 79,
|
|
sizeInBits = 1
|
|
})
|
|
.WithMinAndMax(0, 1)
|
|
.Finish();
|
|
ctrlKeyboardnumpadMultiply.keyCode = UnityEngine.InputSystem.Key.NumpadMultiply;
|
|
return ctrlKeyboardnumpadMultiply;
|
|
}
|
|
|
|
private UnityEngine.InputSystem.Controls.KeyControl Initialize_ctrlKeyboardnumpadPlus(InternedString kKeyLayout, InputControl parent)
|
|
{
|
|
var ctrlKeyboardnumpadPlus = new UnityEngine.InputSystem.Controls.KeyControl();
|
|
ctrlKeyboardnumpadPlus.Setup()
|
|
.At(this, 83)
|
|
.WithParent(parent)
|
|
.WithName("numpadPlus")
|
|
.WithDisplayName("Numpad +")
|
|
.WithLayout(kKeyLayout)
|
|
.IsButton(true)
|
|
.WithStateBlock(new InputStateBlock
|
|
{
|
|
format = new FourCC(1112101920),
|
|
byteOffset = 0,
|
|
bitOffset = 80,
|
|
sizeInBits = 1
|
|
})
|
|
.WithMinAndMax(0, 1)
|
|
.Finish();
|
|
ctrlKeyboardnumpadPlus.keyCode = UnityEngine.InputSystem.Key.NumpadPlus;
|
|
return ctrlKeyboardnumpadPlus;
|
|
}
|
|
|
|
private UnityEngine.InputSystem.Controls.KeyControl Initialize_ctrlKeyboardnumpadMinus(InternedString kKeyLayout, InputControl parent)
|
|
{
|
|
var ctrlKeyboardnumpadMinus = new UnityEngine.InputSystem.Controls.KeyControl();
|
|
ctrlKeyboardnumpadMinus.Setup()
|
|
.At(this, 84)
|
|
.WithParent(parent)
|
|
.WithName("numpadMinus")
|
|
.WithDisplayName("Numpad -")
|
|
.WithLayout(kKeyLayout)
|
|
.IsButton(true)
|
|
.WithStateBlock(new InputStateBlock
|
|
{
|
|
format = new FourCC(1112101920),
|
|
byteOffset = 0,
|
|
bitOffset = 81,
|
|
sizeInBits = 1
|
|
})
|
|
.WithMinAndMax(0, 1)
|
|
.Finish();
|
|
ctrlKeyboardnumpadMinus.keyCode = UnityEngine.InputSystem.Key.NumpadMinus;
|
|
return ctrlKeyboardnumpadMinus;
|
|
}
|
|
|
|
private UnityEngine.InputSystem.Controls.KeyControl Initialize_ctrlKeyboardnumpadPeriod(InternedString kKeyLayout, InputControl parent)
|
|
{
|
|
var ctrlKeyboardnumpadPeriod = new UnityEngine.InputSystem.Controls.KeyControl();
|
|
ctrlKeyboardnumpadPeriod.Setup()
|
|
.At(this, 85)
|
|
.WithParent(parent)
|
|
.WithName("numpadPeriod")
|
|
.WithDisplayName("Numpad .")
