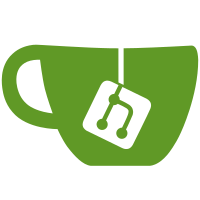
Got the formula for pocketpicking done. I am pretty happy with it. its based on value, weight, and your dexterity. the better your dexterity, and lower value and weight of the item, the better chance of stealing.
203 lines
9.8 KiB
C#
203 lines
9.8 KiB
C#
using System.Collections;
|
|
using System.Collections.Generic;
|
|
using UnityEngine;
|
|
using UnityEngine.UI;
|
|
using RPGCreationKit;
|
|
using RPGCreationKit.AI;
|
|
|
|
namespace RPGCreationKit
|
|
{
|
|
public class AmmoItemInLootingUI_DrawDeposit : AmmoItemInInventoryUI
|
|
{
|
|
public override void OnClick(bool takeAll = false)
|
|
{
|
|
if (LootingInventoryUI.instance.curIsPickPocket && LootingInventoryUI.instance.curLootingPoint != null)
|
|
{
|
|
// Player is attempting to pickpocket
|
|
// This is a VERY simple rule, if the weight of the item is over 1 the player will never be able to successfully pickpocket it
|
|
// You may want to change/alter this for your own projects
|
|
float B = (float)EntityAttributes.PlayerAttributes.attributes.Dexterity / 100f; // Dexterity - Your dexterity divided by 100. level 5 is 5% chance
|
|
float A = (1f - ((float)ammoItemInInventory.item.Value / 100f)); // Value - The value will work backwards starting at 50 Gold. if its value is 49, you have a 2% chance.
|
|
float C = (1f - ((float)ammoItemInInventory.item.Weight / 100f)); // Weight - the weight will work backwards at 50 LBS. if it is 49 LBS, you have a 2% chance
|
|
|
|
float ChanceForSuccess = ((A+B+C) / 3); // add the percenct, divide by 3 to get average. - Done.
|
|
|
|
// lets base it off chance.
|
|
|
|
// Could use Vector3.Dot to make less likely to succeed when infront of the AI
|
|
float RandomChance = (Random.value);
|
|
Debug.Log("Item: "+ammoItemInInventory.item.ItemName+"; A: "+A+"; B: "+B+"; C: "+C+"; ChanceForSuccess:"+ChanceForSuccess+"; RandomChance: "+RandomChance+";");
|
|
|
|
if (ChanceForSuccess < RandomChance) { // random selects a percent between 0% and 100%, and sees if yours is greater, if so, you have success.
|
|
// Failed chance check
|
|
RckAI ai = LootingInventoryUI.instance.curLootingPoint.GetComponent<RckAI>();
|
|
ai.TryEngageWithPlayer(); // Engage with player - Lets get faction instead of every fucker in the area.
|
|
LootingInventoryUI.instance.CloseUI();
|
|
return;
|
|
} else { // Reward Player
|
|
EntityAttributes.PlayerAttributes.attributes.DexterityPoints += 1;
|
|
A = (float)EntityAttributes.PlayerAttributes.attributes.DexterityPoints;
|
|
B = (float)EntityAttributes.PlayerAttributes.attributes.Dexterity;
|
|
while (A >= (B*3)) {
|
|
EntityAttributes.PlayerAttributes.attributes.Dexterity += 1;
|
|
float D = (A-(B*3f));
|
|
EntityAttributes.PlayerAttributes.attributes.DexterityPoints = (int)D;
|
|
A = (float)EntityAttributes.PlayerAttributes.attributes.DexterityPoints;
|
|
B = (float)EntityAttributes.PlayerAttributes.attributes.Dexterity;
|
|
}
|
|
}
|
|
}
|
|
// Some palce in the codebase seems to be calling this method after the CloseUI randomly, not sure why or where yet
|
|
// this is a backup in that case
|
|
if (LootingInventoryUI.instance.curIsPickPocket && LootingInventoryUI.instance.curLootingPoint == null) {
|
|
return;
|
|
}
|
|
|
|
if (LootingInventoryUI.instance.isDrawing)
|
|
{
|
|
// if the amount is 1, add it one time
|
|
if (base.ammoItemInInventory.Amount <= 1)
|
|
{
|
|
Inventory.PlayerInventory.AddItem(base.ammoItemInInventory.item, base.ammoItemInInventory.metadata, 1, !takeAll);
|
|
|
|
if (base.ammoItemInInventory.isEquipped)
|
|
LootingInventoryUI.instance.curLootingPoint.equipment.Unequip(base.ammoItemInInventory);
|
|
|
|
// Remove the item from the current loot inventory
|
|
LootingInventoryUI.instance.curLootingPoint.inventory.RemoveItem(base.ammoItemInInventory, 1);
|
|
|
|
LootingInventoryUI.instance.SelectNextButton();
|
|
|
|
// Disable this object
|
|
pool.usedObjects.Remove(this);
|
|
pool.AmmosPool.usedObjects.Remove(this);
|
|
|
|
gameObject.GetComponent<Button>().onClick.RemoveAllListeners();
|
|
gameObject.SetActive(false);
|
|
}
|
|
