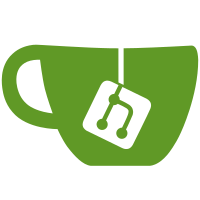
made most of the game Moddable. There is an in editor tool to make the mods and set them all up, and im making an external tool that will show your mods, lets you edit any information and it will pack it up and upload it. currently working on the uploading. Next I will make the game able to download and install. - Fuck Nexus.
209 lines
7.7 KiB
C#
209 lines
7.7 KiB
C#
using System.Collections;
|
|
using System.Collections.Generic;
|
|
using System;
|
|
using UnityEngine;
|
|
using UnityEngine.Networking;
|
|
using UnityEngine.UI;
|
|
using System.Text.RegularExpressions;
|
|
|
|
// From TestModManager
|
|
using UnityEngine.AddressableAssets;
|
|
using UnityEngine.ResourceManagement.ResourceLocations;
|
|
using UnityEngine.ResourceManagement.AsyncOperations;
|
|
using UnityEngine.AddressableAssets.ResourceLocators;
|
|
using System.IO;
|
|
using System.Linq;
|
|
|
|
public class ModPanal : MonoBehaviour
|
|
{
|
|
[Header("\tGame Specific Content")]
|
|
[SerializeField] private string Key;
|
|
[SerializeField] private int GameNumber;
|
|
|
|
[Header("\tFillables")]
|
|
[SerializeField] private OnlineAccountHandler Online;
|
|
[SerializeField] private GameObject ModPrefab;
|
|
[SerializeField] private GameObject CatPrefab;
|
|
[SerializeField] public List<GameObject> CreatedModPrefabs;
|
|
[SerializeField] public List<GameObject> CreatedCatPrefabs;
|
|
[SerializeField] private Transform ModContainer;
|
|
[SerializeField] private Transform CatContainer;
|
|
[SerializeField] private AudioSource Audio;
|
|
[SerializeField] private ModHolder modHolder;
|
|
|
|
public string dir;
|
|
|
|
// Start is called before the first frame update
|
|
void Start()
|
|
{
|
|
|
|
}
|
|
|
|
// Update is called once per frame
|
|
void Update()
|
|
{
|
|
|
|
}
|
|
|
|
public void GetOwnedMods() {
|
|
|
|
for (int j = 0; j < CreatedModPrefabs.Count; j++) {
|
|
CreatedModPrefabs[j].SetActive(false);
|
|
}
|
|
CreatedModPrefabs.Clear();
|
|
|
|
string root = Environment.GetFolderPath(Environment.SpecialFolder.MyDocuments)+@"\My Games\Firstborn\Mods";
|
|
|
|
//see if there is data in each folder, if so add it to the dictonary and spawn it in
|
|
foreach (var folder in Directory.GetDirectories(root).Select(d => Path.GetFileName(d)))
|
|
{
|
|
if (folder != "{ProcessFolder}") {
|
|
//try to get description data
|
|
using (var sr = new StreamReader(Path.Combine(root + "/"+ folder, "Info.fc1"))) {
|
|
string A = sr.ReadToEnd();
|
|
var NewMod = Instantiate(ModPrefab, ModContainer);
|
|
CreatedModPrefabs.Add(NewMod);
|
|
ModPrefab Mod = NewMod.GetComponent<ModPrefab>();
|
|
|
|
Mod.Title.text = folder;
|
|
Mod.Date.text = File.GetCreationTime(Path.Combine(root + "/"+ folder, "Info.fc1")).ToString();
|
|
Mod.Author.text = A.Split (new string[] { "Author:" }, StringSplitOptions.None)[1].Split (";")[0];
|
|
Mod.ModID = root + "/" + folder + "/mod.json";
|
|
Mod.Description.text = A.Split (new string[] { "Description:" }, StringSplitOptions.None)[1].Split (";")[0];
|
|
Mod.LoadSS(Path.Combine(root + "/"+ folder, "Image.png"));
|
|
Mod.GO = gameObject;
|
|
Mod.BS.source = Audio;
|
|
|
|
Debug.Log(A);
|
|
}
|
|
}
|
|
}
|
|
}
|
|
|
|
public void ActivateMod(string location) {
|
|
if (location == "") return;
|
|
|
|
//load from the directory were spawning from
|
|
AsyncOperationHandle<IResourceLocator> loadContentCatalogAsync = Addressables.LoadContentCatalogAsync(@"" + location);
|
|
|
|
//call this when were done loading in the content
|
|
loadContentCatalogAsync.Completed += OnCompleted;
|
|
}
|
|
|
|
private void OnCompleted(AsyncOperationHandle<IResourceLocator> obj)
|
|
{
|
|
//find prefabs in the addressable with the tag specified in the first parameter
|
|
IResourceLocator resourceLocator = obj.Result;
|
|
resourceLocator.Locate("Static Items", typeof(GameObject), out IList<IResourceLocation> locations);
|
|
|
|
//if there are locations in the adressable spawn them
|
|
if (locations != null)
|
|
{
|
|
foreach (IResourceLocation resourceLocation in locations)
|
|
{
|
|
GameObject resourceLocationData = (GameObject)resourceLocation.Data;
|
|
AsyncOperationHandle<GameObject> SpriteHandle = Addressables.LoadAsset<GameObject>(resourceLocation);
|
|
SpriteHandle.Completed += GameObjectLoaded;
|
|
}
|
|
}
|
|
}
|
|
|
|