|
|
.WithLayout(kKeyLayout)
|
|
.IsButton(true)
|
|
.WithStateBlock(new InputStateBlock
|
|
{
|
|
format = new FourCC(1112101920),
|
|
byteOffset = 0,
|
|
bitOffset = 82,
|
|
sizeInBits = 1
|
|
})
|
|
.WithMinAndMax(0, 1)
|
|
.Finish();
|
|
ctrlKeyboardnumpadPeriod.keyCode = UnityEngine.InputSystem.Key.NumpadPeriod;
|
|
return ctrlKeyboardnumpadPeriod;
|
|
}
|
|
|
|
private UnityEngine.InputSystem.Controls.KeyControl Initialize_ctrlKeyboardnumpadEquals(InternedString kKeyLayout, InputControl parent)
|
|
{
|
|
var ctrlKeyboardnumpadEquals = new UnityEngine.InputSystem.Controls.KeyControl();
|
|
ctrlKeyboardnumpadEquals.Setup()
|
|
.At(this, 86)
|
|
.WithParent(parent)
|
|
.WithName("numpadEquals")
|
|
.WithDisplayName("Numpad =")
|
|
.WithLayout(kKeyLayout)
|
|
.IsButton(true)
|
|
.WithStateBlock(new InputStateBlock
|
|
{
|
|
format = new FourCC(1112101920),
|
|
byteOffset = 0,
|
|
bitOffset = 83,
|
|
sizeInBits = 1
|
|
})
|
|
.WithMinAndMax(0, 1)
|
|
.Finish();
|
|
ctrlKeyboardnumpadEquals.keyCode = UnityEngine.InputSystem.Key.NumpadEquals;
|
|
return ctrlKeyboardnumpadEquals;
|
|
}
|
|
|
|
private UnityEngine.InputSystem.Controls.KeyControl Initialize_ctrlKeyboardnumpad1(InternedString kKeyLayout, InputControl parent)
|
|
{
|
|
var ctrlKeyboardnumpad1 = new UnityEngine.InputSystem.Controls.KeyControl();
|
|
ctrlKeyboardnumpad1.Setup()
|
|
.At(this, 87)
|
|
.WithParent(parent)
|
|
.WithName("numpad1")
|
|
.WithDisplayName("Numpad 1")
|
|
.WithLayout(kKeyLayout)
|
|
.IsButton(true)
|
|
.WithStateBlock(new InputStateBlock
|
|
{
|
|
format = new FourCC(1112101920),
|
|
byteOffset = 0,
|
|
bitOffset = 85,
|
|
sizeInBits = 1
|
|
})
|
|
.WithMinAndMax(0, 1)
|
|
.Finish();
|
|
ctrlKeyboardnumpad1.keyCode = UnityEngine.InputSystem.Key.Numpad1;
|
|
return ctrlKeyboardnumpad1;
|
|
}
|
|
|
|
private UnityEngine.InputSystem.Controls.KeyControl Initialize_ctrlKeyboardnumpad2(InternedString kKeyLayout, InputControl parent)
|
|
{
|
|
var ctrlKeyboardnumpad2 = new UnityEngine.InputSystem.Controls.KeyControl();
|
|
ctrlKeyboardnumpad2.Setup()
|
|
.At(this, 88)
|
|
.WithParent(parent)
|
|
.WithName("numpad2")
|
|
.WithDisplayName("Numpad 2")
|
|
.WithLayout(kKeyLayout)
|
|
.IsButton(true)
|
|
.WithStateBlock(new InputStateBlock
|
|
{
|
|
format = new FourCC(1112101920),
|
|
byteOffset = 0,
|
|
bitOffset = 86,
|
|
sizeInBits = 1
|
|
})
|
|
.WithMinAndMax(0, 1)
|
|
.Finish();
|
|
ctrlKeyboardnumpad2.keyCode = UnityEngine.InputSystem.Key.Numpad2;
|
|
return ctrlKeyboardnumpad2;
|
|
}
|
|
|
|
private UnityEngine.InputSystem.Controls.KeyControl Initialize_ctrlKeyboardnumpad3(InternedString kKeyLayout, InputControl parent)
|
|
{
|
|
var ctrlKeyboardnumpad3 = new UnityEngine.InputSystem.Controls.KeyControl();
|
|
ctrlKeyboardnumpad3.Setup()
|
|
.At(this, 89)
|
|
.WithParent(parent)
|
|
.WithName("numpad3")
|
|
.WithDisplayName("Numpad 3")
|
|
.WithLayout(kKeyLayout)
