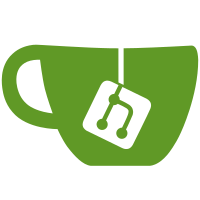
There is an asset in the store I grabbed. the coding is WAY above my head, I got about half of it and integrated and adapted what I can to it. im going as far as I can with it and ill come back in a few month when I understand t better.
63 lines
2.3 KiB
C#
63 lines
2.3 KiB
C#
using System.Collections;
|
|
using System.Collections.Generic;
|
|
using System.Reflection;
|
|
using NUnit.Framework;
|
|
using UnityEditor.AddressableAssets.Build.AnalyzeRules;
|
|
using UnityEditor.AddressableAssets.Build.BuildPipelineTasks;
|
|
using UnityEditor.AddressableAssets.Build.DataBuilders;
|
|
using UnityEditor.AddressableAssets.Settings;
|
|
using UnityEditor.AddressableAssets.Settings.GroupSchemas;
|
|
using UnityEditor.AddressableAssets.Tests;
|
|
using UnityEditor.Build.Pipeline;
|
|
using UnityEditor.Build.Pipeline.Injector;
|
|
using UnityEngine;
|
|
|
|
namespace UnityEditor.AddressableAssets.Tests.AnalyzeRules
|
|
{
|
|
[TestFixture]
|
|
public class AnalyzeRuleBaseTests : AddressableAssetTestBase
|
|
{
|
|
[Test]
|
|
public void ConvertBundleNamesToGroupNames()
|
|
{
|
|
var bundleName = "2398471298347129034_bundlename_1";
|
|
var fakeFileName = "archive://3912983hf9sdf902340jidf";
|
|
var convertedBundleName = "group1_bundlename_1";
|
|
|
|
var group = Settings.CreateGroup("group1", false, false, false, null, typeof(BundledAssetGroupSchema));
|
|
|
|
AddressableAssetsBuildContext context = new AddressableAssetsBuildContext();
|
|
context.Settings = Settings;
|
|
context.assetGroupToBundles = new Dictionary<AddressableAssetGroup, List<string>>()
|
|
{
|
|
{group, new List<string>() {bundleName}}
|
|
};
|
|
|
|
BundleRuleBase baseRule = new BundleRuleBase();
|
|
baseRule.m_ExtractData = new ExtractDataTask();
|
|
|
|
var field = typeof(ExtractDataTask).GetField("m_WriteData", BindingFlags.NonPublic | BindingFlags.Instance);
|
|
field.SetValue(baseRule.m_ExtractData, new BundleWriteData());
|
|
|
|
baseRule.m_AllBundleInputDefs.Add(new AssetBundleBuild()
|
|
{
|
|
assetBundleName = bundleName
|
|
});
|
|
|
|
baseRule.m_ExtractData.WriteData.FileToBundle.Add(fakeFileName, bundleName);
|
|
baseRule.ConvertBundleNamesToGroupNames(context);
|
|
|
|
Assert.AreEqual(convertedBundleName, baseRule.m_ExtractData.WriteData.FileToBundle[fakeFileName]);
|
|
|
|
Settings.RemoveGroup(group);
|
|
}
|
|
|
|
[Test]
|
|
public void BaseAnalyzeRule_DoesNotThrowOnFix()
|
|
{
|
|
BundleRuleBase baseRule = new BundleRuleBase();
|
|
Assert.DoesNotThrow(() => baseRule.FixIssues(Settings));
|
|
}
|
|
}
|
|
}
|