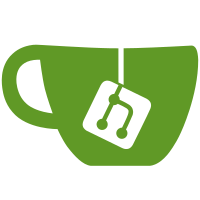
made most of the game Moddable. There is an in editor tool to make the mods and set them all up, and im making an external tool that will show your mods, lets you edit any information and it will pack it up and upload it. currently working on the uploading. Next I will make the game able to download and install. - Fuck Nexus.
314 lines
9.0 KiB
C#
314 lines
9.0 KiB
C#
using System.Collections;
|
|
using System.Collections.Generic;
|
|
using UnityEngine;
|
|
using UnityEngine.SceneManagement;
|
|
using System.IO;
|
|
using UnityEngine.UI;
|
|
using UnityEngine.Networking;
|
|
using TMPro;
|
|
using RPGCreationKit;
|
|
using RPGCreationKit.SaveSystem;
|
|
using UnityEngine.EventSystems;
|
|
using UnityEngine.Events;
|
|
|
|
/// <summary>
|
|
/// Contains base information for a save system panel such as the load/save panels
|
|
/// </summary>
|
|
public class RckSaveSystemPanel : MonoBehaviour
|
|
{
|
|
protected SaveGameButtonUI selectedSaveFile;
|
|
[SerializeField] private GameObject EnvironmentManager;
|
|
public SaveGameButtonUI SelectedSaveFile
|
|
{
|
|
get
|
|
{
|
|
return selectedSaveFile;
|
|
}
|
|
set
|
|
{
|
|
selectedSaveFile = value;
|
|
|
|
OnSelectedSavegameChanges();
|
|
}
|
|
}
|
|
|
|
public virtual void OnSelectedSavegameChanges()
|
|
{
|
|
|
|
}
|
|
|
|
}
|
|
|
|
namespace RPGCreationKit
|
|
{
|
|
public class LoadPanel : RckSaveSystemPanel
|
|
{
|
|
// Load Game
|
|
[SerializeField] private GameObject loadSavePanel;
|
|
[SerializeField] private GameObject descriptionContent;
|
|
|
|
[SerializeField] private Transform saveFilesT;
|
|
[SerializeField] private GameObject savefileUIPrefab;
|
|
|
|
[SerializeField] private List<Button> allButtons = new List<Button>();
|
|
[SerializeField] private List<FileInfo> allSaveFiles = new List<FileInfo>();
|
|
public Button selectedButton;
|
|
[SerializeField] private Button loadButton;
|
|
[SerializeField] private Button deleteButton;
|
|
[SerializeField] private AudioSource MainAudioSource;
|
|
|
|
//Textes
|
|
[SerializeField] private GameObject screenshot;
|
|
[SerializeField] private TextMeshProUGUI loadCharName;
|
|
[SerializeField] private TextMeshProUGUI loadLevel;
|
|
[SerializeField] private TextMeshProUGUI loadLocation;
|
|
[SerializeField] private TextMeshProUGUI loadDate;
|
|
[SerializeField] private TextMeshProUGUI loadSaveNumber;
|
|
[SerializeField] private TextMeshProUGUI loadSaveVersion;
|
|
|
|
[SerializeField] private GameObject deleteConfirmationGameobject;
|
|
[SerializeField] private TextMeshProUGUI deleteConfirmationText;
|
|
[SerializeField] private Button deleteDefaultButton;
|
|
|
|
|
|
|
|
GameObject firstElementInList;
|
|
bool ready;
|
|
|
|
public UnityEvent OnPanelCloses;
|
|
|
|
private void OnEnable()
|
|
{
|
|
ready = false;
|
|
// Clear savegame
|
|
foreach (Transform go in saveFilesT.transform)
|
|
Destroy(go.gameObject);
|
|
|
|
allSaveFiles.Clear();
|
|
SelectedSaveFile = null;
|
|
loadSavePanel.SetActive(true);
|
|
DiscoverSaveFiles();
|
|
SpawnSaveFilesUI();
|
|
ready = true;
|
|
}
|
|
|
|
public void Update()
|
|
{
|
|
if (RckInput.isUsingGamepad)
|
|
{
|
|
if (RckInput.input.currentActionMap.FindAction("Back").triggered)
|
|
ClosePanel();
|
|
}
|
|
}
|
|
|
|
/// <summary>
|
|
/// Scans Application.persistentDataPath to get all the savegames
|
|
/// </summary>
|
|