|
|
.IsButton(true)
|
|
.WithStateBlock(new InputStateBlock
|
|
{
|
|
format = new FourCC(1112101920),
|
|
byteOffset = 0,
|
|
bitOffset = 87,
|
|
sizeInBits = 1
|
|
})
|
|
.WithMinAndMax(0, 1)
|
|
.Finish();
|
|
ctrlKeyboardnumpad3.keyCode = UnityEngine.InputSystem.Key.Numpad3;
|
|
return ctrlKeyboardnumpad3;
|
|
}
|
|
|
|
private UnityEngine.InputSystem.Controls.KeyControl Initialize_ctrlKeyboardnumpad4(InternedString kKeyLayout, InputControl parent)
|
|
{
|
|
var ctrlKeyboardnumpad4 = new UnityEngine.InputSystem.Controls.KeyControl();
|
|
ctrlKeyboardnumpad4.Setup()
|
|
.At(this, 90)
|
|
.WithParent(parent)
|
|
.WithName("numpad4")
|
|
.WithDisplayName("Numpad 4")
|
|
.WithLayout(kKeyLayout)
|
|
.IsButton(true)
|
|
.WithStateBlock(new InputStateBlock
|
|
{
|
|
format = new FourCC(1112101920),
|
|
byteOffset = 0,
|
|
bitOffset = 88,
|
|
sizeInBits = 1
|
|
})
|
|
.WithMinAndMax(0, 1)
|
|
.Finish();
|
|
ctrlKeyboardnumpad4.keyCode = UnityEngine.InputSystem.Key.Numpad4;
|
|
return ctrlKeyboardnumpad4;
|
|
}
|
|
|
|
private UnityEngine.InputSystem.Controls.KeyControl Initialize_ctrlKeyboardnumpad5(InternedString kKeyLayout, InputControl parent)
|
|
{
|
|
var ctrlKeyboardnumpad5 = new UnityEngine.InputSystem.Controls.KeyControl();
|
|
ctrlKeyboardnumpad5.Setup()
|
|
.At(this, 91)
|
|
.WithParent(parent)
|
|
.WithName("numpad5")
|
|
.WithDisplayName("Numpad 5")
|
|
.WithLayout(kKeyLayout)
|
|
.IsButton(true)
|
|
.WithStateBlock(new InputStateBlock
|
|
{
|
|
format = new FourCC(1112101920),
|
|
byteOffset = 0,
|
|
bitOffset = 89,
|
|
sizeInBits = 1
|
|
})
|
|
.WithMinAndMax(0, 1)
|
|
.Finish();
|
|
ctrlKeyboardnumpad5.keyCode = UnityEngine.InputSystem.Key.Numpad5;
|
|
return ctrlKeyboardnumpad5;
|
|
}
|
|
|
|
private UnityEngine.InputSystem.Controls.KeyControl Initialize_ctrlKeyboardnumpad6(InternedString kKeyLayout, InputControl parent)
|
|
{
|
|
var ctrlKeyboardnumpad6 = new UnityEngine.InputSystem.Controls.KeyControl();
|
|
ctrlKeyboardnumpad6.Setup()
|
|
.At(this, 92)
|
|
.WithParent(parent)
|
|
.WithName("numpad6")
|
|
.WithDisplayName("Numpad 6")
|
|
.WithLayout(kKeyLayout)
|
|
.IsButton(true)
|
|
.WithStateBlock(new InputStateBlock
|
|
{
|
|
format = new FourCC(1112101920),
|
|
byteOffset = 0,
|
|
bitOffset = 90,
|
|
sizeInBits = 1
|
|
})
|
|
.WithMinAndMax(0, 1)
|
|
.Finish();
|
|
ctrlKeyboardnumpad6.keyCode = UnityEngine.InputSystem.Key.Numpad6;
|
|
return ctrlKeyboardnumpad6;
|
|
}
|
|
|
|
private UnityEngine.InputSystem.Controls.KeyControl Initialize_ctrlKeyboardnumpad7(InternedString kKeyLayout, InputControl parent)
|
|
{
|
|
var ctrlKeyboardnumpad7 = new UnityEngine.InputSystem.Controls.KeyControl();
|
|
ctrlKeyboardnumpad7.Setup()
|
|
.At(this, 93)
|
|
.WithParent(parent)
|
|
.WithName("numpad7")
|
|
.WithDisplayName("Numpad 7")
|
|
.WithLayout(kKeyLayout)
|
|
.IsButton(true)
|
|
.WithStateBlock(new InputStateBlock
|
|
{
|
|
