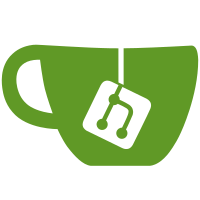
Upgraded the framework which includes the Distant cell rendering. I have been merging meshes to cut down the Draw calls, so far gaining on average 20FPS everywhere. New bugs are the magic fails (Again) and the environment presets fail to call when outside. Currently trying to build new lightmaps for the combined meshes.
109 lines
3.7 KiB
C#
109 lines
3.7 KiB
C#
using System.Collections;
|
|
using System.Collections.Generic;
|
|
using UnityEngine;
|
|
using RPGCreationKit;
|
|
|
|
|
|
namespace RPGCreationKit
|
|
{
|
|
/// <summary>
|
|
/// Class that contains general game settings. Tweak it as you like.
|
|
/// </summary>
|
|
public static class RCKSettings
|
|
{
|
|
public static float NPC_TO_NPC_CONVERSATION_DISTANCE = 3.5f;
|
|
public static float NPC_LOOKAT_TARGET_DISTANCE = 5f;
|
|
public static float NPC_STOP_FOLLOW_AFTER_DISTANCE = 2.2f;
|
|
public static float PLAYER_HEARS_NPC_DIALOGUES_DISTANCE = 15f;
|
|
public static float PLAYER_DISTANCE_TO_DIALOGUE = 3f;
|
|
public static float PLAYER_DISTANCE_TO_DISPLAY_ENEMY_BAR = 100f;
|
|
|
|
public static float CHARGED_ATTACK_MOUSE_DOWN_TIME = 0.5f;
|
|
|
|
public static float MIN_VELOCITY_TO_CONTINUE_ROTATING_ARROW = 5f;
|
|
|
|
public static float DISTANCE_SHOW_NPC_IN_COMBAT_UI = 100f;
|
|
|
|
public static float MELEE_GENERAL_RIGIDBODY_FORCE = 20f;
|
|
public static float ARROW_GENERAL_RIGIDBODY_FORCE = 5f;
|
|
|
|
public static float MELEE_ONBODY_RIGIDBODY_FORCE = 35f;
|
|
public static float ARROW_ONBODY_RIGIDBODY_FORCE = 10f;
|
|
|
|
public static float DRAIN_HEALTH_DEFAULT_RATE = 200f;
|
|
public static float DRAIN_STAMINA_DEFAULT_RATE = 200f;
|
|
|
|
public static float DISTANCE_WHEN_NPCS_START_LOOK_AT = 3.5f;
|
|
|
|
public static float CROSSHAIR_HIT_TIME = .25f;
|
|
|
|
public static float STOPPING_DETECTION_THRESHOLD = 0.15f;
|
|
|
|
public static float HOSTILE_FACTION_COMBAT_THERSHOLD = 10;
|
|
|
|
// EDITOR
|
|
public static string EDITOR_AI_LOAD_LOCATION = "Assets/RPG Creation Kit/Prefab Library/AI/";
|
|
public static string EDITOR_AI_SAVE_LOCATION = "Assets/RPG Creation Kit/Prefab Library/AI/";
|
|
|
|
public static string EDITOR_CREATUREAI_LOAD_LOCATION = "Assets/RPG Creation Kit/Prefab Library/AI/Creatures/";
|
|
public static string EDITOR_CREATUREAI_SAVE_LOCATION = "Assets/RPG Creation Kit/Prefab Library/AI/Creatures/";
|
|
|
|
// Code
|
|
public static float DEFAULT_WEAPON_REACH = 4.0f;
|
|
|
|
public static float INTERACTOR_RAYCAST_MAXDISTANCE = float.MaxValue;
|
|
|
|
public static float DRAIN_STAMINA_ON_ATTACK_SPEEDAMOUNT = 25f;
|
|
public static float DRAIN_STAMINA_ON_ATTACKBLOCKED_SPEEDAMOUNT = 25f;
|
|
public static float DRAIN_MANA_ON_CAST_SPEEDAMOUNT = 25f;
|
|
|
|
public static string GAME_VERSION = "1.4";
|
|
public static string SAVE_TYPE_VERSION = "rck_default";
|
|
|
|
// Save Version should be x.y not x.y.z (1.1, 1.12, 1.123 NOT 1.1.2), when version checking, the "." is removed and the version is the two numbers combined (1.1 = 11, 1.2 = 12, 1.12 = 112)
|
|
public static string FILE_SAVE_VERSION = "1.4";
|
|
|
|
public static float PROJECTILE_DESPAWN_TIME = 30f;
|
|
|
|
public static float DISTANCE_BEFORE_ROTATING_TO_TARGET = 4.5f;
|
|
|
|
|
|
// NEW GAME START
|
|
public static string RCK_NEW_STARTING_LOCATION = "Starting cave";
|
|
public static string RCK_NEW_STARTING_WORLDSPACEID = "00CanyonInteriors";
|
|
public static string RCK_NEW_STARTING_CELLID = "CanyonCaveCell1-1Cave2";
|
|
public static int RCK_NEW_STARTING_LEVEL = 1;
|
|
public static Vector3 RCK_NEW_STARTING_POS = new Vector3(93.52182f, -6.028f, -76.271f);
|
|
public static Vector3 RCK_NEW_STARTING_ROT = new Vector3(0, 0, 0);
|
|
|
|
public static float ATTRIBUTES_DEF_HEALTH = 200.0f;
|
|
public static float ATTRIBUTES_DEF_STAMINA = 200.0f;
|
|
public static float ATTRIBUTES_DEF_MANA = 200.0f;
|
|
|
|
// Save
|
|
public static bool JSON_PRETTY_PRINT = false;
|
|
public static float PLAYER_DAMAGE_SPEED = 100f;
|
|
|
|
|
|
|
|
// SETTINGS MENU - SET BY PLAYER AT RUNTIME
|
|
public static float MOUSE_HORIZONTAL_SPEED;
|
|
public static float MOUSE_VERTICAL_SPEED;
|
|
|
|
public static int GetSaveVersion(string ver)
|
|
{
|
|
// Remove the "." from the version
|
|
string str = ver.Replace(".","");
|
|
|
|
int fileVersion = 0;
|
|
if (int.TryParse(str, out fileVersion))
|
|
{
|
|
//Debug.Log("Loading file version: " + fileVersion);
|
|
return fileVersion;
|
|
}
|
|
else
|
|
return 1;
|
|
}
|
|
|
|
}
|
|
} |