[ContextMenu("Discover")]
|
|
private void DiscoverSaveFiles()
|
|
{
|
|
if (!Directory.Exists(System.Environment.GetFolderPath(System.Environment.SpecialFolder.MyDocuments)+@"\My Games\Firstborn\Saves"))
|
|
Directory.CreateDirectory(System.Environment.GetFolderPath(System.Environment.SpecialFolder.MyDocuments)+@"\My Games\Firstborn\Saves");
|
|
|
|
// Get all files
|
|
DirectoryInfo dir = new DirectoryInfo(System.Environment.GetFolderPath(System.Environment.SpecialFolder.MyDocuments)+@"\My Games\Firstborn\Saves");
|
|
FileInfo[] info = dir.GetFiles("*.json*");
|
|
|
|
foreach (FileInfo f in info)
|
|
allSaveFiles.Add(f);
|
|
|
|
allSaveFiles.Sort(SortByCreationDate);
|
|
}
|
|
|
|
/// <summary>
|
|
/// Spawns the save games as buttons
|
|
/// </summary>
|
|
private void SpawnSaveFilesUI()
|
|
{
|
|
for (int i = 0; i < allSaveFiles.Count; i++)
|
|
{
|
|
GameObject SaveGame = Instantiate(savefileUIPrefab, saveFilesT);
|
|
SaveGameButtonUI go = SaveGame.GetComponent<SaveGameButtonUI>();
|
|
ButtonSounds BS = SaveGame.GetComponent<ButtonSounds>();
|
|
BS.source = MainAudioSource;
|
|
go.text.text = allSaveFiles[i].Name.Substring(0, allSaveFiles[i].Name.IndexOf('.'));
|
|
go.panel = this;
|
|
|
|
go.indexInList = i;
|
|
go.LoadSaveFile(allSaveFiles[i]);
|
|
go.isLoading = true;
|
|
|
|
if (firstElementInList == null)
|
|
firstElementInList = go.gameObject;
|
|
|
|
allButtons.Add(go.GetComponent<Button>());
|
|
}
|
|
}
|
|
|
|
public override void OnSelectedSavegameChanges()
|
|
{
|
|
if (selectedSaveFile == null)
|
|
{
|
|
loadButton.interactable = false;
|
|
deleteButton.interactable = false;
|
|
descriptionContent.SetActive(false);
|
|
}
|
|
else
|
|
{
|
|
loadButton.interactable = true;
|
|
deleteButton.interactable = true;
|
|
descriptionContent.SetActive(true);
|
|
|
|
string screenPath = selectedSaveFile.fileInfo.FullName.Substring(0, selectedSaveFile.fileInfo.FullName.IndexOf('.'));
|
|
screenPath += ".png";
|
|
StartCoroutine(LoadCurrentImageX(screenPath));
|
|
// screenshot.sprite = LoadSprite(screenPath);
|
|
|
|
loadCharName.text = selectedSaveFile.saveFile.PlayerData.playerName;
|
|
loadLevel.text = selectedSaveFile.saveFile.PlayerData.playerLevel.ToString();
|
|
loadLocation.text = selectedSaveFile.saveFile.PlayerData.playerLocation;
|
|
loadDate.text = selectedSaveFile.saveFile.SaveFileData.saveDate;
|
|
loadSaveNumber.text = selectedSaveFile.saveFile.SaveFileData.saveNumber.ToString();
|
|
loadSaveVersion.text = selectedSaveFile.saveFile.SaveFileData.fileVersion.ToString();
|
|
}
|
|
}
|
|
|
|
IEnumerator LoadCurrentImageX(string imagePath) {
|
|
UnityWebRequest WWW = UnityWebRequestTexture.GetTexture(imagePath);
|
|
yield return WWW.SendWebRequest();
|
|
if (WWW.result != UnityWebRequest.Result.ConnectionError) {
|
|
screenshot.GetComponent<RawImage> ().texture = DownloadHandlerTexture.GetContent(WWW);
|
|
screenshot.GetComponent<RawImage> ().SetNativeSize();
|
|
screenshot.GetComponent<RawImage> ().SizeToParent();
|
|
}
|
|
WWW.Dispose();
|
|
}
|
|
|
|
public void ConfirmLoadSave()
|
|
{
|
|
Time.timeScale = 1;
|
|
|
|
if (selectedSaveFile != null)
|
|
SaveSystemManager.instance.LoadSaveGame(selectedSaveFile.fileInfo, selectedSaveFile.saveFile);
|
|
}
|
|
|
|
public void ConfirmDeleteSaveGame()
|
|
{
|
|
if (selectedSaveFile != null)
|
|
{
|
|
DeleteSaveGame(selectedSaveFile);
|
|