format = new FourCC(1112101920),
|
|
byteOffset = 0,
|
|
bitOffset = 91,
|
|
sizeInBits = 1
|
|
})
|
|
.WithMinAndMax(0, 1)
|
|
.Finish();
|
|
ctrlKeyboardnumpad7.keyCode = UnityEngine.InputSystem.Key.Numpad7;
|
|
return ctrlKeyboardnumpad7;
|
|
}
|
|
|
|
private UnityEngine.InputSystem.Controls.KeyControl Initialize_ctrlKeyboardnumpad8(InternedString kKeyLayout, InputControl parent)
|
|
{
|
|
var ctrlKeyboardnumpad8 = new UnityEngine.InputSystem.Controls.KeyControl();
|
|
ctrlKeyboardnumpad8.Setup()
|
|
.At(this, 94)
|
|
.WithParent(parent)
|
|
.WithName("numpad8")
|
|
.WithDisplayName("Numpad 8")
|
|
.WithLayout(kKeyLayout)
|
|
.IsButton(true)
|
|
.WithStateBlock(new InputStateBlock
|
|
{
|
|
format = new FourCC(1112101920),
|
|
byteOffset = 0,
|
|
bitOffset = 92,
|
|
sizeInBits = 1
|
|
})
|
|
.WithMinAndMax(0, 1)
|
|
.Finish();
|
|
ctrlKeyboardnumpad8.keyCode = UnityEngine.InputSystem.Key.Numpad8;
|
|
return ctrlKeyboardnumpad8;
|
|
}
|
|
|
|
private UnityEngine.InputSystem.Controls.KeyControl Initialize_ctrlKeyboardnumpad9(InternedString kKeyLayout, InputControl parent)
|
|
{
|
|
var ctrlKeyboardnumpad9 = new UnityEngine.InputSystem.Controls.KeyControl();
|
|
ctrlKeyboardnumpad9.Setup()
|
|
.At(this, 95)
|
|
.WithParent(parent)
|
|
.WithName("numpad9")
|
|
.WithDisplayName("Numpad 9")
|
|
.WithLayout(kKeyLayout)
|
|
.IsButton(true)
|
|
.WithStateBlock(new InputStateBlock
|
|
{
|
|
format = new FourCC(1112101920),
|
|
byteOffset = 0,
|
|
bitOffset = 93,
|
|
sizeInBits = 1
|
|
})
|
|
.WithMinAndMax(0, 1)
|
|
.Finish();
|
|
ctrlKeyboardnumpad9.keyCode = UnityEngine.InputSystem.Key.Numpad9;
|
|
return ctrlKeyboardnumpad9;
|
|
}
|
|
|
|
private UnityEngine.InputSystem.Controls.KeyControl Initialize_ctrlKeyboardnumpad0(InternedString kKeyLayout, InputControl parent)
|
|
{
|
|
var ctrlKeyboardnumpad0 = new UnityEngine.InputSystem.Controls.KeyControl();
|
|
ctrlKeyboardnumpad0.Setup()
|
|
.At(this, 96)
|
|
.WithParent(parent)
|
|
.WithName("numpad0")
|
|
.WithDisplayName("Numpad 0")
|
|
.WithLayout(kKeyLayout)
|
|
.IsButton(true)
|
|
.WithStateBlock(new InputStateBlock
|
|
{
|
|
format = new FourCC(1112101920),
|
|
byteOffset = 0,
|
|
bitOffset = 84,
|
|
sizeInBits = 1
|
|
})
|
|
.WithMinAndMax(0, 1)
|
|
.Finish();
|
|
ctrlKeyboardnumpad0.keyCode = UnityEngine.InputSystem.Key.Numpad0;
|
|
return ctrlKeyboardnumpad0;
|
|
}
|
|
|
|
private UnityEngine.InputSystem.Controls.KeyControl Initialize_ctrlKeyboardf1(InternedString kKeyLayout, InputControl parent)
|
|
{
|
|
var ctrlKeyboardf1 = new UnityEngine.InputSystem.Controls.KeyControl();
|
|
ctrlKeyboardf1.Setup()
|
|
.At(this, 97)
|
|
.WithParent(parent)
|
|
.WithName("f1")
|
|
.WithDisplayName("F1")
|
|
.WithLayout(kKeyLayout)
|
|
.IsButton(true)
|
|
.WithStateBlock(new InputStateBlock
|
|
{
|
|
format = new FourCC(1112101920),
|
|
byteOffset = 0,
|
|
bitOffset = 94,
|
|
sizeInBits = 1
|
|
})
|
|