private void GameObjectLoaded(AsyncOperationHandle<GameObject> handle)
|
|
{
|
|
if (handle.Status == AsyncOperationStatus.Succeeded)
|
|
{
|
|
GameObject result = handle.Result;
|
|
Debug.Log("result: "+result.name);
|
|
var NewMod = Instantiate(result, modHolder.gameObject.transform);
|
|
modHolder.Mods.Add(NewMod);
|
|
NewMod.SetActive(false);
|
|
}
|
|
}
|
|
|
|
public void GetCategory(int i) {
|
|
for (int j = 0; j < CreatedModPrefabs.Count; j++) {
|
|
CreatedModPrefabs[j].SetActive(false);
|
|
}
|
|
CreatedModPrefabs.Clear();
|
|
|
|
string url = "https://schaken-mods.com/api/downloads/files?key="+Key+"&categories="+i+"&sortDir=desc&hidden=0&page=1&sortDir=desc&hidden=0";
|
|
StartCoroutine(GetCategoryMods(url));
|
|
}
|
|
|
|
IEnumerator GetCategoryMods(string uri) {
|
|
UnityWebRequest uwr = UnityWebRequest.Get(uri);
|
|
yield return uwr.SendWebRequest();
|
|
if (uwr.result == UnityWebRequest.Result.ConnectionError) {
|
|
Debug.Log("Error While Sending: " + uwr.error);
|
|
} else {
|
|
// string FullTXT = uwr.downloadHandler.text.Replace(@"\", string.Empty);
|
|
string FullTXT = System.Text.RegularExpressions.Regex.Unescape(uwr.downloadHandler.text);
|
|
|
|
string BreakingPoints = "hasPendingVersion";
|
|
string[] Content = FullTXT.Split(BreakingPoints);
|
|
for (int i = 0; i < (Content.Length - 1); i++) {
|
|
var NewMod = Instantiate(ModPrefab, ModContainer);
|
|
CreatedModPrefabs.Add(NewMod);
|
|
ModPrefab Mod = NewMod.GetComponent<ModPrefab>();
|
|
|
|
string NewScreenshot = "hi";
|
|
if (Content[i].Contains("\"primaryScreenshotThumb\": null")) {
|
|
NewScreenshot = "https://schaken-mods.com/uploads/monthly_2021_11/NoImage.png.7598ee76366f585b2bb10610b640e551.png";
|
|
} else {
|
|
NewScreenshot = Content[i].Split (new string[] { "primaryScreenshotThumb" }, StringSplitOptions.None)[1].Split (',')[1].Split (new string[] { "\"url\": \"" }, StringSplitOptions.None)[1].Split ("\"")[0].Trim ();
|
|
}
|
|
Mod.Title.text = Content[i].Split (new string[] { "\"title\": \"" }, StringSplitOptions.None)[1].Split ("\"")[0].Trim ();
|
|
Mod.Date.text = DateTime.Parse(Content[i].Split (new string[] { "\"date\": \"" }, StringSplitOptions.None)[1].Split ("\"")[0].Trim ()).ToString();
|
|
Mod.Author.text = Content[i].Split (new string[] { "\"name\": \"" }, StringSplitOptions.None)[2].Split ("\"")[0].Trim ();
|
|
Mod.ModID = Content[i].Split (new string[] { "\"id\": " }, StringSplitOptions.None)[1].Split (",")[0].Trim ();
|
|
string EndDescription = "\",";
|
|
string OrigDescription = Content[i].Split (new string[] { "\"description\": \"" }, StringSplitOptions.None)[1].Split (EndDescription)[0].Trim ();
|
|
Mod.Description.text = Regex.Replace(OrigDescription, "<.*?>", String.Empty);
|
|
Mod.LoadSS(NewScreenshot);
|
|
}
|
|
|
|
}
|
|
}
|
|
|
|
public void GetCategoryList() {
|
|
|
|
for (int j = 0; j < CreatedCatPrefabs.Count; j++) {
|
|
CreatedCatPrefabs[j].SetActive(false);
|
|
}
|
|
CreatedCatPrefabs.Clear();
|
|
|
|
string url = "https://schaken-mods.com/api/downloads/categories?key="+Key+"&clubs=0&page=1&perPage=300";
|
|
StartCoroutine(GetCategoryMods(url, GameNumber));
|
|
}
|
|
|
|
IEnumerator GetCategoryMods(string uri, int j)
|
|
{
|
|
UnityWebRequest uwr = UnityWebRequest.Get(uri);
|
|
yield return uwr.SendWebRequest();
|
|
if (uwr.result == UnityWebRequest.Result.ConnectionError) {
|
|
Debug.Log("Error While Sending: " + uwr.error);
|
|
} else {
|
|
string FullTXT = System.Text.RegularExpressions.Regex.Unescape(uwr.downloadHandler.text);
|
|
Debug.Log("Mod Categories Full List: "+FullTXT);
|
|
|
|
string BreakingPoints = "\"perm_3\"";
|
|
string[] Content = FullTXT.Split(BreakingPoints);
|
|
Debug.Log("Content Count: "+Content.Length);
|
|
for (int i = 0; i < (Content.Length - 1); i++) {
|
|
if (Content[i].Contains("\"parentId\": "+j+",")) {
|
|
var NewCat = Instantiate(CatPrefab, CatContainer);
|
|
CreatedCatPrefabs.Add(NewCat);
|
|
CatItem Cat = NewCat.GetComponent<CatItem>();
|
|
Cat.CatName.text = Content[i].Split (new string[] { "\"name\": \"" }, StringSplitOptions.None)[1].Split ("\"")[0].Trim ();
|
|
Cat.CatID = Content[i].Split (new string[] { "\"id\": " }, StringSplitOptions.None)[1].Split (",")[0].Trim ();
|
|
ModPanal TempThis = gameObject.GetComponent<ModPanal>();
|
|
Cat.ModMan = TempThis;
|
|
}
|
|
}
|
|
|
|
}
|
|
|
|
}
|
|
|
|
}
|