Destroy(selectedSaveFile.gameObject);
|
|
|
|
if(RckInput.isUsingGamepad && allButtons.Count > 1 && allButtons[0] != null)
|
|
allButtons[0].Select();
|
|
|
|
SelectedSaveFile = null;
|
|
}
|
|
}
|
|
|
|
public void DeleteSaveGame(SaveGameButtonUI _save)
|
|
{
|
|
string filePath = _save.fileInfo.FullName;
|
|
|
|
if (File.Exists(filePath))
|
|
File.Delete(filePath);
|
|
|
|
string imagePath = filePath.Replace(".json", ".png");
|
|
|
|
if (File.Exists(imagePath))
|
|
File.Delete(imagePath);
|
|
|
|
deleteConfirmationGameobject.SetActive(false);
|
|
}
|
|
|
|
public void ClosePanel()
|
|
{
|
|
loadSavePanel.SetActive(false);
|
|
SelectedSaveFile = null;
|
|
|
|
// Clear savegame
|
|
foreach (Transform t in saveFilesT.transform)
|
|
Destroy(t.gameObject);
|
|
|
|
OnPanelCloses.Invoke();
|
|
}
|
|
|
|
public static int SortByCreationDate(FileInfo f1, FileInfo f2)
|
|
{
|
|
var d1 = File.GetLastWriteTime(f1.FullName);
|
|
var d2 = File.GetLastWriteTime(f2.FullName);
|
|
return System.DateTime.Compare(d2, d1);
|
|
}
|
|
|
|
|
|
/// <summary>
|
|
/// Selects the first button that represents a savegame
|
|
/// </summary>
|
|
public void SelectFirstElementInList()
|
|
{
|
|
StartCoroutine(SelectFirstElementTask());
|
|
}
|
|
|
|
IEnumerator SelectFirstElementTask()
|
|
{
|
|
while (!ready)
|
|
yield return null;
|
|
|
|
if (firstElementInList != null)
|
|
EventSystem.current.SetSelectedGameObject(firstElementInList);
|
|
}
|
|
|
|
public void DeleteButton()
|
|
{
|
|
selectedButton = selectedSaveFile.GetComponent<Button>();
|
|
|
|
deleteConfirmationText.text = "Are you sure you want to delete the savegame: \n\n" + selectedSaveFile.fileInfo.Name.Replace(".json", "") + "?";
|
|
deleteConfirmationGameobject.SetActive(true);
|
|
|
|
deleteDefaultButton.Select();
|
|
StartCoroutine(ConfirmationButtonSelectionTask());
|
|
}
|
|
|
|
IEnumerator ConfirmationButtonSelectionTask()
|
|
{
|
|
yield return new WaitForSeconds(1);
|
|
deleteDefaultButton.Select();
|
|
}
|
|
|
|
public void CloseDeleteConfirmation()
|
|
{
|
|
deleteConfirmationGameobject.SetActive(false);
|
|
selectedButton.Select();
|
|
}
|
|
}
|
|
}
|
|
|
|
static class CanvasExtensions {
|
|
public static Vector2 SizeToParent(this RawImage image, float padding = 0) {
|
|
float w = 0, h = 0;
|
|
var parent = image.transform.parent.GetComponent<RectTransform>();
|
|
var imageTransform = image.GetComponent<RectTransform>();
|
|
|
|
// check if there is something to do
|
|
if (image.texture != null) {
|
|
if (!parent) { return imageTransform.sizeDelta; } //if we don't have a parent, just return our current width;
|
|
padding = 1 - padding;
|
|
float ratio = image.texture.width / (float)image.texture.height;
|
|
var bounds = new Rect(0, 0, parent.rect.width, parent.rect.height);
|
|
if (Mathf.RoundToInt(imageTransform.eulerAngles.z) % 180 == 90) {
|
|
//Invert the bounds if the image is rotated
|
|
bounds.size = new Vector2(bounds.height, bounds.width);
|
|
}
|
|
//Size by height first
|
|
h = bounds.height * padding;
|
|
w = h * ratio;
|
|
if (w < bounds.width * padding) { //If it doesn't fit, fallback to width;
|
|
w = bounds.width * padding;
|
|
h = w / ratio;
|
|
}
|
|
}
|
|
imageTransform.SetSizeWithCurrentAnchors(RectTransform.Axis.Horizontal, w);
|
|
imageTransform.SetSizeWithCurrentAnchors(RectTransform.Axis.Vertical, h);
|
|
return imageTransform.sizeDelta;
|
|
}
|
|
} |