.WithMinAndMax(0, 1)
|
|
.Finish();
|
|
ctrlKeyboardf1.keyCode = UnityEngine.InputSystem.Key.F1;
|
|
return ctrlKeyboardf1;
|
|
}
|
|
|
|
private UnityEngine.InputSystem.Controls.KeyControl Initialize_ctrlKeyboardf2(InternedString kKeyLayout, InputControl parent)
|
|
{
|
|
var ctrlKeyboardf2 = new UnityEngine.InputSystem.Controls.KeyControl();
|
|
ctrlKeyboardf2.Setup()
|
|
.At(this, 98)
|
|
.WithParent(parent)
|
|
.WithName("f2")
|
|
.WithDisplayName("F2")
|
|
.WithLayout(kKeyLayout)
|
|
.IsButton(true)
|
|
.WithStateBlock(new InputStateBlock
|
|
{
|
|
format = new FourCC(1112101920),
|
|
byteOffset = 0,
|
|
bitOffset = 95,
|
|
sizeInBits = 1
|
|
})
|
|
.WithMinAndMax(0, 1)
|
|
.Finish();
|
|
ctrlKeyboardf2.keyCode = UnityEngine.InputSystem.Key.F2;
|
|
return ctrlKeyboardf2;
|
|
}
|
|
|
|
private UnityEngine.InputSystem.Controls.KeyControl Initialize_ctrlKeyboardf3(InternedString kKeyLayout, InputControl parent)
|
|
{
|
|
var ctrlKeyboardf3 = new UnityEngine.InputSystem.Controls.KeyControl();
|
|
ctrlKeyboardf3.Setup()
|
|
.At(this, 99)
|
|
.WithParent(parent)
|
|
.WithName("f3")
|
|
.WithDisplayName("F3")
|
|
.WithLayout(kKeyLayout)
|
|
.IsButton(true)
|
|
.WithStateBlock(new InputStateBlock
|
|
{
|
|
format = new FourCC(1112101920),
|
|
byteOffset = 0,
|
|
bitOffset = 96,
|
|
sizeInBits = 1
|
|
})
|
|
.WithMinAndMax(0, 1)
|
|
.Finish();
|
|
ctrlKeyboardf3.keyCode = UnityEngine.InputSystem.Key.F3;
|
|
return ctrlKeyboardf3;
|
|
}
|
|
|
|
private UnityEngine.InputSystem.Controls.KeyControl Initialize_ctrlKeyboardf4(InternedString kKeyLayout, InputControl parent)
|
|
{
|
|
var ctrlKeyboardf4 = new UnityEngine.InputSystem.Controls.KeyControl();
|
|
ctrlKeyboardf4.Setup()
|
|
.At(this, 100)
|
|
.WithParent(parent)
|
|
.WithName("f4")
|
|
.WithDisplayName("F4")
|
|
.WithLayout(kKeyLayout)
|
|
.IsButton(true)
|
|
.WithStateBlock(new InputStateBlock
|
|
{
|
|
format = new FourCC(1112101920),
|
|
byteOffset = 0,
|
|
bitOffset = 97,
|
|
sizeInBits = 1
|
|
})
|
|
.WithMinAndMax(0, 1)
|
|
.Finish();
|
|
ctrlKeyboardf4.keyCode = UnityEngine.InputSystem.Key.F4;
|
|
return ctrlKeyboardf4;
|
|
}
|
|
|
|
private UnityEngine.InputSystem.Controls.KeyControl Initialize_ctrlKeyboardf5(InternedString kKeyLayout, InputControl parent)
|
|
{
|
|
var ctrlKeyboardf5 = new UnityEngine.InputSystem.Controls.KeyControl();
|
|
ctrlKeyboardf5.Setup()
|
|
.At(this, 101)
|
|
.WithParent(parent)
|
|
.WithName("f5")
|
|
.WithDisplayName("F5")
|
|
.WithLayout(kKeyLayout)
|
|
.IsButton(true)
|
|
.WithStateBlock(new InputStateBlock
|
|
{
|
|
format = new FourCC(1112101920),
|
|
byteOffset = 0,
|
|
bitOffset = 98,
|
|
sizeInBits = 1
|
|
})
|
|
.WithMinAndMax(0, 1)
|
|
.Finish();
|
|
ctrlKeyboardf5.keyCode = UnityEngine.InputSystem.Key.F5;
|
|
return ctrlKeyboardf5;
|
|
}
|
|
|
|
private UnityEngine.InputSystem.Controls.KeyControl Initialize_ctrlKeyboardf6(InternedString kKeyLayout, InputControl parent)
