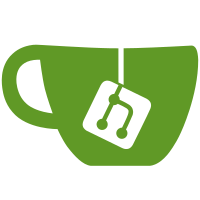
There is an asset in the store I grabbed. the coding is WAY above my head, I got about half of it and integrated and adapted what I can to it. im going as far as I can with it and ill come back in a few month when I understand t better.
62 lines
2.0 KiB
C#
62 lines
2.0 KiB
C#
using System;
|
|
using UnityEngine;
|
|
|
|
namespace UnityEditor.AddressableAssets.Build
|
|
{
|
|
/// <summary>
|
|
/// The result of IDataBuilder.Build.
|
|
/// </summary>
|
|
public interface IDataBuilderResult
|
|
{
|
|
/// <summary>
|
|
/// Duration of the build in seconds.
|
|
/// </summary>
|
|
double Duration { get; set; }
|
|
/// <summary>
|
|
/// The number of addressable assets contained in the build.
|
|
/// </summary>
|
|
int LocationCount { get; set; }
|
|
/// <summary>
|
|
/// Error string, if any. If Succeeded is true, this may be null.
|
|
/// </summary>
|
|
string Error { get; set; }
|
|
/// <summary>
|
|
/// Path of runtime settings file
|
|
/// </summary>
|
|
string OutputPath { get; set; }
|
|
/// <summary>
|
|
/// Registry of files created during the build
|
|
/// </summary>
|
|
FileRegistry FileRegistry { get; set; }
|
|
}
|
|
|
|
/// <summary>
|
|
/// Builds objects of type IDataBuilderResult.
|
|
/// </summary>
|
|
public interface IDataBuilder
|
|
{
|
|
/// <summary>
|
|
/// The name of the builder, used for GUI.
|
|
/// </summary>
|
|
string Name { get; }
|
|
/// <summary>
|
|
/// Can this builder build the type of data requested.
|
|
/// </summary>
|
|
/// <typeparam name="T">The data type.</typeparam>
|
|
/// <returns>True if the build can build it.</returns>
|
|
bool CanBuildData<T>() where T : IDataBuilderResult;
|
|
/// <summary>
|
|
/// Build the data of a specific type.
|
|
/// </summary>
|
|
/// <typeparam name="TResult">The data type.</typeparam>
|
|
/// <param name="builderInput">The builderInput used to build the data.</param>
|
|
/// <returns>The built data.</returns>
|
|
TResult BuildData<TResult>(AddressablesDataBuilderInput builderInput) where TResult : IDataBuilderResult;
|
|
|
|
/// <summary>
|
|
/// Clears all cached data.
|
|
/// </summary>
|
|
void ClearCachedData();
|
|
}
|
|
}
|