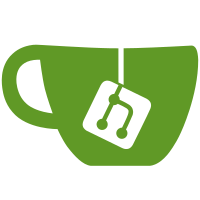
made most of the game Moddable. There is an in editor tool to make the mods and set them all up, and im making an external tool that will show your mods, lets you edit any information and it will pack it up and upload it. currently working on the uploading. Next I will make the game able to download and install. - Fuck Nexus.
158 lines
4.1 KiB
C#
158 lines
4.1 KiB
C#
using UnityEngine;
|
|
using RPGCreationKit;
|
|
using RPGCreationKit.SaveSystem;
|
|
|
|
namespace RPGCreationKit
|
|
{
|
|
[ExecuteAlways]
|
|
public class SunLightManager : MonoBehaviour
|
|
{
|
|
public static SunLightManager instance;
|
|
private bool Loaded = false;
|
|
private float TimeTrigger = 0f;
|
|
|
|
[Header("References")]
|
|
[SerializeField] private Light DirectionalLight;
|
|
[SerializeField] public SunLightPreset Preset;
|
|
[SerializeField] private Transform StarsSky;
|
|
[SerializeField] private Transform Player;
|
|
[SerializeField] private LightingSettings InteriorLS;
|
|
[SerializeField] private LightingSettings ExteriorLS;
|
|
|
|
[Header("Time")]
|
|
[SerializeField] public int Year = 0;
|
|
[SerializeField] public int Month = 1;
|
|
[SerializeField] public int Day = 1;
|
|
[SerializeField, Range(0, 24)] public float TimeOfDay;
|
|
|
|
private Material StarsMat;
|
|
public bool IsInterior = false;
|
|
|
|
void Start() {
|
|
StarsMat = null;
|
|
StarsMat = StarsSky.GetComponent<ParticleSystem>().GetComponent<Renderer>().sharedMaterial;
|
|
|
|
Year = SaveSystemManager.instance.saveFile.Environment.Year;
|
|
Month = SaveSystemManager.instance.saveFile.Environment.Month;
|
|
Day = SaveSystemManager.instance.saveFile.Environment.Day;
|
|
TimeOfDay = SaveSystemManager.instance.saveFile.Environment.TimeOfDay;
|
|
|
|
Loaded = true;
|
|
}
|
|
|
|
// Update is called once per frame
|
|
void Update() {
|
|
if (TimeTrigger > TimeOfDay) { // Should only trigger once a day.
|
|
Day += 1;
|
|
}
|
|
|
|
TimeTrigger = TimeOfDay;
|
|
|
|
if (Day > 28) {
|
|
Day = 1;
|
|
Month += 1;
|
|
}
|
|
|
|
if (Month > 13) {
|
|
Month = 1;
|
|
Year += 1;
|
|
}
|
|
|
|
if (Loaded == false) {
|
|
return;
|
|
}
|
|
|
|
if (Preset == null) {
|
|
return;
|
|
}
|
|
|
|
if (Application.isPlaying) {
|
|
TimeOfDay += (Time.deltaTime / 180f);
|
|
TimeOfDay %= 24;
|
|
float TimePercent = TimeOfDay / 24f;
|
|
|
|
Color WorldBrightness = Preset.WorldBrightness.Evaluate(TimePercent);
|
|
|
|
if (IsInterior == false) {
|
|
|
|
// Setting the Sun
|
|
if (DirectionalLight.gameObject.activeSelf == true) {
|
|
if (RenderSettings.sun != DirectionalLight) {
|
|
RenderSettings.sun = DirectionalLight;
|
|
|
|
}
|
|
}
|
|
|
|
// Setting the Sun
|
|
Color DirectionalTempColor = Preset.DirectionalColor.Evaluate(TimePercent);
|
|
DirectionalLight.color = DirectionalTempColor;
|
|
RenderSettings.skybox.SetFloat("_Exposure", (DirectionalTempColor.a * 5));
|
|
|
|
// Setting the Ambiance Lighting
|
|
//InteriorLS.indirectScale = WorldBrightness.a * 1.5f;
|
|
} else {
|
|
//ExteriorLS.indirectScale = WorldBrightness.a * 1.5f;
|
|
}
|
|
UpdateLighting(TimePercent);
|
|
}
|
|
|
|
}
|
|
|
|
private void UpdateLighting(float TimePercent) {
|
|
RenderSettings.ambientLight = Preset.AmbientColor.Evaluate(TimePercent);
|
|
|
|
// Fog
|
|
Color FogTempColor = Preset.FogColor.Evaluate(TimePercent);
|
|
RenderSettings.fogColor = FogTempColor;
|
|
RenderSettings.fogDensity = ((FogTempColor.a / 10) * 2);
|
|
|
|
// Setting the stars
|
|
StarsSky.position = Player.position;
|
|
Color StarsColor = Preset.StarsVisability.Evaluate(TimePercent);
|
|
Color color = StarsMat.color;
|
|
color.a = StarsColor.a;
|
|
StarsMat.color = color;
|
|
|
|
// Sun Intensity (Item reflections power)
|
|
Color SunTempInt = Preset.SunIntensity.Evaluate(TimePercent);
|
|
DirectionalLight.intensity = ((SunTempInt.a) * 1.5f);
|
|
|
|
if (DirectionalLight != null) {
|
|
|
|
DirectionalLight.transform.localRotation = Quaternion.Euler(new Vector3((TimePercent * 360f) -90f, 170f, 0f));
|
|
StarsSky.localRotation = Quaternion.Euler(new Vector3((TimePercent * 360f) -90f, -100, 40));
|
|
}
|
|
}
|
|
|
|
public string GetMonth(int A) { // Ari, Tau, Gen, Can, Le, Ver, Lib, Sco, Sol, Sag, Cap, Aua, Pis.
|
|
if (A == 0) {
|
|
return "Aries";
|
|
} else if (A == 1) {
|
|
return "Taurus";
|
|
} else if (A == 2) {
|
|
return "Gemini";
|
|
} else if (A == 3) {
|
|
return "Cancer";
|
|
} else if (A == 4) {
|
|
return "Leo";
|
|
} else if (A == 5) {
|
|
return "Virgo";
|
|
} else if (A == 6) {
|
|
return "Libra";
|
|
} else if (A == 7) {
|
|
return "Scorpio";
|
|
} else if (A == 8) {
|
|
return "Sol";
|
|
} else if (A == 9) {
|
|
return "Sagittarius";
|
|
} else if (A == 10) {
|
|
return "Capricorn";
|
|
} else if (A == 11) {
|
|
return "Aquarius";
|
|
} else {
|
|
return "Pisces";
|
|
}
|
|
}
|
|
}
|
|
}
|