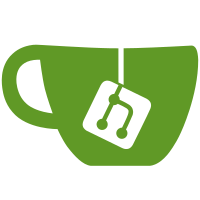
made most of the game Moddable. There is an in editor tool to make the mods and set them all up, and im making an external tool that will show your mods, lets you edit any information and it will pack it up and upload it. currently working on the uploading. Next I will make the game able to download and install. - Fuck Nexus.
201 lines
7.5 KiB
C#
201 lines
7.5 KiB
C#
using System;
|
|
using System.Collections;
|
|
using System.Collections.Generic;
|
|
using UnityEngine;
|
|
using TMPro;
|
|
using UnityEngine.Networking;
|
|
using UnityEngine.UI;
|
|
|
|
public class OnlineAccountHandler : MonoBehaviour
|
|
{
|
|
[SerializeField] private TMP_InputField Username;
|
|
[SerializeField] private TMP_InputField Password;
|
|
[SerializeField] private GameObject LoginBox;
|
|
[SerializeField] private GameObject UserInfoBox;
|
|
[SerializeField] private GameObject ModsButton;
|
|
[SerializeField] private GameObject AutoLogin;
|
|
[SerializeField] private TextMeshProUGUI[] UserInfo;
|
|
[SerializeField] private string AccountName;
|
|
[SerializeField] private INIReader INI;
|
|
[SerializeField] public string BearerID;
|
|
[SerializeField] private RawImage ProfilePic;
|
|
[SerializeField] private RawImage ProfileBackground;
|
|
[SerializeField] public bool Loggedin = false;
|
|
[SerializeField] private GameObject ModContainer;
|
|
|
|
// Start is called before the first frame update
|
|
void Start() {
|
|
StartCoroutine(DelayStart());
|
|
// StartCoroutine(CheckForAccount());
|
|
|
|
}
|
|
|
|
// Update is called once per frame
|
|
void Update()
|
|
{
|
|
|
|
}
|
|
|
|
IEnumerator DelayStart() {
|
|
yield return new WaitForSeconds(2.0f);
|
|
Username.text = INI.ReadSettingString("Account Info", "Username", "None");
|
|
Password.text = INI.ReadSettingString("Account Info", "password", "None");
|
|
if (Username.text != "None") {
|
|
AutoLogin.SetActive(true);
|
|
}
|
|
}
|
|
|
|
public void SubmitInfo() {
|
|
StartCoroutine(Login());
|
|
}
|
|
|
|
public void LogMeIn() {
|
|
StartCoroutine(CheckForAccount());
|
|
}
|
|
|
|
public void UserButtonPushed() {
|
|
if (Loggedin) {
|
|
if (UserInfoBox.activeSelf == true) {
|
|
UserInfoBox.SetActive(false);
|
|
} else {
|
|
UserInfoBox.SetActive(true);
|
|
}
|
|
} else {
|
|
if (LoginBox.activeSelf == true) {
|
|
LoginBox.SetActive(false);
|
|
} else {
|
|
LoginBox.SetActive(true);
|
|
}
|
|
}
|
|
}
|
|
|
|
private IEnumerator CheckForAccount() {
|
|
yield return new WaitForSeconds(2.0f);
|
|
string Token = INI.ReadSettingString("Account Info", "refresh_token", "None");
|
|
if (Token == "None") {
|
|
AutoLogin.SetActive(false);
|
|
yield break;
|
|
}
|
|
Dictionary<string, string> content = new Dictionary<string, string>();
|
|
//Fill key and value
|
|
content.Add("grant_type", "refresh_token");
|
|
content.Add("redirect_uri", "https://schaken-mods.com/tools/modhuntergooglelogin/");
|
|
content.Add("client_id", "15012dd3e9d0af7ce386b3dffe53292b");
|
|
content.Add("refresh_token", Token);
|
|
content.Add("scope", "test");
|
|
|
|
UnityWebRequest www = UnityWebRequest.Post("https://schaken-mods.com/oauth/token/", content);
|
|
yield return www.SendWebRequest();
|
|
if (www.result != UnityWebRequest.Result.ConnectionError) {
|
|
string resultContent = www.downloadHandler.text;
|
|
// Debug.Log("Response: "+resultContent);
|
|
if (resultContent.Contains("\"access_token\":\"")) {
|
|
string NewToken = resultContent.Split (new string[] { "\"access_token\":\"" }, StringSplitOptions.None)[1].Split ('\"')[0].Trim ();
|
|
string RefreshToken = resultContent.Split (new string[] { "\"refresh_token\":\"" }, StringSplitOptions.None)[1].Split ('\"')[0].Trim ();
|
|
INI.SaveSettingString("Account Info", "access_token", NewToken);
|
|
INI.SaveSettingString("Account Info", "refresh_token", RefreshToken);
|
|
BearerID = NewToken;
|
|
StartCoroutine(GetUserInfo());
|
|
} else {
|
|
Debug.Log("Response: "+resultContent);
|
|
}
|
|
} else {
|
|
Debug.Log("Token Failed: "+www.error);
|
|
}
|
|
www.Dispose();
|
|
AutoLogin.SetActive(false);
|
|
}
|
|
|
|
private IEnumerator Login() {
|
|
if (Username.text == "") {
|
|
Debug.Log("Username empty");
|
|
yield break;
|
|
}
|
|
if (Password.text == "") {
|
|