|
|
{
|
|
var ctrlKeyboardf6 = new UnityEngine.InputSystem.Controls.KeyControl();
|
|
ctrlKeyboardf6.Setup()
|
|
.At(this, 102)
|
|
.WithParent(parent)
|
|
.WithName("f6")
|
|
.WithDisplayName("F6")
|
|
.WithLayout(kKeyLayout)
|
|
.IsButton(true)
|
|
.WithStateBlock(new InputStateBlock
|
|
{
|
|
format = new FourCC(1112101920),
|
|
byteOffset = 0,
|
|
bitOffset = 99,
|
|
sizeInBits = 1
|
|
})
|
|
.WithMinAndMax(0, 1)
|
|
.Finish();
|
|
ctrlKeyboardf6.keyCode = UnityEngine.InputSystem.Key.F6;
|
|
return ctrlKeyboardf6;
|
|
}
|
|
|
|
private UnityEngine.InputSystem.Controls.KeyControl Initialize_ctrlKeyboardf7(InternedString kKeyLayout, InputControl parent)
|
|
{
|
|
var ctrlKeyboardf7 = new UnityEngine.InputSystem.Controls.KeyControl();
|
|
ctrlKeyboardf7.Setup()
|
|
.At(this, 103)
|
|
.WithParent(parent)
|
|
.WithName("f7")
|
|
.WithDisplayName("F7")
|
|
.WithLayout(kKeyLayout)
|
|
.IsButton(true)
|
|
.WithStateBlock(new InputStateBlock
|
|
{
|
|
format = new FourCC(1112101920),
|
|
byteOffset = 0,
|
|
bitOffset = 100,
|
|
sizeInBits = 1
|
|
})
|
|
.WithMinAndMax(0, 1)
|
|
.Finish();
|
|
ctrlKeyboardf7.keyCode = UnityEngine.InputSystem.Key.F7;
|
|
return ctrlKeyboardf7;
|
|
}
|
|
|
|
private UnityEngine.InputSystem.Controls.KeyControl Initialize_ctrlKeyboardf8(InternedString kKeyLayout, InputControl parent)
|
|
{
|
|
var ctrlKeyboardf8 = new UnityEngine.InputSystem.Controls.KeyControl();
|
|
ctrlKeyboardf8.Setup()
|
|
.At(this, 104)
|
|
.WithParent(parent)
|
|
.WithName("f8")
|
|
.WithDisplayName("F8")
|
|
.WithLayout(kKeyLayout)
|
|
.IsButton(true)
|
|
.WithStateBlock(new InputStateBlock
|
|
{
|
|
format = new FourCC(1112101920),
|
|
byteOffset = 0,
|
|
bitOffset = 101,
|
|
sizeInBits = 1
|
|
})
|
|
.WithMinAndMax(0, 1)
|
|
.Finish();
|
|
ctrlKeyboardf8.keyCode = UnityEngine.InputSystem.Key.F8;
|
|
return ctrlKeyboardf8;
|
|
}
|
|
|
|
private UnityEngine.InputSystem.Controls.KeyControl Initialize_ctrlKeyboardf9(InternedString kKeyLayout, InputControl parent)
|
|
{
|
|
var ctrlKeyboardf9 = new UnityEngine.InputSystem.Controls.KeyControl();
|
|
ctrlKeyboardf9.Setup()
|
|
.At(this, 105)
|
|
.WithParent(parent)
|
|
.WithName("f9")
|
|
.WithDisplayName("F9")
|
|
.WithLayout(kKeyLayout)
|
|
.IsButton(true)
|
|
.WithStateBlock(new InputStateBlock
|
|
{
|
|
format = new FourCC(1112101920),
|
|
byteOffset = 0,
|
|
bitOffset = 102,
|
|
sizeInBits = 1
|
|
})
|
|
.WithMinAndMax(0, 1)
|
|
.Finish();
|
|
ctrlKeyboardf9.keyCode = UnityEngine.InputSystem.Key.F9;
|
|
return ctrlKeyboardf9;
|
|
}
|
|
|
|
private UnityEngine.InputSystem.Controls.KeyControl Initialize_ctrlKeyboardf10(InternedString kKeyLayout, InputControl parent)
|
|
{
|
|
var ctrlKeyboardf10 = new UnityEngine.InputSystem.Controls.KeyControl();
|
|
ctrlKeyboardf10.Setup()
|
|
.At(this, 106)
|
|
.WithParent(parent)
|
|
.WithName("f10")
|
|
.WithDisplayName("F10")
|
|