else if (base.ammoItemInInventory.item.isCumulable && base.ammoItemInInventory.Amount > 1)
|
|
{
|
|
if (!takeAll)
|
|
{
|
|
LootingInventoryUI.instance.takeDepositItemsPanel.gameObject.SetActive(true);
|
|
LootingInventoryUI.instance.takeDepositItemsPanel.Init(ammoItemInInventory, this);
|
|
}
|
|
else
|
|
{
|
|
ConfirmButtonCumulableItem(base.ammoItemInInventory.Amount);
|
|
}
|
|
}
|
|
} else // we're depositing
|
|
{
|
|
if(base.ammoItemInInventory.item.QuestItem)
|
|
{
|
|
AlertMessage.instance.InitAlertMessage("You can't leave Quest Items", AlertMessage.DEFAULT_MESSAGE_DURATION_MEDIUM);
|
|
return;
|
|
}
|
|
|
|
// if the amount is 1, add it one time
|
|
if (base.ammoItemInInventory.Amount <= 1)
|
|
{
|
|
// Remove the item from the current loot inventory
|
|
LootingInventoryUI.instance.curLootingPoint.inventory.AddItem(base.ammoItemInInventory.item, base.ammoItemInInventory.metadata, 1);
|
|
|
|
if (ammoItemInInventory.isEquipped)
|
|
{
|
|
Equipment.PlayerEquipment.Unequip(ammoItemInInventory);
|
|
PlayerCombat.instance.OnEquipmentChanges();
|
|
PlayerInInventory.instance.OnEquipmentChangesHands();
|
|
PlayerInInventory.instance.OnEquipmentChangesAmmo();
|
|
}
|
|
|
|
Inventory.PlayerInventory.RemoveItem(base.ammoItemInInventory, 1);
|
|
|
|
LootingInventoryUI.instance.SelectNextButton();
|
|
|
|
// Disable this object
|
|
pool.usedObjects.Remove(this);
|
|
pool.AmmosPool.usedObjects.Remove(this);
|
|
|
|
gameObject.GetComponent<Button>().onClick.RemoveAllListeners();
|
|
gameObject.SetActive(false);
|
|
}
|
|
else if (base.ammoItemInInventory.item.isCumulable && base.ammoItemInInventory.Amount > 1)
|
|
{
|
|
LootingInventoryUI.instance.takeDepositItemsPanel.gameObject.SetActive(true);
|
|
LootingInventoryUI.instance.takeDepositItemsPanel.Init(ammoItemInInventory, this);
|
|
}
|
|
}
|
|
}
|
|
|
|
public override void ConfirmButtonCumulableItem(int amount)
|
|
{
|
|
if (LootingInventoryUI.instance.isDrawing)
|
|
{
|
|
// Add in PlayerInventory
|
|
Inventory.PlayerInventory.AddItem(base.ammoItemInInventory.item, base.ammoItemInInventory.metadata, amount);
|
|
|
|
if (base.ammoItemInInventory.Amount <= amount)
|
|
if (base.ammoItemInInventory.isEquipped)
|
|
LootingInventoryUI.instance.curLootingPoint.equipment.Unequip(base.ammoItemInInventory);
|
|
|
|
// Remove from Loot Inventory
|
|
LootingInventoryUI.instance.curLootingPoint.inventory.RemoveItem(base.ammoItemInInventory, amount);
|
|
|
|
if (ammoItemInInventory.Amount <= 0)
|
|
{
|
|
LootingInventoryUI.instance.takeDepositItemsPanel.SetPreviousSelected();
|
|
LootingInventoryUI.instance.SelectNextButton(true);
|
|
|
|
//If we've took all the items
|
|
pool.usedObjects.Remove(this);
|
|
pool.AmmosPool.usedObjects.Remove(this);
|
|
|
|
|
|
// Disable this object
|
|
gameObject.GetComponent<Button>().onClick.RemoveAllListeners();
|
|
gameObject.SetActive(false);
|
|
}
|
|
else
|
|
{
|
|
// Update the UI
|
|
Init();
|
|
}
|
|
}
|
|
else // we're depositing
|
|
{
|
|
// Add it to Loot Inventory
|
|
LootingInventoryUI.instance.curLootingPoint.inventory.AddItem(base.ammoItemInInventory.item, base.ammoItemInInventory.metadata, amount);
|
|
|
|
if (base.ammoItemInInventory.Amount <= amount)
|
|
if (ammoItemInInventory.isEquipped)
|
|
{
|
|
Equipment.PlayerEquipment.Unequip(ammoItemInInventory);
|
|
PlayerCombat.instance.OnEquipmentChanges();
|
|
PlayerInInventory.instance.OnEquipmentChangesHands();
|
|
PlayerInInventory.instance.OnEquipmentChangesAmmo();
|
|
}
|
|
|
|
// Remove in PlayerInventory
|
|
Inventory.PlayerInventory.RemoveItem(base.ammoItemInInventory, amount);
|
|
|
|
if (ammoItemInInventory.Amount <= 0)
|
|
{
|
|
LootingInventoryUI.instance.takeDepositItemsPanel.SetPreviousSelected();
|
|
LootingInventoryUI.instance.SelectNextButton(true);
|
|
|
|
//If we've took all the items
|
|
pool.usedObjects.Remove(this);
|
|
pool.AmmosPool.usedObjects.Remove(this);
|
|
|
|
// Disable this object
|
|
gameObject.GetComponent<Button>().onClick.RemoveAllListeners();
|
|
gameObject.SetActive(false);
|
|
}
|
|
else
|
|
{
|
|
// Update the UI
|
|
Init();
|
|
}
|
|
}
|
|
}
|
|
}
|
|
} |