Debug.Log("password empty");
|
|
yield break;
|
|
}
|
|
Dictionary<string, string> content = new Dictionary<string, string>();
|
|
//Fill key and value
|
|
content.Add("grant_type", "password");
|
|
content.Add("redirect_uri", "https://schaken-mods.com/tools/modhuntergooglelogin/");
|
|
content.Add("client_id", "15012dd3e9d0af7ce386b3dffe53292b");
|
|
content.Add("username", Username.text);
|
|
content.Add("password", Password.text);
|
|
content.Add("scope", "test");
|
|
|
|
UnityWebRequest www = UnityWebRequest.Post("https://schaken-mods.com/oauth/token/", content);
|
|
yield return www.SendWebRequest();
|
|
if (www.result != UnityWebRequest.Result.ConnectionError) {
|
|
string resultContent = www.downloadHandler.text;
|
|
// Debug.Log("Response: "+resultContent);
|
|
if (resultContent.Contains("\"access_token\":\"")) {
|
|
string NewToken = resultContent.Split (new string[] { "\"access_token\":\"" }, StringSplitOptions.None)[1].Split ('\"')[0].Trim ();
|
|
string RefreshToken = resultContent.Split (new string[] { "\"refresh_token\":\"" }, StringSplitOptions.None)[1].Split ('\"')[0].Trim ();
|
|
INI.SaveSettingString("Account Info", "access_token", NewToken);
|
|
INI.SaveSettingString("Account Info", "refresh_token", RefreshToken);
|
|
BearerID = NewToken;
|
|
StartCoroutine(GetUserInfo());
|
|
} else {
|
|
Debug.Log("Response: "+resultContent);
|
|
}
|
|
} else {
|
|
Debug.Log("Token Failed: "+www.error);
|
|
}
|
|
www.Dispose();
|
|
}
|
|
|
|
private IEnumerator GetUserInfo() {
|
|
var url = "https://schaken-mods.com/api/core/me";
|
|
|
|
UnityWebRequest uwr = UnityWebRequest.Get(url);
|
|
uwr.SetRequestHeader("Authorization", "Bearer " + BearerID);
|
|
yield return uwr.SendWebRequest();
|
|
if (uwr.result == UnityWebRequest.Result.ConnectionError) {
|
|
Debug.Log("Error While Sending for User info: " + uwr.error);
|
|
} else {
|
|
string result = uwr.downloadHandler.text;
|
|
string FullTXT = result.Replace(@"\", string.Empty);
|
|
|
|
// Debug.Log("FullTXT: " + FullTXT);
|
|
if (FullTXT.Contains("photoUrl") == true) {
|
|
Loggedin = true;
|
|
ModsButton.SetActive(true);
|
|
string TempProPic = FullTXT.Split (new string[] { "\"photoUrl\": \"" }, StringSplitOptions.None)[1].Split ('\"')[0].Trim ();
|
|
string TempBackPic = FullTXT.Split (new string[] { "\"coverPhotoUrl\": \"" }, StringSplitOptions.None)[1].Split ('\"')[0].Trim ();
|
|
string TempBirthday = FullTXT.Split (new string[] { "\"birthday\": \"" }, StringSplitOptions.None)[1].Split ('\"')[0].Trim ();
|
|
string TempRank = FullTXT.Split (new string[] { "\"rank\"" }, StringSplitOptions.None)[1].Split (new string[] { "\"name\": \"" }, StringSplitOptions.None)[1].Split ('\"')[0].Trim ();
|
|
AccountName = FullTXT.Split (new string[] { "\"name\": \"" }, StringSplitOptions.None)[1].Split ('\"')[0].Trim ();
|
|
string TempJoined = FullTXT.Split (new string[] { "\"joined\": \"" }, StringSplitOptions.None)[1].Split ('\"')[0].Trim ();
|
|
string TempPoints = FullTXT.Split (new string[] { "\"achievements_points\": " }, StringSplitOptions.None)[1].Split (',')[0].Trim ();
|
|
string TempPosts = FullTXT.Split (new string[] { "\"posts\": " }, StringSplitOptions.None)[1].Split (',')[0].Trim ();
|
|
string TempViews = FullTXT.Split (new string[] { "\"profileViews\": " }, StringSplitOptions.None)[1].Split (',')[0].Trim ();
|
|
StartCoroutine(LoadCurrentImage(TempProPic, ProfilePic));
|
|
StartCoroutine(LoadCurrentImage(TempBackPic, ProfileBackground));
|
|
UserInfo[0].text = AccountName;
|
|
UserInfo[1].text = TempBirthday;
|
|
UserInfo[2].text = TempRank;
|
|
UserInfo[3].text = TempJoined;
|
|
UserInfo[4].text = TempPoints;
|
|
UserInfo[5].text = TempPosts;
|
|
UserInfo[6].text = TempViews;
|
|
UserInfo[7].text = AccountName;
|
|
|
|
// load mods holder
|
|
DontDestroyOnLoad(ModContainer);
|
|
}
|
|
}
|
|
}
|
|
|
|
IEnumerator LoadCurrentImage(string imagePath, RawImage TheImage) {
|
|
UnityWebRequest WWW = UnityWebRequestTexture.GetTexture(imagePath);
|
|
yield return WWW.SendWebRequest();
|
|
if (WWW.result != UnityWebRequest.Result.ConnectionError) {
|
|
TheImage.texture = DownloadHandlerTexture.GetContent(WWW);
|
|
TheImage.SetNativeSize();
|
|
TheImage.SizeToParent();
|
|
}
|
|
WWW.Dispose();
|
|
}
|
|
}
|