.WithLayout(kKeyLayout)
|
|
.IsButton(true)
|
|
.WithStateBlock(new InputStateBlock
|
|
{
|
|
format = new FourCC(1112101920),
|
|
byteOffset = 0,
|
|
bitOffset = 103,
|
|
sizeInBits = 1
|
|
})
|
|
.WithMinAndMax(0, 1)
|
|
.Finish();
|
|
ctrlKeyboardf10.keyCode = UnityEngine.InputSystem.Key.F10;
|
|
return ctrlKeyboardf10;
|
|
}
|
|
|
|
private UnityEngine.InputSystem.Controls.KeyControl Initialize_ctrlKeyboardf11(InternedString kKeyLayout, InputControl parent)
|
|
{
|
|
var ctrlKeyboardf11 = new UnityEngine.InputSystem.Controls.KeyControl();
|
|
ctrlKeyboardf11.Setup()
|
|
.At(this, 107)
|
|
.WithParent(parent)
|
|
.WithName("f11")
|
|
.WithDisplayName("F11")
|
|
.WithLayout(kKeyLayout)
|
|
.IsButton(true)
|
|
.WithStateBlock(new InputStateBlock
|
|
{
|
|
format = new FourCC(1112101920),
|
|
byteOffset = 0,
|
|
bitOffset = 104,
|
|
sizeInBits = 1
|
|
})
|
|
.WithMinAndMax(0, 1)
|
|
.Finish();
|
|
ctrlKeyboardf11.keyCode = UnityEngine.InputSystem.Key.F11;
|
|
return ctrlKeyboardf11;
|
|
}
|
|
|
|
private UnityEngine.InputSystem.Controls.KeyControl Initialize_ctrlKeyboardf12(InternedString kKeyLayout, InputControl parent)
|
|
{
|
|
var ctrlKeyboardf12 = new UnityEngine.InputSystem.Controls.KeyControl();
|
|
ctrlKeyboardf12.Setup()
|
|
.At(this, 108)
|
|
.WithParent(parent)
|
|
.WithName("f12")
|
|
.WithDisplayName("F12")
|
|
.WithLayout(kKeyLayout)
|
|
.IsButton(true)
|
|
.WithStateBlock(new InputStateBlock
|
|
{
|
|
format = new FourCC(1112101920),
|
|
byteOffset = 0,
|
|
bitOffset = 105,
|
|
sizeInBits = 1
|
|
})
|
|
.WithMinAndMax(0, 1)
|
|
.Finish();
|
|
ctrlKeyboardf12.keyCode = UnityEngine.InputSystem.Key.F12;
|
|
return ctrlKeyboardf12;
|
|
}
|
|
|
|
private UnityEngine.InputSystem.Controls.KeyControl Initialize_ctrlKeyboardOEM1(InternedString kKeyLayout, InputControl parent)
|
|
{
|
|
var ctrlKeyboardOEM1 = new UnityEngine.InputSystem.Controls.KeyControl();
|
|
ctrlKeyboardOEM1.Setup()
|
|
.At(this, 109)
|
|
.WithParent(parent)
|
|
.WithName("OEM1")
|
|
.WithDisplayName("OEM1")
|
|
.WithLayout(kKeyLayout)
|
|
.IsButton(true)
|
|
.WithStateBlock(new InputStateBlock
|
|
{
|
|
format = new FourCC(1112101920),
|
|
byteOffset = 0,
|
|
bitOffset = 106,
|
|
sizeInBits = 1
|
|
})
|
|
.WithMinAndMax(0, 1)
|
|
.Finish();
|
|
ctrlKeyboardOEM1.keyCode = UnityEngine.InputSystem.Key.OEM1;
|
|
return ctrlKeyboardOEM1;
|
|
}
|
|
|
|
private UnityEngine.InputSystem.Controls.KeyControl Initialize_ctrlKeyboardOEM2(InternedString kKeyLayout, InputControl parent)
|
|
{
|
|
var ctrlKeyboardOEM2 = new UnityEngine.InputSystem.Controls.KeyControl();
|
|
ctrlKeyboardOEM2.Setup()
|
|
.At(this, 110)
|
|
.WithParent(parent)
|
|
.WithName("OEM2")
|
|
.WithDisplayName("OEM2")
|
|
.WithLayout(kKeyLayout)
|
|
.IsButton(true)
|
|
.WithStateBlock(new InputStateBlock
|
|
{
|
|
format = new FourCC(1112101920),
|
|
byteOffset = 0,
|
|
bitOffset = 107,
|
|
sizeInBits = 1
|
|
})
|
|
.WithMinAndMax(0, 1)
|
|
.Finish();
|
|
ctrlKeyboardOEM2.keyCode = UnityEngine.InputSystem.Key.OEM2;
|
|
return ctrlKeyboardOEM2;
|
|
}
|
|
|
|
private UnityEngine.InputSystem.Controls.KeyControl Initialize_ctrlKeyboardOEM3(InternedString kKeyLayout, InputControl parent)
|
|
{
|
|
var ctrlKeyboardOEM3 = new UnityEngine.InputSystem.Controls.KeyControl();
|
|
ctrlKeyboardOEM3.Setup()
|
|
.At(this, 111)
|
|
.WithParent(parent)
|
|
.WithName("OEM3")
|
|
.WithDisplayName("OEM3")
|
|
.WithLayout(kKeyLayout)
|
|
.IsButton(true)
|
|
.WithStateBlock(new InputStateBlock
|
|
{
|
|
format = new FourCC(1112101920),
|
|
byteOffset = 0,
|
|
bitOffset = 108,
|
|
sizeInBits = 1
|
|
})
|
|
.WithMinAndMax(0, 1)
|
|
.Finish();
|
|
ctrlKeyboardOEM3.keyCode = UnityEngine.InputSystem.Key.OEM3;
|
|
return ctrlKeyboardOEM3;
|
|
}
|
|
|
|
private UnityEngine.InputSystem.Controls.KeyControl Initialize_ctrlKeyboardOEM4(InternedString kKeyLayout, InputControl parent)
|
|
{
|
|
var ctrlKeyboardOEM4 = new UnityEngine.InputSystem.Controls.KeyControl();
|
|
ctrlKeyboardOEM4.Setup()
|
|
.At(this, 112)
|
|
.WithParent(parent)
|
|
.WithName("OEM4")
|
|
.WithDisplayName("OEM4")
|
|
.WithLayout(kKeyLayout)
|
|
.IsButton(true)
|
|
.WithStateBlock(new InputStateBlock
|
|
{
|
|
format = new FourCC(1112101920),
|
|
byteOffset = 0,
|
|
bitOffset = 109,
|
|
sizeInBits = 1
|
|
})
|
|
.WithMinAndMax(0, 1)
|
|
.Finish();
|
|
ctrlKeyboardOEM4.keyCode = UnityEngine.InputSystem.Key.OEM4;
|
|
return ctrlKeyboardOEM4;
|
|
}
|
|
|
|
private UnityEngine.InputSystem.Controls.KeyControl Initialize_ctrlKeyboardOEM5(InternedString kKeyLayout, InputControl parent)
|
|
{
|
|
var ctrlKeyboardOEM5 = new UnityEngine.InputSystem.Controls.KeyControl();
|
|
ctrlKeyboardOEM5.Setup()
|
|
.At(this, 113)
|
|
.WithParent(parent)
|
|
.WithName("OEM5")
|
|
.WithDisplayName("OEM5")
|
|
.WithLayout(kKeyLayout)
|
|
.IsButton(true)
|
|
.WithStateBlock(new InputStateBlock
|
|
{
|
|
format = new FourCC(1112101920),
|
|
byteOffset = 0,
|
|
bitOffset = 110,
|
|
sizeInBits = 1
|
|
})
|
|
.WithMinAndMax(0, 1)
|
|
.Finish();
|
|
ctrlKeyboardOEM5.keyCode = UnityEngine.InputSystem.Key.OEM5;
|
|
return ctrlKeyboardOEM5;
|
|
}
|
|
|
|
private UnityEngine.InputSystem.Controls.ButtonControl Initialize_ctrlKeyboardIMESelected(InternedString kButtonLayout, InputControl parent)
|
|
{
|
|
var ctrlKeyboardIMESelected = new UnityEngine.InputSystem.Controls.ButtonControl();
|
|
ctrlKeyboardIMESelected.Setup()
|
|
.At(this, 114)
|
|
.WithParent(parent)
|
|
.WithName("IMESelected")
|
|
.WithDisplayName("IMESelected")
|
|
.WithLayout(kButtonLayout)
|
|
.IsSynthetic(true)
|
|
.IsButton(true)
|
|
.WithStateBlock(new InputStateBlock
|
|
{
|
|
format = new FourCC(1112101920),
|
|
byteOffset = 0,
|
|
bitOffset = 111,
|
|
sizeInBits = 1
|
|
})
|
|
.WithMinAndMax(0, 1)
|
|
.Finish();
|
|
return ctrlKeyboardIMESelected;
|
|
}
|
|
}
|
|
}
|